Introduction to Babel
Babel is a JavaScript compiler that allows you to code in ES2015, with encryption (lots of encryption), and convert JavaScript files from a newer standard to ES5, the older standard. In this series of articles, we’ll be covering Babel version 6.5—from explaining basic concepts and up through plugins and presets.
In this article I’ll explain why one might need Babel, and then we’ll take a look at a simple example. If you'd like to get firmaliar with ES2015 a.k.a ECMAScript 6 or just ES 6, check out our article: intro to ECMAScript 6.
Babel is a tool that solves a significant problem for us—a lack of support by browsers and other platforms (Node.js for example) for many of the advanced features of ECMAScript 6, and for features of newer versions of ECMAScript that are numbered according to their year: ES2016 and ES2017 (with more certainly on the way). As you’re probably aware, for years now web developers have had to deal with the fact that new standard versions are being released, but it takes far too long for web browsers to get up to speed. So instead of using cool new features like generators or templating, we’re stuck using the old standard since some customers may have laughably outdated versions of Internet Explorer some computers don’t have modern browsers, or the Node.js version running on the server may be outdated.
So how do we solve this problem? There are a few ways. One popular method would be to use a process that takes JavaScript code written according to the new standard and transpiles it. This means the code is converted to new, different code, on the same abstraction layer (if this is making you dizzy, just think of it as compiling). This transpiled code will contain polyfills if need be, or be written in a way that the code will work with the older standard.
To demonstrate this point, have a look at the following illustration:
ES6, ES2016, and ES2017 are turned into ES5 with polyfills if need be
What does this mean exactly? In practice, first you write JavaScript according to the new standard. Then when finished, or while writing, you run Babel on the files and compile them to the ECMAScript 5 standard, which is a standard that all browsers and versions of Node.js support without issue. If something arises in the new standard that can’t be supported by ECMSScript 5, the Babel library makes sure to bring in polyfills—special JavaScript code that makes a kind of detour and allows for the new standard. The applications contain the compiled files and not the original ones, thus leaving you free from worry about all kinds of incompatibility problems.
So let’s check out an example! Say we have some code written in ES6 (which BTW is short for ECMAScript 6) and we want to be certain that it will run on Node.js version 0.1.2 which, despite being well past its prime, it still found on many servers that run node.
Here is some code:
let customer = { name: "Moshe" },
message = `Hello ${customer.name}`;
console.log(message); //Hello Moshe
If you’re familiar with ES6 you’ll understand the significance of the code above. What it does is make use of JavaScript templating. And yet this simple code, that works perfectly well in Chrome, Firefox and even Edge (you’re welcome to copy and paste it into the console if you don’t believe me), won’t work in Node.js 0.1.2, or in Explorer 11, which is the browser used in most companies and corporations.
But if we run Babel on this code, it will convert the code to ES5 allowing older systems and browsers to run it. So how does Babel do this? The code above would be converted into something like this:
"use strict";
var customer = { name: "Moshe" },
message = "Hello " + customer.name;
console.log(message); //Hello Moshe
So how do we use it? Well, first just go to Babel’s site and use their interactive demo. Paste in some ES6 code on the left and see the results in ES5 on the right.
Obviously, the last thing that we want to do after writing code is to copy & paste it somewhere. The demo above is just to show what Babel can do and how it works. In reality and outside of the Hello World! universe, we need these things to run somewhere as part of the build process.
In the next article we’ll take a look at Babel with Node.js and how to integrate it into our development process.
Click here for the next article: Getting started with CLI |
About the author: Ran Bar-Zik is an experienced web developer whose personal blog, Internet Israel, features articles and guides on Node.js, MongoDB, Git, SASS, jQuery, HTML 5, MySQL, and more. Translation of the original article by Aaron Raizen.
Recent Stories
Top DiscoverSDK Experts
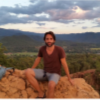
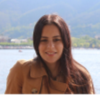
Compare Products
Select up to three two products to compare by clicking on the compare icon () of each product.
{{compareToolModel.Error}}
{{CommentsModel.TotalCount}} Comments
Your Comment