Working with Pipes in AngularJS2
Pipes in Angular 2 surpasses the filters in AngularJS 1.
In JavaScript, there is a filter() method in built-in Arrays that creates or returns an Array that passes a test (which is usually provided as a function). For example, if you have an array of several numbers, and you want to get the Array that satisfies that all the numbers are greater than 10, then you will create a function for the test (let's say greaterThan()) and then pass it to the filter() method to get the desired Array.
Filters have been a part of the web development process. There are times when you want the user or client on the website to search about the product or some information, but you want the results to be relevant to the search, perhaps you want to only show products that share the same category. In this scenario, filters are used to gettting the desired results according to some rule or test.
Angular 2 uses pipes over filters (yes, "pipes" sound cool!) which basically allows you to use the "pipe" character | in the view (or the HTML side) that transforms the text according to the rules of the pipes. There are several built-in pipes, but additional pipes can be created as well with ease, thus allowing you to transform your text to the next level!
In this article, I am going to show you how to work with Pipes in AngularJS 2, and how to create custom pipes. Let's get started!
Working with Pipes in Angular 2
Let's see how the pipes work in Angular 2. All you need to do to use the built-in pipes in your HTML code is to simply provide the specific text with the pipe character | along with the pipe name.
<p>My name is {{name | uppercase}}</p>
Following is the code in "app.component.ts".
app.component.ts
import { Component } from '@angular/core';
@Component({
selector: 'app-component',
template: '<p>New Date is {{ newdate | date }}</p>'
})
export class AppComponent {
newdate = new Date(1988, 3, 15); // April 15, 1988
}
If you noticed the HTML side, I have used "| date" with property newdate that simply transforms the default date format into a clear format. The "date" pipe can only be applied to the instance of the "Data" class.
AngularJS 2 supports some built-in types: DatePipe, UpperCasePipe, LowerCasePipe, CurrencyPipe, and PercentPipe. These pipes should be self-explanatory (i.e., UpperCasePipe transforms the text to upper case, etc.). "date" pipe comes from DatePipe.
You can also parameterize a pipe by giving optional parameters to change the output. In the above example, change the HTML side to the following:
<p>New Date is {{ newdate| date:"MM/dd/yy" }} </p>
Here, the optional parameters will change the format of the date according to the rules provided in it.
You can also chain several pipes together.
<p>New Date is {{ newdate| date:"MM/dd/yy" | uppercase }} </p>
You can chain as many pipes as you want, just provide an additional pipe after the previous pipe.
Creating the custom pipes
So far, you have learned about built-in pipes. The cool thing about Pipes in AngularJS 2 is that you can create your own custom pipes that can be applied to any HTML data (unless you specify explicitly). All you need to do is create a new component (or use an already established component) implement the PipeTransform interface and then define the transform() method that will set the rules or conditions for the pipe. The @Pipe decorator will be used to give the name to the pipe that will be used in the HTML view side (or the template expressions).
In the following code, I have created a new pipe called "SquarePipe".
import { Pipe, PipeTransform } from '@angular/core';
@Pipe({
name: 'square'
})
export class SquarePipe implements PipeTransform {
transform(value: any, args?: any): any {
return value * value;
}
}
Now, you can use the pipe in your HTML code:
<p>Square of {{number}} is {{ number | square }}</p>
Remember to include the SquarePipe in declarations array of the AppModule if you want it to be used in the whole application.
Pure and Impure Pipes
We know that there are two sides to everything. In AngularJS 2, Pipes come in two flavors: Pure and Impure Pipes. Sounds confusing? Pipes are pure by default (unless you put the dirty socks on them!). We can set the pipe to pure by setting the pure flag to false.
AngularJS 2 executes the pure pipes when it detects the changes in the text or the input. Impure pipes on the other hand will be called everytime some keystroke or mouse movement happens. Having said that, pure pipes are faster, impure pipes are slower and may take a lot of the time. So, the question would be: Why use impure pipes if the pure pipes are faster?
In certain cases, impure pipes are necessary. For example, if you are using HTTP methods to send and receive the information from some API, then you need to reset the value every time an event or change occurs in the server. in AngularJS 2, AsyncPipe is an interesting example of it, which subscribes to an Observable or Promise and returns the latest value it has emitted (we will see the example later).
