Introduction to Kotlin for Java Developers
This lesson will introduce you to the world of Kotlin, a new programming language by JetBrains which is now officially a first-class citizen for Android.
This means that you are free to develop Android apps in Kotlin just as you would in Java. Apart from Android, you can use Kotlin in server-side applications as well, like with Spring Boot development. Let's look at this wonderful language to start our journey.
Maven Dependency
To start working with Kotlin, we will use a simple Maven based project with the appropriate Maven dependencies. Add the following and only one dependency to start working with Kotlin API:
<dependency>
<groupId>org.jetbrains.kotlin</groupId>
<artifactId>kotlin-stdlib</artifactId>
<version>1.0.4</version>
</dependency>
We will also add a kotlin plugin in our Maven file so that it can decide upon goals it needs to execute. After adding all dependencies, the pom.xml file should look like:
<?xml version="1.0" encoding="UTF-8"?>
<project xmlns="http://maven.apache.org/POM/4.0.0"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>stephan-kotlin</groupId>
<artifactId>kotlin-demo</artifactId>
<version>1.0-SNAPSHOT</version>
<dependencies>
<dependency>
<groupId>org.jetbrains.kotlin</groupId>
<artifactId>kotlin-stdlib</artifactId>
<version>1.0.4</version>
</dependency>
</dependencies>
<build>
<sourceDirectory>${project.basedir}/src/main/kotlin</sourceDirectory>
<testSourceDirectory>${project.basedir}/src/test/kotlin</testSourceDirectory>
<plugins>
<plugin>
<artifactId>kotlin-maven-plugin</artifactId>
<groupId>org.jetbrains.kotlin</groupId>
<version>1.0.4</version>
<executions>
<execution>
<id>compile</id>
<goals>
<goal>compile</goal>
</goals>
</execution>
<execution>
<id>test-compile</id>
<goals>
<goal>test-compile</goal>
</goals>
</execution>
</executions>
</plugin>
</plugins>
</build>
</project>
Also, our directory structure looks like:
Hello World
Starting a tutorial with any programming language should start with a Hello World program. Let us write our first program for this.
Let us look at this program closely:
- Initial package statement is same as in Java. This defines in which package this program is lying.
- Next, we start with a function with fun keyword. Now, functions in Kotlin are first-level citizens as well. This means that functions can exist separately from a class. This is what is happening here.
- Inside the function, we make a simple call to the println(...) method with String arguments.
When we run this program, we can see the magical message.
Hello World!
Getting command line parameters
Now, just like in Java’s main method, we can also pass parameters from the command line and use them in our program. Let us write a simple program to do so:
package com.hello
fun main(args: Array<String>) {
println("Hello ${args[0]}")
}
This program demonstrates 2 things:
- First, how we can use command line arguments as parameters inside our program, simply with an array and 0 based index.
- Second, String formatting. In Kotlin, we can simply insert Strings in our program with using $ followed by curly braces {}.
Data Types and Variables in Kotlin
In Kotlin, we have similar data types as Java. Let us list them here:
- Numbers
- Double (64 bits)
- Float (32 bits)
- Long (64 bits)
- Int (32 bits)
- Short (16 bits)
- Byte (8 bits)
- Characters
- Booleans
- Arrays
- Strings
So, basically all the types are exactly the same in Kotlin as in Java. But defining them is somewhat different. Let us define each of these types here:
var a: Int
a = 21
Here, we simply defined an int variable and then assigned a value to it. We can also define final variables.
Finals in Kotlin
We can also define final constants in Kotlin. We use the val keyword for this:
Of course, these values can never be modified now, just like in Java.
Strings and String formatting
Strings in Kotlin are stored the same way as in Java. The advantage here goes to Kotlin because of the very high formatting capabilities.
Kotlin provides various ways in which strings can be formatted and manipulated. Let us see some tricks here.
Math Calculation during formatting
Kotlin allows you to perform mathematical operations during String formatting:
val five = "five: ${2 + 3}"
String Concatenation
Let us now append two Strings:
val initialName = "Stephan"
val lastName = "Charko"
val appendedName = "$initialName + $lastName"
So, there is no need for a separate function and making extra variables or calling in-built functions for simple String operations.
Classes in Kotlin
This can be best demonstrated using an example. Let us start by defining a very simple POJO, a Car class in Java.
package com.hello;
public class Car {
private String brandName;
private String modelName;
private long enginePower;
private long price;
public String getBrandName() {
return brandName;
}
public void setBrandName(String brandName) {
this.brandName = brandName;
}
public String getModelName() {
return modelName;
}
public void setModelName(String modelName) {
this.modelName = modelName;
}
public long getEnginePower() {
return enginePower;
}
public void setEnginePower(long enginePower) {
this.enginePower = enginePower;
}
public long getPrice() {
return price;
}
public void setPrice(long price) {
this.price = price;
}
}
Now, of course this looks simple enough. Wait till you see the Kotlin version. Let’s define that next:
package com.hello
class Car {
var brandName: String? = null
var modelName: String? = null
var enginePower: Long = 0
var price: Long = 0
}
We have some points we should take note of here:
- There are no semicolons in Kotlin
- No getters and setters in Kotlin are needed as they are auto-generated for all fields
- Also, equals, hashCode and toString methods are also auto-generated in Kotlin
Performance : Java vs Kotlin
Now, the most basic question which arises when comparing two languages is which one is faster?
This question is usually naive because the speed of a program is actually determined by the style instead of a language. Now, if we really need to compare these two languages, let us first compile some facts about them:
- There is no difference in the bytecode generated by either Kotlin or Java.
- Kotlin doesn’t add any more semantics over Java and has no added meta-programming.
With above facts in mind, we can freely say that the performance of Kotlin is virtually exactly the same as Java as the generated bytecode is exactly the same. Still, Kotlin does provide us very clean programming style and constructs.
Hope you enjoyed this intro to Kotlin. Check back for more Kotlin articles and be sure to stop by the homepage to search and compare SDKs, APIs, code libraries and other development tools.
Recent Stories
Top DiscoverSDK Experts
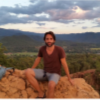
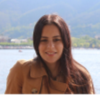
Compare Products
Select up to three two products to compare by clicking on the compare icon () of each product.
{{compareToolModel.Error}}
{{CommentsModel.TotalCount}} Comments
Your Comment