Getting started with Spring
In this tutorial, we will get started using Spring framework and develop a Hello World application. You can check out our introduction to Spring framework if havne't already.
As already mentioned in our first article in this series, Spring framework is an open source Java platform that provides MVC infrastructure support for developing robust Java applications very easily and very rapidly using the recommended MVC pattern.
Hello World
Now let's start some actual programming with Spring Framework. Before you start writing your first example using Spring framework, you need to make sure that your Spring environment is setup properly.
Create a new project
The first step is to create a Java Project using Eclipse IDE. Follow the option File -> New -> Project and finally select Java Project wizardg from the wizard list. Now name your project as HelloWorld.
Add external libraries
Now use Add External JARs button available under Libraries tab to add the following core JARs from Spring Framework and Common Logging installation directories:
- commons-logging-1.1.1
- spring-aop-4.1.6.RELEASE
- spring-aspects-4.1.6.RELEASE
- spring-beans-4.1.6.RELEASE
- spring-context-4.1.6.RELEASE
- spring-context-support-4.1.6.RELEASE
- spring-core-4.1.6.RELEASE
- spring-expression-4.1.6.RELEASE
- spring-instrument-4.1.6.RELEASE
- spring-instrument-tomcat-4.1.6.RELEASE
- spring-jdbc-4.1.6.RELEASE
- spring-jms-4.1.6.RELEASE
- spring-messaging-4.1.6.RELEASE
- spring-orm-4.1.6.RELEASE
- spring-oxm-4.1.6.RELEASE
- spring-test-4.1.6.RELEASE
- spring-tx-4.1.6.RELEASE
- spring-web-4.1.6.RELEASE
- spring-webmvc-4.1.6.RELEASE
- spring-webmvc-portlet-4.1.6.RELEASE
- spring-websocket-4.1.6.RELEASE
Add source code files
Create a new package named com.discoversdk package. Also, create two files:
- HelloWorld.java
- MainApp.java
public class HelloWorld {
private String message;
public void setMessage(String message){
this.message = message;
}
public void getMessage(){
System.out.println("Your Message : " + message);
}
}
In the second class, put following code:
import org.springframework.context.ApplicationContext;
import org.springframework.context.support.ClassPathXmlApplicationContext;
public class MainApp {
public static void main(String[] args) {
ApplicationContext context =
new ClassPathXmlApplicationContext("Beans.xml");
HelloWorld obj = (HelloWorld) context.getBean("helloWorld");
obj.getMessage();
}
}
There are few points here that are necessary for you to understand:
- Firstly, we will need to create application context where we used framework API ClassPathXmlApplicationContext(). This API loads beans configuration file and eventually based on the provided API, it takes care of creating and initializing all the objects i.e. the beans mentioned in the configuration file.
- Second step is used to get required bean using getBean() method of the created context. This method uses bean ID to return a generic object which finally can be casted to an actual object. Once we have object, we can use this object to call any class method.
Create Bean class
The Beans.xml is used to assign unique IDs to different beans and to control the creation of objects with different values without impacting any of the Spring source files. For example, using the file below we can pass any value for the "message" variable and so we can print different values of message without impacting the HelloWorld.java and MainApp.java files. Let's take a look how it works:
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://www.springframework.org/schema/beans
http://www.springframework.org/schema/beans/spring-beans-3.0.xsd">
<bean id="helloWorld" class="com.discoversdk.HelloWorld">
<property name="message" value="Hello World!"/>
</bean>
</beans>
When Spring application gets loaded into the memory, Framework makes use of the above configuration file to create all the beans defined and assigns them a unique ID as defined in <bean> tag. We can use the <property> tag to pass the values of different variables used at the time of object creation.
Run the program
Next, just hit the run button to run the project.
Congratulations, you have successfully created your first Spring Application. You can see the flexibility of the above Spring application by changing the value of "message" property and keeping both the source files unchanged. Further, let us start doing something more interesting in next few chapters.
IoC container
The Spring container is at the core of the Spring Framework. The container will make the objects, handle dependencies, configure them, and manage their complete lifecycle from birth till death. The Spring container uses dependency injection (DI) to manage the components that make up an application. These objects are called Spring Beans which we will discuss in future article.
The container gets its instructions on what objects to create, configure, and assemble by reading configuration metadata provided by us, either from an XML file or Java based annotations. The configuration metadata can be represented either by XML, Java annotations, or Java code. The Spring IoC container makes use of Java POJO classes and configuration metadata to produce a fully configured and executable system or application.
The ApplicationContext container includes all functionality of the BeanFactory container, so it is generally recommended over the BeanFactory. BeanFactory can still be used for lightweight applications like mobile devices or applet based applications where data volume and speed is significant.
Servlet vs Ioc Container
They are very different concepts although their names are similar.
A Servlet Container or Web Container (like Tomcat) is an implementation of various Java EE specifications like Java Servlet, JSP, etc. Put simply, it is an environment where Java web applications can live. A web server + Java support.
A Spring Container on the other hand, is the core and the engine of the Spring Framework. In fact it is an IoC Container which handles Spring applications life cycles creating new beans and injecting dependencies.
Because a Spring application can be a web-app (or a classical console application), a Spring Container can "live" inside a Web Container.
A servlet container is simply a web server that runs your java code. Ex: Glassfish, Tomcat, Weblogic, Jetty.
IoC (Inversion of Control) is a design pattern whose primary goal is to reduce coupling between dependencies. An IoC container is a framework that assists with dependency injection and allows you to easily implement those IoC patterns. Some examples are Spring, Dagger, and Guice.
In a normal program, you create methods and those methods create whatever objects they need (dependencies) and then use them. This results in high coupling. Your class controls how those objects are created, configured, and used.
In IoC, the IoC container creates the dependant objects for you, then 'injects' them into the class. The class does not need to know, nor care about how it's dependencies were created. It just has a reference to them and can use them as if it created them itself, typically provided in the constructor for the class. In this case, the dependencies essentially configured and created themselves (through the IoC container). Instead of your class controlling how they are created, the dependencies themselves control their own configuration, resulting in the 'inversion of control'.
Conclusion
In this article, we started by creating a Hello World app and then looking at the IoC container in Spring. Then, we continued by comparing the Servlet and the powerful Spring Container.
Continue to part 3 - Dispatcher Servlet and Bean Definition
Recent Stories
Top DiscoverSDK Experts
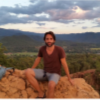
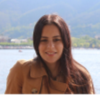
Compare Products
Select up to three two products to compare by clicking on the compare icon () of each product.
{{compareToolModel.Error}}
{{CommentsModel.TotalCount}} Comments
Your Comment