Adding Dynamic Behavior to our Angular Application
In the previous article I showed you how to make our application dynamic with the help of variable interpolation. But that did not do anything more than putting the values directly inside the template. So, what happens when we want to change the profession without reloading the page. For example, think about facebook (or other social medias) where you post something and when someone clicks the like button the number of likes get updated on your screen. The data is retrieved in the background (let's not think about whether Ajax or Websocket is being used for the purpose) and the view is updated without reloading the page. Now, let's think that someone is viewing your profile and at that time your edited your profile and made some change to your current profession. This data should be reflected on browser window of whoever is viewing your profile.
Currently we are not going to connect to some kind of server to get some data in real time. We will work with networking stuff later in this series of learning Angular. If we were connecting to some kind of server to get data overtime we would do that asynchronously. The same behavior can be emulated with the help of the setTimeout() function. We will run some code after some time to change the variable value of profession. And in this way you will see the profession get updated without reloading our page. In future article we can implement the same thing with some kind of networking involved.
Let's see what we already have in place.
app.component.html
<b> Hello World </b> <br/>
My name is Md. {{ name }} <br/>
I am a {{ profession }}. <br/>
Do not forget to visit me at: {{ website }}
app.component.ts
import { Component } from '@angular/core';
@Component({
selector: 'app-root',
templateUrl: './app.component.html',
styleUrls: ['./app.component.css']
})
export class AppComponent {
name = "Md. Sabuj Sarker";
profession = "Software Engineer";
website = "http://sabuj.me";
}
Now we want to change the value of profession from "Software Engineer" to "Writer." But we want to do that after some time so that we can see the change. We want to wait for 1 second, which is 1000 milliseconds. Our code should look like this:
setTimeout(()=>{
this.profession = "Writer";
}, 1000);
In the code above this refers to the current component object. But where do we run this code? Every class can has a constructor. A constructor is a method (a method is a function inside a class) that is executed every time a class is instantiated. The constructor method in TypeScript is identified as constructor. Let's put our code inside of the class.
class AppComponent {
name = "Md. Sabuj Sarker";
profession = "Software Engineer";
website = "http://sabuj.me";
constructor(){
setTimeout(()=>{
this.profession = "Writer";
}, 1000);
}
}
Go the browser to see the changes after one second. If it happens quickly increase the wait time.
Did you notice one thing that we are using an arrow function with setTimeout instead of a an ordinary function? If have no idea about arrow functions then you can learn about it or replace that with a pure JavaScript anonymous function.
So, we have made our application interactive enough by now. Now we can advance by creating a clock below our profile. Let's modify our class by adding a time field to it.
class AppComponent {
name = "Md. Sabuj Sarker";
profession = "Software Engineer";
website = "http://sabuj.me";
time = new Date();
constructor(){
setTimeout(()=>{
this.profession = "Writer";
}, 1000);
}
}
We also need to add proper HTML to show the date. Let's just fall back to interpolation.
<b> Hello World </b> <br/>
My name is Md. {{ name }} <br/>
I am a {{ profession }}. <br/>
Do not forget to visit me at: {{ website }} <br/>
Current time: {{ time }}
This time we will use setInterval to update the clock once every second.
class AppComponent {
name = "Md. Sabuj Sarker";
profession = "Software Engineer";
website = "http://sabuj.me";
time = new Date();
constructor(){
setTimeout(()=>{
this.profession = "Writer";
}, 1000);
setInterval(() => {
this.time = new Date();
}, 1000);
}
}
Go to the browser and you will see a ticking clock under your profile with the current date and time on it.
To bring it further I would like to make a counter. Every time you click a button a number will increase by one and display the increased value on another part of the screen. In a later article I will explore about Angular events, but for now we will just need to understand a little bit of Angular syntax for handling button clicks. Let's say we have an HTML button like below.
<button> Update++ </button>
Now if we want to say that we want to call some function from the component class when the button is clicked we write it like below:
<button (click)="handleClick()"> Update++ </button>
Notice that little cute syntax: (click) - it instructs Angular to run the code on the right side of the equal sign and in this case it is a method from the component class. Let's make the click handler function, create a component field (variable) for counter and initialize that to 0.
class AppComponent {
name = "Md. Sabuj Sarker";
profession = "Software Engineer";
website = "http://sabuj.me";
time = new Date();
counter = 0;
constructor(){
setTimeout(()=>{
this.profession = "Writer";
}, 1000);
setInterval(() => {
this.time = new Date();
}, 1000);
}
handleClick(){
this.counter++;
}
}
Now, a little bit of change to our HTML template.
<b> Hello World </b> <br/>
My name is Md. {{ name }} <br/>
I am a {{ profession }}. <br/>
Do not forget to visit me at: {{ website }} <br/>
Current time: {{ time }} <br/>
Counter: {{ counter }} <br/>
<button (click)="handleClick()"> Update++ </button>
Go to the browser, see the changes happening, click the Update++ button to see the counter value changing.
Conclusion
We have just started to make our application dynamic. This is just the beginning of the magic of Angular—we have a lot more left to do.
Previous article: Creating Your First Simple Angular Application |
Next article: Displaying an Array of Elements in an Angular Template |
About the Author
My name is Md. Sabuj Sarker. I am a Software Engineer, Trainer and Writer. I have over 10 years of experience in software development, web design and development, training, writing and some other cool stuff including few years of experience in mobile application development. I am also an open source contributor. Visit my github repository with username SabujXi.
Recent Stories
Top DiscoverSDK Experts
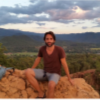
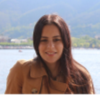
Compare Products
Select up to three two products to compare by clicking on the compare icon () of each product.
{{compareToolModel.Error}}
{{CommentsModel.TotalCount}} Comments
Your Comment