ECMAScript 6 - New Methods
Besides all the cool new features of ES6, there are also several new expansions and methods that can really make our lives easier. Those of you who have used utility libraries like lodash will feel at home here since a large portion of these new expansions actually came from lodash. What's definite is that it's really cool that they exist, and they can really help us solve some significant problems. So let's get started.
Object.assign - add the values of one or more objects to a target object
All in all, it's pretty easy. We have one object which is the target object, and we want to put all the properties of a source object or objects inside of it. With Object.assign it’s really easy and we don't need to use any kind of loop.
var firstObj = {};
var src = {a: 1, b: 2};
var src2 = {d: 1, e: 2};
Object.assign(firstObj, src, src2);
console.log(firstObj);
//Object {a: 1,b: 2,d: 1,e: 2}
Here both src and src2 have the property a. What will go inside the target object is the property from src2, since it comes last in the list of objects that we passed to Object.assign.
Searching through an array with find
Let's say I have an array. It could be a simple array or one with complex objects. Let's say something like this:
var inventory = [
{name: 'apple', quantity: 2},
{name: 'banana', quantity: 0},
{name: 'pineapple', quantity: 5},
{name: 'avocado', quantity: 5},
];
Now let's say I want to search for something inside of the array. For instance, I want to return all of the members in apple. How do we do this? We can use filter or something like that. But the most elegant way is to use the find method that exists solely for arrays. This method takes a simple search function, just like filter. I can pass the name of a function, the function itself, or an arrow function. In the example, you can see all of the possibilities.
var inventory = [
{name: 'apple', quantity: 2},
{name: 'banana', quantity: 0},
{name: 'pineapple', quantity: 5},
{name: 'avocado', quantity: 5},
];
function findApple(el) {
return el.name === 'apple';
}
console.log(inventory.find(findApple)); // { name: 'apple', quantity: 2 }
console.log(inventory.find(function(el) {return el.name === 'apple';})); // { name: 'apple', quantity: 2 }
console.log(inventory.find(el => el.name === 'apple'); // { name: 'apple', quantity: 2 }
In the function, I can carry out all kinds of advanced operations--particularly useful with complex objects. Take note of something important--if there are several objects in the array meet a condition, only the first will be returned. Here's an example:
var inventory = [
{name: 'apple', quantity: 3},
{name: 'banana', quantity: 0},
{name: 'pineapple', quantity: 1},
{name: 'avocado', quantity: 0},
];
function findNotZero(el) {
return el.quantity > 0;
}
console.log(inventory.find(findNotZero)); // { name: 'apple', quantity: 3 }
console.log(inventory.find(function(el) {return el.quantity > 0})); // { name: 'apple', quantity: 3 }
console.log(inventory.find(el => el.quantity > 0)); // { name: 'apple', quantity: 3 }
The condition is an amount that is more than zero. There are a few members that meet this, but we get back just the first that passed the test. If no members meet the condition, we'll get back undefined.
Searching Text Strings
Say we have a string and want to know if it begins with, ends with, or contains something specific. Good news, the reign of terror of the very unintuitive indexOf is over! Now we have several nice methods we can use on a text string to check things. In a case like this, the easiest way is with an example:
Look how nice! Three cute little methods that are very intuitive. startsWith checks if the string starts with whatever we choose, endsWith checks what the strings ends with, and include checks if it contains what we're looking for.
We can also pass offset to these methods, but there's no real reason to do this in my opinion. If you need offset it's a good sign you really need indexOf.
Multiplying Text Strings
If you have a text string that you want to multiply for whatever reason, you can use repeat--a method used only on text strings that multiplies the text as we like.
var myString = 'abc';
console.log(myString.repeat(2)); // "abcabc"
console.log(myString.repeat(3)); // "abcabcabc"
Of course, if you pass 0, you'll get an empty string as a result. If you pass something that's not a number, you'll get an error.
Checking if a number is positive or negative
ES6 didn't leave behind numbers, they too have their own interesting methods. Anyone who's coded more than a line or two of JavaScript know that it's not the best with numbers. So there are a few ES6 methods that are trying to address the issue. One such method is a check to see if a number is positive or negative. I think the example pretty much speaks for itself here:
console.log(Math.sign(7)) // 1
console.log(Math.sign(0)) // 0
console.log(Math.sign(-0)) // -0
console.log(Math.sign(-7)) // -1
console.log(Math.sign(NaN)) // NaN
If the number is negative, we'll get back -1, and if it's positive we'll get a 1. If it's positive zero we'll get a 0 and a -0 for negative zero. And if you're wondering just what positive and negative zero are, it's because the hexadecimal value of zero can be 0000 and can also be 1000. But really there isn't a difference and in JavaScript at least, 0 === -0.
Finding a Number After a Decimal Point
Sometimes when we have a fraction, we need to find the whole number. For example, if we have 42.4 we need to get the 42, or if we have -1.1 we need the 1. Normally we would use an equation like this:
function mathTrunc (x) {
return (x < 0 ? Math.ceil(x) : Math.floor(x));
}
console.log(mathTrunc(42.7)) // 42
console.log(mathTrunc( 0.1)) // 0
With ES6 we can use Math.trunc to do this:
console.log(Math.trunc(42.7)) // 42
console.log(Math.trunc( 0.1)) // 0
There are still many other operations we can do with numbers in ES6, but they start to get a bit esoteric with things like, isFinite or isSafeInteger. So I won't get into them here since the chance that you'll actually need to use them is pretty low.
This wraps up our series on ES6 where we covered all the new features of ECMAScript 6. There are only a few things we didn't cover here, like TypedArray and other things connected to binary data processing, which is a bit out of my range of knowledge.
For more, check back soon for articles on ES2016, ES2017, and ES2018, or get started with our series on Babel.
About the author: Ran Bar-Zik is an experienced web developer whose personal blog, Internet Israel, features articles and guides on Node.js, MongoDB, Git, SASS, jQuery, HTML 5, MySQL, and more. Translation of the original article by Aaron Raizen.
Recent Stories
Top DiscoverSDK Experts
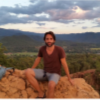
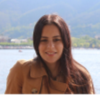
Compare Products
Select up to three two products to compare by clicking on the compare icon () of each product.
{{compareToolModel.Error}}
{{CommentsModel.TotalCount}} Comments
Your Comment