Putting it all together
Now, let's create a project and put everything we have learnt so far into it. Create a new project called "AngularJS2-Pipes":
$ng new AngularJS2-Pipes
This is what your "app.component.ts" and "app.component.html" should look like:
app.component.ts
import { Component } from '@angular/core';
@Component({
selector: 'app-root',
templateUrl: './app.component.html',
styleUrls: ['./app.component.css']
})
export class AppComponent {
newdate = new Date(1988, 3, 15); // April 15, 1988
}
app.component.html
<h1>
<p>New Date is {{ newdate | date }}</p>
<p>New Date is {{ newdate| date:"MM/dd/yy" }}</p>
<p>New Date is {{ newdate | date | uppercase}}</p>
</h1>
You can "ng serve" to test the results. Now, we need to create custom pipes. Let's first create the SquarePipe:
$ng g pipe square
Copy the following code in "square.pipe.ts":
square.pipe.ts
import { Pipe, PipeTransform } from '@angular/core';
@Pipe({
name: 'square'
})
export class SquarePipe implements PipeTransform {
transform(value: any, args?: any): any {
return value * value;
}
}
In order to test our new Pipe, add the following line in "app.component.ts" (make sure to add it inside the AppComponent class):
number = 3;
Now, add the following line in "app.component.html":
<p>Square of {{number}} is {{ number | square }}</p>
You will notice that the provided number will show the "squared" value. Now, create a new pipe called "CapitalizePipe":
$ng g pipe capitalize
Now copy the following code to "capitalize.pipe.ts":
capitalize.pipe.ts
import { Pipe, PipeTransform } from '@angular/core';
@Pipe({name: 'capitalize'})
export class CapitalizePipe implements PipeTransform {
transform(value: string, args: string[]): any {
if (!value) return value;
return value.replace(/\w\S*/g, function(txt) {
return txt.charAt(0).toUpperCase() + txt.substr(1).toLowerCase();
});
}
}
Now, we will add the promises in our component to test the async pipe to show you the impure pipes quality. Add the following code in "app.component.ts":
promise: Promise<string>;
constructor() {
// The text will be shown in 2 seconds
this.promise = new Promise(function(resolve, reject) {
setTimeout(function() {
resolve("This is coming from Promise!");
}, 2000)
});
}
async is a built-in pipe and by default is set to impure, so you don't need to change the flag to make it impure.
Now, let's put it all together. Following is the content of "app.component.ts":
app.component.ts
import { Component } from '@angular/core';
import { Pipe, PipeTransform } from '@angular/core';
import { TempConvertPipe } from "./temp-convert.pipe";
@Component({
selector: 'app-root',
templateUrl: './app.component.html',
styleUrls: ['./app.component.css']
})
export class AppComponent {
newdate = new Date(1992, 2, 12); // March 12, 1988
// Default number is 3
number = 3;
name = "richard john";
date: Date;
temperature: number;
promise: Promise<string>;
constructor() {
this.date = new Date();
this.temperature = 90;
// The text will be shown in 2 seconds
this.promise = new Promise(function(resolve, reject) {
setTimeout(function() {
resolve("This is coming from Promise!");
}, 2000)
});
}
}
Here, you can see that I have defined some properties that we will use in our View side.
Now add the following code in "app.component.html":
app.component.html
<p>Date is {{ newdate | date }}</p>
<p>Date is {{ newdate | date:"MM/dd/yy" }}</p>
<p>Date is {{ newdate | date:'mediumDate' }}</p>
<p>Date is {{ newdate | date:'yMMMMd' }}</p>
<p>Date is {{ newdate | date:'shortTime' }}</p>
<p>Date is {{ newdate | date | uppercase}}</p>
<p>Square of {{number}} is {{ number | square }}</p>
<p>My name is <strong>{{ name | capitalize }}</strong>.</p>
<h1>Async</h1>
<p>{{ promise | async }}</p>
Make sure to include all the Pipes to the "declarations" in app.module.ts as that will allow you to use the pipes in your whole application (this is very useful if you have pipes like translate pipes that need to be used globally!), but if you are using AngularJS 2 CLI, then it should be already added the moment you create new pipes.
.....and that's it! You can see the whole source code here (https://github.com/danyalzia/AngularJS2-Pipes).
Beyond it...
There are many interesting implementations of pipes that you can use right away. For example, there is a project called "ng2-translate" that allows you to use new translate pipe to translate the text to any language! Check it out here (https://github.com/ocombe/ng2-translate). Apart from it, AngularJ 2 pipes library (https://github.com/fknop/angular-pipes) provides several useful pipes for your next professional projects!
Conclusion
Thus far, you can see that I have highlighted the powerful feature of Pipes in AngularJS 2 and what makes it different from the filters in AngularJS 1. There is no doubt that usecases of Pipes in AngularJS 2 are unlimited and they are only limited within the limits of your imagination. Custom or global pipes ease the many tasks even more, let alone the projects that implement the pipes featured to create new useful pipes!
If you have any questions regarding Pipes, then please ask so in the comment section below!
Recent Stories
Top DiscoverSDK Experts
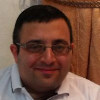
Compare Products
Select up to three two products to compare by clicking on the compare icon () of each product.
{{compareToolModel.Error}}
{{CommentsModel.TotalCount}} Comments
Your Comment