New Features in ES2016
ECMAScript 6 is the last numbered version of ECMAScript—the schema upon which JavaScript is built. The versions that come after are named according to the year in which they are released, with new modules begin released each year that will expand the language. This is significant since JavaScript has become one of the most important structural components of the net. In 2016, the ES2016 standard was released, then ES2017 in 2017 and so on.
And just who releases these standards? In an article on ES6 I wrote about ECMA—the organization behind ECMAScript. The actual people that decide upon the standard are called the Technical Committee 39 (TC39). The committee members are usually made up of representatives from large bodies, (Google, Microsoft, Intel, etc.) who debate and then agree upon the changes and new features in the standard. Every idea goes through 4 stages.
Stage 0 - the idea is proposed without complex specifications and without the support of an organization.
Stage 1 - the idea gets the support of one of the members present at the committee and complete technical specifications.
Stage 2 - first draft. The idea has been discussed and accepted along with the specifications. From here there likely won’t be major changes to the API or in the implementation of the change or feature.
Stage 3 - candidate for entry. The idea is almost part of the standard and other committee members must sign off on it. At this point, we can assume there won’t be any changes unless there is a critical issue with implementation.
Stage 4 - The idea becomes part of the standard.
The procedure is pretty transparent and we can watch the creation of the new standards and even try to write specifications for it. Of course, the creators of the popular browsers don’t always rush to implement the changes of the new standard—surely we’re talking about future standards. Today, as opposed to the jurassic era of Internet Explorer, we don't need to wait years for the standard to be accepted—we may want to use a cool new feature of ES2017 that's in stage 4 in 2017. Just for this we have Babel—you can read the full guide starting with the introduction. Babel has polyfills and plugins that will convert code written in ES6 and later versions to ES5, which can be run by all browsers. In principle, the official presets contain only the features that are in stage 4, but there are also add-ons of earlier stages if you want. Personally, I'd rather jump off a bridge than use something from stage 0 in production, everybody's is free to make their own choices.
I'm going to assume that you are familiar with ES6 and with Babel, or that you at least bothered to read our series on them. This would mean that everything we talked about so far doesn't sound like Chinese. If you're not already familiar with presets and polyfills, a few minutes of reading should get you up to speed.
Naturally, after the enormous version of ES6, the ES2016 standard is quite trim and really only contains just two central features. Today, all major browsers and environments support these new features except... Node version 4 and lower (which is pretty old) and of course Microsoft’s wondrous browser IE 11, aka the angel of death. How do I know? This site shows me browser support for various features. In order to be certain that our code will work in all environments, we can use Babel, especially with the 2015 and 2016 presets. 2015 will cover all of ES6 and 2016 will cover ES2016. Some of you may ask why we need to write both of them. Why doesn't 2016 have all of 2015 inside of it? This is because version 6 and higher of Babel are very modular, and the presets are basically a collection of add-ons. The 2016 preset contains only the features of ES2016, and doesn't take 2015 along with it.
Installing the presets with Babel is simple:
npm install --save-dev babel-cli babel-preset-es2015 babel-preset-es2016
To run it use this command:
node_modules/.bin/babel src -d lib --presets es2016,es2015 -w
All code that we write in the scr directory is converted immediately to ES5.
So let's start. The first feature of ES2016 is...
Exponentiation Operator
This is pretty straightforward. If we want to write 2 to the power of 3, we need to use the following:
Math.pow(2, 3);
The ES2016 standard allows us to use two asterisks:
2 ** 3
Here is an example of simple code in ES2016:
let a = 2;
let b = 3;
let c = a ** b;
console.log(c); //8
If we compile this to ES5, we'll see that our asterisks were changed to power of functions:
"use strict";
var a = 2;
var b = 3;
var c = Math.pow(a, b);
console.log(c); //8
OK, and now for the second feature...
Searching for Array Members
Long story short, this feature helps us find members of an array and returns true or false. Here's the example:
let a = ['a', 'b', 'c', 'd', 'last'];
let x = a.includes('d');
let y = a.includes('lo po');
let z = a.includes('b', 2);
console.log(x); //true
console.log(y); //false
console.log(z); //false, because we start from 0
We receive true or false. Notice that, as opposed to indexOf, we don't get the place in the array. Additionally, there is an offset which is the second parameter. Here we can define where we want to start. Pretty fun! Since we're talking about an addition to Array, this is not being handled by Babel but by its polyfills.
If you want to mess around with it, you can here:
And that's about it. We you expecting a lot more? In the coming articles of this series, we'll look at the new features of ES2017 and later versions. Notice that I'm only looking at features from stage 4 (those that are certain to go into the standard).
About the author: Ran Bar-Zik is an experienced web developer whose personal blog, Internet Israel, features articles and guides on Node.js, MongoDB, Git, SASS, jQuery, HTML 5, MySQL, and more. Translation of the original article by Aaron Raizen.
Recent Stories
Top DiscoverSDK Experts
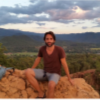
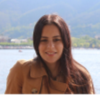
Compare Products
Select up to three two products to compare by clicking on the compare icon () of each product.
{{compareToolModel.Error}}
{{CommentsModel.TotalCount}} Comments
Your Comment