Spring - Dispatcher Servlet and Bean Definition
In this tutorial, we will dive deep into Spring Dispatcher Servlet, how it works, and the Bean Definition methods in Spring. Check out our "getting starting with Spring" if you need to bursh up before hand.
As already studied in our previous articles of the series, Spring framework is an open source Java platform that provides MVC infrastructure support for developing robust Java applications very easily and very rapidly using recommended MVC pattern.
Dispatcher Servlet
To handle a request and provide a response, DispatcherServlet handles the flow of the request through the web app and finally delivers the response back to the client. During the flow, the request stops at many places and many events occur. Let us go through each of them:
- After receiving an HTTP request, DispatcherServlet consults the HandlerMapping to call the appropriate Controller.
- The Controller takes the request and calls the appropriate service methods based on used GET or POST method. The service method will set model data based on defined business logic and returns view name to the DispatcherServlet.
- The DispatcherServlet will take help from ViewResolver to pickup the defined view for the request.
- Once the view is finalized, The DispatcherServlet passes the model data to the view which is finally rendered on the browser.
All the above mentioned components i.e. HandlerMapping, Controller and ViewResolver are parts of WebApplicationContext which is an extension of the plain ApplicationContext with some extra features necessary for web applications.
We might have:
- a file /WEB-INF/jsp/pages/Home.jsp
- and a method on a class:
@RequestMapping(value="/pages/Home.html")
private ModelMap buildHome() {
return somestuff;
}
The Dispatcher servlet is the bit that "knows" to call that method when a browser requests the page, and to combine its results with the matching JSP file to make an html document.
How it accomplishes this varies widely with configuration and Spring version.
There's also no reason the end result has to be web pages. It can do the same thing to locate RMI endpoints, handle SOAP requests, anything that can come into a servlet.
>>> A web application can define any number of DispatcherServlets.
Each servlet will operate in its own namespace, loading its own application context with mappings, handlers, etc. Only the root application context as loaded by ContextLoaderListener, if any, will be shared.
Bean Lifecycle
The objects that form the backbone of our application and that are managed by the Spring IoC container are called beans.
A bean is an object that is instantiated, assembled, and otherwise managed by a Spring IoC container. These beans are created with the configuration metadata that you supply to the container, for example, in the form of XML <bean/> definitions which we have already seen in previous chapters.
The bean definition contains the information called configuration metadata which is needed for the container to know the following:
- How to create a bean
- A bean's lifecycle details
- A bean's dependencies
The following are the stages in a bean’s life cycle.
- Instantiate - The Spring container instantiates the bean.
- Populate properties- Spring IoC container injects the bean’s properties.
- Set Bean Name- Spring container sets the bean name. If the bean implements BeanNameAware, spring container passes the bean’s id to setBeanName() method.
- Set Bean Factory - If the bean implements BeanFactoryAware, Spring container passes the BeanFactory to setBeanFactory().
- Pre Initialization - This stage is also called the bean postprocess. If there are any BeanPostProcessors, the Spring container calls the postProcesserBeforeInitialization() method.
- Initialize beans - If the bean implements IntializingBean, its afterPropertySet() method is called. If the bean has init method declaration, the specified initialization method is called.
- Post Initialization - If BeanPostProcessors is implemented by the bean, the Spring container calls their postProcessAfterinitalization() method.
- Ready to Use - Now the bean is ready to be used by the application.
- Destroy - The bean is destroyed during this stage. If the bean implements DisposableBean, the Spring IoC container will call the destroy() method . If a custom destroy() method is defined, the container calls the specified method.
Although there are many stages in the Spring bean lifecycle, this tutorial will discuss only two important bean lifecycle callback methods which are required at the time of bean initialization and its destruction.
To define setup and teardown methods for a bean, we simply declare them with init-method and/or destroy-method parameters. The init-method attribute specifies a method that is to be called on the bean immediately upon instantiation. Similarly, destroy-method specifies a method that will be called just before a bean is removed from the container.
XML configuration
In our previous ‘Hello World’ article, we have already seen how to configure beans in XML, though, let us do that again here to provide a clear picture:
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://www.springframework.org/schema/beans
http://www.springframework.org/schema/beans/spring-beans-3.0.xsd">
<!-- A simple bean definition -->
<bean id="..." class="...">
<!-- collaborators and configuration for this bean go here -->
</bean>
<!-- A bean definition with lazy init set on -->
<bean id="..." class="..." lazy-init="true">
<!-- collaborators and configuration for this bean go here -->
</bean>
<!-- A bean definition with initialization method -->
<bean id="..." class="..." init-method="...">
<!-- collaborators and configuration for this bean go here -->
</bean>
<!-- A bean definition with destruction method -->
<bean id="..." class="..." destroy-method="...">
<!-- collaborators and configuration for this bean go here -->
</bean>
<!-- more bean definitions go here -->
</beans>
We can also configure Java Beans using pure Java based annotation which we will configure in a later article as it is important to cover some more concepts before we start writing our apps.
Java Beans vs EJB
A JavaBean is just a plain old Java object that conforms to certain conventions including the use of accessor functions (getFoo/setFoo) for member access, provision of a default constructor and a few other things like that.
An Enterprise JavaBean is a component in a Java EE application server which comes in several flavours, the details of which vary by which version of Java EE you're talking about (or, more specifically, which specific set of EJB specifications are involved).
JavaBeans were originally mostly intended to be used in builder tools by providing a known interface which could be looked for through introspection in the tools. They quickly turned into what amounts to a religion, however.
Enterprise JavaBeans are intended to provide encapsulated business logic for enterprise applications within a general container that provided things like session management, security, resource pooling, etc. as services thus allowing the business logic to be (relatively) untainted by these cross-cutting concerns. Whether or not they accomplished this is a matter that is up for debate, given how difficult they were to use at first. More recent versions of the specification have made this easier, however. Legacy apps, though, are still a pain and sadly likely the majority of the EJBs you're likely to encounter.
Conclusion
In this article, we explored Spring Dispatcher Servlet, how it works and the Bean Definition methods in Spring.
We also covered how to configure the beans in an XML file. We will continue the discussion on Bean Lifecycle using Java based annotations in coming posts.
Contine to the next article in the series - Bean Scope and Post processor.
Recent Stories
Top DiscoverSDK Experts
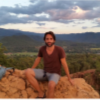
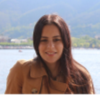
Compare Products
Select up to three two products to compare by clicking on the compare icon () of each product.
{{compareToolModel.Error}}
{{CommentsModel.TotalCount}} Comments
Your Comment