Babel - getting started with CLI
One of the easiest ways to work with Babel is via Node.js CLI options. Don’t be alarmed—even if you’re a front-end developer who’s not too familiar with Node.js, it’s still one of the simplest ways to work with Babel like a grown up. No need for previous knowledge in Node, we’ll cover all you need to know to get going. If you’re already proficient in Node.js, feel free to skip ahead to the section called Installing the Module.
So, all the cool kids that already know Node.js are gone? OK, then I can level with you. The truth is that Node.js is JavaScript that can run on your OS and carry out a wide variety of tasks. For instance, it can perform optimization to pictures, convert SASS files to CSS, or compress JavaScript files, and a lot more. One thing it does particularly well is run Babel and convert ES5 files to ES6.
You can easily install Node.js on Windows, Mac, or Linux. It’s really not a big effort. Just head on over to the Node.js site, install the version for your machine, and that’s really all there is to it. If the installation was successful, you should be able to just open the console/command line and type:
node -v
See a number? Awesome. You now have Node.js on the OS, and can now run all of Node’s operations. But how? Well, this depends on the module.
Installing the Module
Let’s open a folder, for instance babelProject which is the main folder of our project. We’ll create a file in there call package.json. To do this we’ll run:
npm init
If you don’t already know, package.json is a heavily used file in Node that contains all of the installation and operating instructions of the various modules that Node uses in a project. If it’s not a Node project, but still uses an aspect of Node, we’ll need the package.json file.
Let’s make a folder called src with a file called index.js that will contain something like this:
let customer = { name: "Moshe" },
message = `Hello ${customer.name}`;
console.log(message); //Hello Moshe
This file is our application file. It can be a standalone JS file or a JS file that appears in the SRC of an HTML page. It’s not important—a JavaScript file is still a JavaScript file and the code above will not work in Internet Explorer 11, whether it’s part of the front-end or in Node.js 0.1.2 as part of the back-end. But we want it to work. Luckily, as I explained in the previous article, that’s exactly what we have Babel for.
I’m going to head on over to Babel’s site and go to Setup. There I’m asked to choose my versions. In this case, we want CLI (command line interface). When we click on it, we’re taken to a page with installation instructions. You can follow them on Babel’s site but we’ll cover them here just as well.
In order to install Babel CLI, we’ll need to first install the local module for our project. Let’s go back to our first folder, bableProject, and type:
npm install --save-dev babel-cli babel-preset-es2015
This does two things: installs the files of the babel-cli module and the Babel plugins called babel-preset-es2015, and inserts instructions for installing the module to package.json. We’ll expand upon the plugin a little later on.
Now we can start working. Type the following into the command line:
./node_modules/.bin/babel src -d lib –presets es2015
Looking at the file, we see that we have a folder called lib and there is a file called index.js. This file contains the ES5 version of the ES6 code that we wrote!
"use strict";
var customer = { name: "Moshe" },
message = "Hello " + customer.name;
console.log(message); //Hello Moshe
So what exactly happened here? Let’s take a look at the magical line of code that we wrote and break it down a bit.
./node_modules/.bin/babel src -d lib –presets es2015
The part in bold above is essentially the call to the Babel CLI module, just like typing c:\somedirectory\somefile.exe. In this call we’re running the Babel module.
./node_modules/.bin/babel src -d lib –presets es2015
The src is the name of the folder where the source files are to be found. In this case it’s the same src that we made earlier where we put the index.js file.
./node_modules/.bin/babel src -d lib –presets es2015
The minus d’s purpose is to dump all the file in the folder that follow—n this case lib. I could have written any folder name and Babel would have created it.
./node_modules/.bin/babel src -d lib –presets es2015
This is the important part. Here we use a plugin that says “this code is ES2015” (a.k.a. ES6 or ECMAScript 6).
We can also use -w which will cause Babel to listen to the file that’s in the src folder and change the file in lib with each change. If we use -w, it appears that the process doesn’t end, and that with each change to the original file, Babel CLI will report that activity.
When we run the command Babel with -w, it tracks the files in the scr folder and compiles them to the lib folder with each change.
This is just one method to working with Babel and we can incorporate it into slightly more complex build processes. But this is a foundation with which we can begin to work. In the next article we’ll talk a bit about sourcemap, so check back soon.
Previous article: Introduction to Babel | Next article: Creating a souremap and settings file |
About the author: Ran Bar-Zik is an experienced web developer whose personal blog, Internet Israel, features articles and guides on Node.js, MongoDB, Git, SASS, jQuery, HTML 5, MySQL, and more. Translation of the original article by Aaron Raizen.
Recent Stories
Top DiscoverSDK Experts
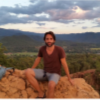
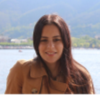
Compare Products
Select up to three two products to compare by clicking on the compare icon () of each product.
{{compareToolModel.Error}}
{{CommentsModel.TotalCount}} Comments
Your Comment