React Stateful Components
In the last article, we learned about components. But, there might be a slight problem with those components in that they’re too simple and can only display things. But most components that we need for our projects will have to be interactive in some way and as such will need to be able to store some kind of data that can change. This data might come from outside, from inside, or anyplace really, but our components must be able to store data. In React, these components that store data are called state, while simple components that are used only for displaying content are called stateless. Got it? Well, if you know how to work with parameters, which we covered earlier in the series, you’ll be able to handle states as well. Long story short, states in React are objects that contain data, and this data can be changed.
Let’s get into an example—everything is easier when we’ve got some code to look at. Let’s pretend we work at a shitty company with a failing new cybersecurity product—one that will show a component that counts the number of cyber attacks in a given country. Company experts (i.e. the marketing manager who very well might be stoned) checked and found that there were 1200 attacks. OK, that’s pretty easy to implement. We’ll make two components, the first of which will be CurrentCyberAttackDisplay that wraps the number and gives us a graphic read out, and the second will be CurrentCyberAttackCounter that only provides the number. So the first contains the second within it. And the code looks a little something like this:
class CurrentCyberAttackDisplay extends React.Component {
render() {
const bigStyle = {
backgroundColor: 'black',
borderRadius: 10,
color: 'silver',
fontSize: '42px',
textAlign: 'center',
width: 250,
};
const smallStyle = {
color: 'gold',
fontSize: '25px',
};
return <div style={bigStyle}>
<CurrentCyberAttackCounter />
<div style={smallStyle}>Cyber attacks per day</div>
</div>
}
}
class CurrentCyberAttackCounter extends React.Component {
render() {
const content = <div>1200</div> // This is JSX
return content;
}
}
const target = document.getElementById('content');
ReactDOM.render(
<CurrentCyberAttackDisplay />,
target
);
At this point of our series on React, you should be able to thoroughly understand the code above. If you didn’t then this is a good time to go back over the previous articles.
What’s the problem with this component? Let’s say the marketing manager says that he wants the number to go up by 100 every 20 seconds, in order to scare and pressure the customer into buying their shitty cyber product. How can we do this? There’s no other way than using state, meaning a variable in the CurrentCyberAttackCounter component that will take 1200, and running a method that will increase this variable by 100 every 20 seconds. So, how do we do this?
The first step—creating and displaying a state. This is as easy as could be and all we need is to make use of a constructor. Not sure what that is? Just check out our article on Keepin it ‘Classy’ in ES6, but ignore the punny title—it’s from the terrible copywriter inside of me.
Inside the constructor that will be in CurrentCyberAttackCounter, we go into the state 1200 times.
class CurrentCyberAttackCounter extends React.Component {
constructor(props) {
super(props);
this.state = {
attackNumber: 1200,
};
}
render() {
const content =
{ this.state.attackNumber }
// This is JSX
return content;
}
}
This is, well, pretty easy, no? No different from display, except now we have a variable that we can use. How? Just write a simple method called timerTick that increases this.state.attackNumber by exactly 10.
class CurrentCyberAttackCounter extends React.Component {
constructor(props) {
super(props);
this.state = {
attackNumber: 1200,
};
}
timerTick() {
this.setState({attackNumber: this.state.attackNumber + 10});
}
render() {
const content =
{ this.state.attackNumber }
// This is JSX
return content;
}
}
That’s great, but who exactly calls this method? If no one activates timerTick it would just lie around uselessly. We need a function that will immediately be activated when the component starts to render. This is exactly why we have the React API. Just like render, we have componentDidMount that runs solely when the component is ready for action. We need to put in everything we want to be ready to be used right after the component works. In our case, the call to timeTick, or to be more exact, we’ll use setInterval to call timerTick every second (1000 milliseconds). This will look like so:
class CurrentCyberAttackCounter extends React.Component {
constructor(props) {
super(props);
this.state = {
attackNumber: 1200,
};
}
timerTick() {
this.setState({attackNumber: this.state.attackNumber + 10});
}
componentDidMount() {
setInterval(this.timerTick, 1000);
}
render() {
const content =
{ this.state.attackNumber }
// This is JSX
return content;
}
}
Hold on there just one minute, there’s still one more thing. If I run the code above it won’t work and we’ll get an error.
Why? Because when timeTick runs in the setInterval scope, it can’t access this. We need to to a bind using:
this.timerTick = this.timerTick.bind(this);
If you understand why, awesome! If not, you probably need to go over scope because it’s a very important point. It’s critical to understand why we need bind because we use scope all the time in React (and in general in modern JavaScript), and if you don’t know what you’re doing you’ll be running into errors left and right. The rule is: if a function or method that you are using doesn’t know how to get to the variable, then you have a scope problem and you need to use bind or call/apply.
So here you have it—our complete code:
class CurrentCyberAttackDisplay extends React.Component {
render() {
const bigStyle = {
backgroundColor: 'black',
borderRadius: 10,
color: 'silver',
fontSize: '42px',
textAlign: 'center',
width: 250,
};
const smallStyle = {
color: 'gold',
fontSize: '25px',
};
return
Cyber attacks per day
}
}
class CurrentCyberAttackCounter extends React.Component {
constructor(props) {
super(props);
this.state = {
attackNumber: 1200,
};
this.timerTick = this.timerTick.bind(this);
}
timerTick() {
this.setState({attackNumber: this.state.attackNumber + 10});
}
componentDidMount() {
setInterval(this.timerTick, 1000);
}
render() {
const content =
{ this.state.attackNumber }
// This is JSX
return content;
}
}
const target = document.getElementById('content');
ReactDOM.render(
,
target
);
And it even works! Try for yourself:
Using just one example we’ve seen how to work with stateful components in React. We learned about constructor which is important for initialization of the state and about componentDidMount through which we managed the state. In the next article, we’ll take a look at events in React components and do some more interactive things. Promise.
Previous Article: React Component Design | Next article: React Components w/ Events |
About the author: Ran Bar-Zik is an experienced web developer whose personal blog, Internet Israel, features articles and guides on Node.js, MongoDB, Git, SASS, jQuery, HTML 5, MySQL, and more. Translation of the original article by Aaron Raizen.
Recent Stories
Top DiscoverSDK Experts
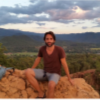
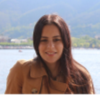
Compare Products
Select up to three two products to compare by clicking on the compare icon () of each product.
{{compareToolModel.Error}}
{{CommentsModel.TotalCount}} Comments
Your Comment