Functions in Kotlin
Being a statically typed language, functions have an important role to play in Kotlin. You’re all familiar with functions of course, and functions in Kotlin are not so different from those in other languages. In Kotlin, we declare functions using the keyword “fun.” As with other object-oriented languages, you’ll also need a return type and an optional argument list.
Let’s not wait any time here and jump right into an example. Here, we’ll define a function called FirstFunction which we’ll call from main before passing an argument. Pretty basic stuff, so let’s go for it:
fun main(args: Array<String>) {
println(FirstFunction("DiscoverSDK.com"))
}
fun FirstFunction(x: String): String {
var c:String = "Hello and welcome to: "
return (c+x)
}
When we run this code, we receive the following output:
Hello and welcome to: DiscoverSDK.com
Notice the basic syntax used in the function:
fun <functionName>(<argument>:<argumentType>):<ReturnType>
OK, now let’s have a look at some different types of functions we can use in Kotlin
The Lambda Function in Kotlin
Lambda functions in Kotlin are high-level functions that are really handy in reducing the amount of boilerplate code we need to use by defining a function and declaring it. In Kotlin, we are free to declare our own lambda functions. Just declare your lambda and then pass it to the lambda function.
Here’s an example:
fun main(args: Array<String>) {
val firstlambda :(String)->Unit = {s:String->print(s)}
val v:String = "DiscoverSDK.com"
firstlambda(v)
}
In this little piece of code, we created our own lambda called “firstlambda” and then passed one variable to it. This variable is of the type String and has the value of “DiscoverSDK.com”.
No surprise, here is what the code returns:
DiscoverSDK.com
Inline Functions in Kotlin
In the last example, we saw a basic lambda function in Kotlin. Now, we can pass that lambda to a different function to get our output. This function that makes the call is an inline function.
Let’s have a look at an example:
fun main(args: Array<String>) {
val mylambda:(String)->Unit = {s:String->print(s)}
val v:String = "DiscoverSDK.com"
myFun(v,mylambda) //passing the lambda as a parameter of another function
}
fun myFun(a :String, action: (String)->Unit) { //passing the lambda
print("Hello ")
action(a)// call to lambda function
}
With our inline function, we passed our lambda as a parameter—pretty useful! Any function can be made to be inline—simply use the keywords “inline.”
Here is the output from our example”
Hello DiscoverSDK.com
That’s all for this time. In the next article, we’ll take a look at exception handling and more.
Previous Article: Delegation in Kotlin | Next Article: coming soon |
Recent Stories
Top DiscoverSDK Experts
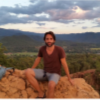
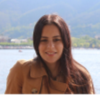
Compare Products
Select up to three two products to compare by clicking on the compare icon () of each product.
{{compareToolModel.Error}}
{{CommentsModel.TotalCount}} Comments
Your Comment