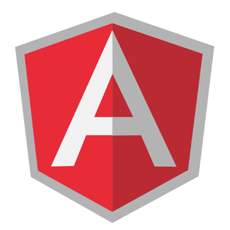
What is it all about?
Angularjs is a free JavaScript open-source framework for client-side MVC or MVVM architecture, along with components that are commonly used in Internet Applications based on the expansion of HTML with new tags.
Key Features
Lets you write client-side web applications as if you had a smarter browser. It lets you use good old HTML (or HAML, Jade and friends!) as your template language and lets you extend HTML’s syntax to express your application’s components clearly and succinctly. Automatically synchronizes data from your UI (view) with your JavaScript objects (model) through 2-way data binding. To help you structure your application better and make it easy to test, AngularJS teaches the browser how to do dependency injection and inversion of control. Helps with server-side communication, taming async callbacks with promises and deferreds. Makes client-side navigation and deeplinking with hashbang urls or HTML5 pushState a piece of cake. Client-side MVC modular style. Models are simple objects in your JavaScript controller functions which are manipulated on the views using scopes. Two way binding + custom watchers for variable change Extension to HTML, i.e. Directives in the shape of custom HTML tags or attributes. Dependency Injection to order Services etc on the go in your code files Best SPA (Single Page Application) modal with services like $routeProvider and $location. Different built in services like $http, $resource, $view etc Filters, built-in e.g. for currency, orderby and user defined.
Resources
Resource Type |
Link |
---|---|
Wikipedia | https://en.wikipedia.org/wiki/AngularJS |
Code Example | http://www.w3schools.com/angular/ |
YouTube Video | https://youtu.be/zKkUN-mJtPQ |
Compare Products
Select up to three two products to compare by clicking on the compare icon () of each product.
{{compareToolModel.Error}}
{{CommentsModel.TotalCount}} Comments
Your Comment