Displaying an Array of Elements in an Angular Template
In the previous article we were able to make our application dynamic and interactive. Our application started to react to changes, displayed an active clock with both date and time, reacted to button clicks. All the data that our Angular HTML template was receiving came from individual variables or class properties. But what if we want to display a lot of data of a similar type?
In our profile we currently have only one link that is a link to our website. Let's say that we want to show more links, links to our facebook, twitter, linkedin, etc. It's not wise to create a class property or variable for each of them. Tomorrow you may want to add more links and then you have to modify your code again, rebuild the application and redeploy it. That's a lot of pain to handle each time you change your mind or have a change in requirements. Again, you may want to retrieve the links from some server instead of statically putting them there.
You guessed it right! We need an array for this thing. We need to loop over the array of links and put them in the template. How do we do this? We can put the array in our component class as a variable called social_links. We can loop over the array in a method inside the class, but how do we show them in the template? Isn't it convenient if the template had some kind of mechanism to loop over them and put them in place with the help of interpolation? Yes, Angular provides such a mechanism that allows you to loop over an array declared in the class and put the elements in the template.
We have a concept of directives in Angular that we will explore in details in some later articles. For now let's work with the one that will solve our current problem. ngFor is a directive that will help us iterate over an array. A directive is put inside a start tag like an attribute. We precede the directive ngFor with an asterisk like this *ngFor. The tag in which we put this directive is replicated on every iteration. On the right hand side of the directive we put the name of the array that we want to iterate over and the variable that we want to put each individual elements of the array on each iteration. We can do other things there, but at this moment this is enough for us. The format of the code on the right hand side of ngFor is let an_element of the_array. If you have basic knowledge about ES6 or TypeScript you already know that let is used to declare a variable and of is used to instruct to take every element from an iterable. Let's start our coding.
Currently our app.component.ts looks like this:
import { Component } from '@angular/core';
@Component({
selector: 'app-root',
templateUrl: './app.component.html',
styleUrls: ['./app.component.css']
})
export class AppComponent {
name = "Md. Sabuj Sarker";
profession = "Software Engineer";
website = "http://sabuj.me";
time = new Date();
counter = 0;
constructor(){
setTimeout(()=>{
this.profession = "Writer";
}, 1000);
setInterval(() => {
this.time = new Date();
}, 1000);
}
handleClick(){
this.counter++;
}
}
We want to remove the counter, the button and the date-time. And thus we no longer need the associated code inside the constructor. We also do not want to change the position after one second. So, our constructor will be empty for now.
class AppComponent {
name = "Md. Sabuj Sarker";
profession = "Software Engineer";
website = "http://sabuj.me";
constructor(){
}
}
Now, declare a new variable called social_links that will contain an array of links you want to put inside the template.
class AppComponent {
name = "Md. Sabuj Sarker";
profession = "Software Engineer";
website = "http://sabuj.me";
social_links = [
"https://facebook.com/SabujXiP",
"https://twitter.com/SabujXi",
"https://github.com/SabujXi"
]
constructor(){
}
}
Let's modify the HTML template accordingly.
<h1>My profile</h1>
<b> Hello World </b> <br/>
My name is Md. {{ name }} <br/>
I am a {{ profession }}. <br/>
Do not forget to visit me at: {{ website }} <br/>
My Social Links: <br/>
<ol>
<li *ngFor="let link of social_links"> {{ link }} </li>
</ol>
So, the li element in the above template will be replicated on each iteration. The link variable created for the looping will be used to interpolate the value inside the list element.
Fire up your development server to see if things are working properly. Ah, it works perfectly.
One question that may pop up in your mind? What if the array is manipulated? Will Angular react to changes if we manipulate the array as it worked for changing the variable value? Changing the value of a variable and manipulating an object associated with that variable is not the same thing—your doubt is perfectly valid. Let's try it with an example. Let's try to add an extra element to the array after two seconds with that setTimeout trick.
Notice that I am using the word variable instead of field or property so that it makes you comfortable as a beginner and avoid future confusion with other similar Angular stuff that will get in our way.
class AppComponent {
name = "Md. Sabuj Sarker";
profession = "Software Engineer";
website = "http://sabuj.me";
social_links = [
"https://facebook.com/SabujXiP",
"https://twitter.com/SabujXi",
"https://github.com/SabujXi"
]
constructor(){
setTimeout(() => {
this.social_links.push("https://youtube.com/c/MdSabujSarker");
}, 2000);
}
}
Go to your browser and see that after two seconds another list element appears. Isn't that fun?
Let's try removing one element from the array after 4 seconds. I am going to remove the first element.
class AppComponent {
name = "Md. Sabuj Sarker";
profession = "Software Engineer";
website = "http://sabuj.me";
social_links = [
"https://facebook.com/SabujXiP",
"https://twitter.com/SabujXi",
"https://github.com/SabujXi"
]
constructor(){
setTimeout(() => {
this.social_links.push("https://youtube.com/c/MdSabujSarker");
}, 2000);
setTimeout(() => {
this.social_links.shift();
}, 4000);
}
}
Go to your browser to see our expected behavior of our application.
Conclusion
Angular has made frontend programming much easier. The developers behind this framework realized that looping over elements and creating HTML with that should be done in template rather than in the JavaScript/TypeScript code. I’ll have more articles for you soon.
Previous article: Adding Dynamic Behavior to our Angular Application |
Next article: Working With Objects, ngFor and ngIf in Angular |
About the Author
My name is Md. Sabuj Sarker. I am a Software Engineer, Trainer and Writer. I have over 10 years of experience in software development, web design and development, training, writing and some other cool stuff including few years of experience in mobile application development. I am also an open source contributor. Visit my github repository with username SabujXi.
Recent Stories
Top DiscoverSDK Experts
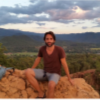
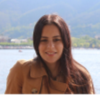
Compare Products
Select up to three two products to compare by clicking on the compare icon () of each product.
{{compareToolModel.Error}}
{{CommentsModel.TotalCount}} Comments
Your Comment