Introduction to Backend Web Development With Node.js
The most used and most popular programming language on the web is JavaScript. Once it was just a language that could be run on web browsers alone. Companies had to hire JavaScript developers to make the frontend interactive, and backend developers to make the backend dynamic. Backend developers had various options of choosing different programming languages, frameworks, and other tools. One could use PHP, Java, Python, Perl, etc. as the programming language. But in 2009 the landscape changed. Node.js appeared as a backend JavaScript engine for writing backend code in pure JavaScript. It enabled frontend programmers to make use of their JavaScript skills and apply them to the backend, while enabling companies and businesses to hire a single programmer to work on both sides of the web. That is just a very small part of the whole story.
In the previous series of articles I was teaching you Angular 5 by developing a to-do application. Did you notice that all the tasks we added were lost when we refreshed the page or when we restarted the application from the command line? This happened because we did not use any persistent layer. Literally that means that we did not send the data to a database through a backend server and then retrieve it on the application restart. So, I thought that the most practical way to proceed teaching you developing web applications is to start teaching things when they start to block our way of learning. To fulfill our knowledge of Angular we will need to know about the reactive extension of JavaScript (RxJs), making internet requests (including ajax and WebSockets), etc. But the most important thing to learn now is how to code for a backend application so that we can save our data. Don't think that the knowledge earned in this series will only be applicable to Angular 5—this series on Node.js is independent of anything else, including Angular.
I have setup a Github repository for you to keep the code in this series of articles. The code will be available in the articles themselves and you will be able to find them as complete projects in the Github repository. If you have questions, you can ask them by creating issues on Github. Go to Learn Node.js With Sabuj to browse the code. Code for each repository is placed in different branches. Browse to specific branches for the code of a specific articles. For example, the link to the branch for this article is Introduction to Backend Web Development With Node.js
I was teaching vanilla JavaScript using Node.js for quite some time on this blog. So, I think you are already familiar with how to install Node.js, write scripts and execute them. Let's start by creating a simple server application in Node.js. Create or choose a directory where you want to keep your JavaScript files. Create a file called 01_first_server.js. Cd into that directory from the command line. Run node 01_first_server.js from the command line to check that everything is alright.
For creating a server, we will need to import (or 'require' in Node.js's dialect) the 'http' module. In Node.js different responsibilities that are not core to the language are divided into modules. To use those modules we need to import them into the script we want to use them from. In Node.js we need require() to import them. Inside require() we need to pass the name of the module as a string. We will also need to save the return value from require into a variable so that we can use it throughout the script. Let's get to code:
var http = require('http');
You could save the module into a variable named anything other than http, but we are keeping it the same to make things more transparent.
The http module has a function called createServer() that will create a Server object. The createServer() function takes a request listener callback function. This callback function is responsible for accepting requests from user agents and returning a response. This callback function accepts two parameters where the first one is the request object and the second is the response object. Let's talk in code:
http.createServer(function (request, response) {
// TODO: write request processing code here.
});
The Server object has a method called listen() that is responsible for starting the process of accepting requests and serving them with the request listener callback function. We can specify host and port through this method, but here we will leave the default to localhost and the port to 5000.
http.createServer(function (request, response) {
// TODO: write request processing code here.
}).listen(5000);
We still have the request processing code unwritten. To get any request and user agent related information we can use the request object that is being passed through the request parameter. But that is not a concern for us in this article. We want to send a response to the user agent (browser in this case) no matter what URL they use or whatever request method they request with. To inform the user agent that everything is alright we need to send it a 200 response code. And to tell it that the response type is an HTML document we need to send the Content-Type header. To send the response code and to send the header we need to use the writeHead() method on the response object. The first parameter will be an integer indicating the response code and the second parameter will be an object containing all the headers.
http.createServer(function (request, response) {
response.writeHead(200, {'Content-Type': 'text/html'});
}).listen(5000);
To make some more breathing room, let's refactor like below:
http.createServer(
function (request, response) {
response.writeHead(200,
{
'Content-Type': 'text/html'
}
);
})
.listen(5000);
We haven't sent any data yet. We can send some data and end the request with the end() method on the response object by passing it a string like below:
http.createServer(
function (request, response) {
response.writeHead(200,
{
'Content-Type': 'text/html'
}
);
response.end("<h1>This is a sample response</h1>");
})
.listen(5000);
So, the complete code becomes:
var http = require('http');
http.createServer(
function (request, response) {
response.writeHead(200,
{
'Content-Type': 'text/html'
}
);
response.end("<h1>This is a sample response</h1>");
})
.listen(5000);
Now, fire up your terminal and execute the script. Go to the browser and browse to http://localhost:5000. You will see a bold and dark message with text: This is a sample response
Too keep things concise I haven't written complete HTML in the string. Try yourself and have fun with new knowledge of creating backend web applications with Node.js. See you in the next article.
About the Author
My name is Md. Sabuj Sarker. I am a Software Engineer, Trainer and Writer. I have over 10 years of experience in software development, web design and development, training, writing and some other cool stuff including few years of experience in mobile application development. I am also an open source contributor. Visit my github repository with username SabujXi.
Recent Stories
Top DiscoverSDK Experts
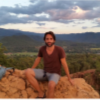
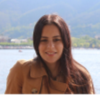
Compare Products
Select up to three two products to compare by clicking on the compare icon () of each product.
{{compareToolModel.Error}}
{{CommentsModel.TotalCount}} Comments
Your Comment