Creating Your First Simple Angular Application
In the previous article I covered how to create a new project with Angular CLI and start a very simple Angular web application. We did not write a single line of code and did not modify anything. In this article we will start modifying our very minimal Angular application and make an application of our own.
The index.html File
In Angular applications we rarely talk about the index.html file. We create it and we forget about it. Let's see what content we have in our existing index.html file.
<!doctype html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>AwesomeApp</title>
<base href="/">
<meta name="viewport" content="width=device-width, initial-scale=1">
<link rel="icon" type="image/x-icon" href="favicon.ico">
</head>
<body>
<app-root></app-root>
</body>
</html>
There is no link to JavaScript or CSS files present in the file. Even, no link to any Angular JavaScript/Typescript files exists. We do not need to include them. Our Angular CLI has set up everything for us so that after building the application, Webpack automatically includes everything for us inside the index.html file. As a result at this beginning stage you do not need to worry about how that is being done. We will explore internals in some future articles when you have moved to a more advanced stage in learning Angular.
There is also a style.css in the root src directory of your Angular project. This is the root stylesheet for your angular application. We will also use component specific CSS files in our applications.
The most important part of index.html is <app-root></app-root> inside body. app-root is the placeholder for our application. This tag is specified at some place in our Angular application that we will have a look in another section of this article. For now, keep in mind that this is the entry point of showing our application views in the index.html file.
The app.component.html
When we started our application with ng serve we saw an image, links and texts. Those things are not present inside the index.html file. So, where are those things coming from? Open app.component.html inside the src/app directory.
<!--The content below is only a placeholder and can be replaced.-->
<div style="text-align:center">
<h1>
Welcome to {{ title }}!
</h1>
<img width="300" alt="Angular Logo" src="data:image/svg+xml;base64,PHN2ZyB4bWxucz0iaHR0cDovL3d3dy53My5vcmcvMjAwMC9zdmciIHZpZXdCb3g9IjAgMCAyNTAgMjUwIj4KICAgIDxwYXRoIGZpbGw9IiNERDAwMzEiIGQ9Ik0xMjUgMzBMMzEuOSA2My4ybDE0LjIgMTIzLjFMMTI1IDIzMGw3OC45LTQzLjcgMTQuMi0xMjMuMXoiIC8+CiAgICA8cGF0aCBmaWxsPSIjQzMwMDJGIiBkPSJNMTI1IDMwdjIyLjItLjFWMjMwbDc4LjktNDMuNyAxNC4yLTEyMy4xTDEyNSAzMHoiIC8+CiAgICA8cGF0aCAgZmlsbD0iI0ZGRkZGRiIgZD0iTTEyNSA1Mi4xTDY2LjggMTgyLjZoMjEuN2wxMS43LTI5LjJoNDkuNGwxMS43IDI5LjJIMTgzTDEyNSA1Mi4xem0xNyA4My4zaC0zNGwxNy00MC45IDE3IDQwLjl6IiAvPgogIDwvc3ZnPg==">
</div>
<h2>Here are some links to help you start: </h2>
<ul>
<li>
<h2><a target="_blank" rel="noopener" href="https://angular.io/tutorial">Tour of Heroes</a></h2>
</li>
<li>
<h2><a target="_blank" rel="noopener" href="https://github.com/angular/angular-cli/wiki">CLI Documentation</a></h2>
</li>
<li>
<h2><a target="_blank" rel="noopener" href="https://blog.angular.io/">Angular blog</a></h2>
</li>
</ul>
So, this file is responsible for what we were seeing on our browser window. We want to put our own content there. So, delete everything inside that file, and then write the following lines of HTML inside of it.
<b> Hello World </b> <br/>
My name is Md. Sabuj Sarker <br/>
I am a Software Engineer. <br/>
Do not forget to visit me at: http://sabuj.me
Place your name, profession and website address in place of mine to see your info on the browser window.
Run the command ng serve --open to see the changes.
But that was just the static HTML part! Where are the dynamic behaviors? Where is JavaScript or TypeScript?
The app.component.ts
As I told you before that views in Angular are governed by components. The app.component.html is just the HTML code for the component. The real component, inside the TypeScript code, is inside the app.component.ts inside src/app directory. A component is a class decorated with the @Component decorator. Components are the place we write code for the views. So, let's open the TypeScript file and see what's inside:
import { Component } from '@angular/core';
@Component({
selector: 'app-root',
templateUrl: './app.component.html',
styleUrls: ['./app.component.css']
})
export class AppComponent {
title = 'app';
}
The class named AppComponent there is one of the component I was talking about. And it is decorated with the decorator called Component. For now understand that a decorator is preceded with an @ symbol. Do you remember app-root from the index.html file? In the Component decorator an object is passed. This object contains a key named selector that indicates where this component inside the parent will be rendered. So, this component is going to be rendered inside the index.html file.
The little amount of HTML we have written for app.component.html is static, we want it to take some data from the component class. In the component class we are going to declare that data as public variables. There is a preexisting variable in the class body called title—just remove that and put your name, profession, and website address there.
export class AppComponent {
name = "Md. Sabuj Sarker";
profession = "Software Engineer";
website = "http://sabuj.me";
}
Now, how do we tell the template app.component.html to accept data from the component? This action can take place with the help of interpolation. It is a sweet easy syntax inside HTML. Put the variable names inside double opening and closing curly braces. For example, {{ variable_name }}. The spaces around the variable name is optional. Let's see the result by going to the browser again.
So, it is time to modify our HTML code of the component accordingly as below.
<b> Hello World </b> <br/>
My name is {{ name }} <br/>
I am a {{ profession }}. <br/>
Do not forget to visit me at: {{ website }}
If you did not stop your development server started with ng serve you do not need to do anything again to recompile and run your application again. Go to the browser and see the changes.
Conclusion
In this lesson we have made our application dynamic, but not as dynamic as we usually want. Putting variables and values in the class versus putting values directly inside the HTML template is still not different. But, in reality we have made huge improvement. Every journey starts with a single first step. You will see how that is in the next article. Till then, keep practicing.
Next article: Adding Dynamic Behavior to our Angular Application |
About the Author
My name is Md. Sabuj Sarker. I am a Software Engineer, Trainer and Writer. I have over 10 years of experience in software development, web design and development, training, writing and some other cool stuff including few years of experience in mobile application development. I am also an open source contributor. Visit my github repository with username SabujXi.
Recent Stories
Top DiscoverSDK Experts
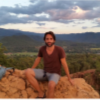
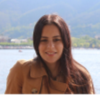
Compare Products
Select up to three two products to compare by clicking on the compare icon () of each product.
{{compareToolModel.Error}}
{{CommentsModel.TotalCount}} Comments
Your Comment