Backend Development in Node.js - Reading and Serving Files
I hope you enjoyed Node.js backend programming in the previous article. Creating a server didn’t require multiple complex software installations and configurations—it was just a few lines of JavaScript code. Anyone who has basic knowledge of JavaScript programming can easily become a backend web developer by learning about Node.js architecture and its modules. The script for the previous article was uploaded to the Github repository titled Learn Node.js With Sabuj. As I told you, code for each article in this series of articles will be available on separate branches on that repository. The complete code for this article will be available on the branch named 002_reading_serving_files.
For this article create or choose a directory where you want to put the JavaScript files. Or, you can clone the repository from Github. Create a file named 02_reading_n_serving_files.js. Run it with node 02_reading_n_serving_files.js to make sure everything is alright.
In the previous article, we wrote a very minimum amount of HTML as the response in the string. You were advised to write the complete HTML code. If you tried then I hope you found it very irritating. You may have already thought of alternatives, like loading the HTML string from files. In this article, we are going to solve that tiresome job of writing HTML inside strings. If you write HTML code inside files instead of strings you get many advantages along with HTML syntax highlighting and auto-completion from IDEs.
To load HTML as a string from the file system we need to read the file contents. If you have previous experience in writing JavaScript for browsers, you might already know that we cannot access file systems from the browser for security issues. But in Node.js you have complete freedom to access the file system just like in other popular programming languages. To access a file system from Node.js you need the fs module (fs stands for ‘file system’). In this article, we don't have any intention of using all the features of the file system with the fs module. We just want to read a file and get the contents as a string.
Let's import the fs module and store it in the variable named fs.
var fs = require('fs');
The fs module has a function called readFile(). This is an asynchronous function. So, to get the result as a string after the reading is complete we will need to provide it with a callback function. As the first parameter to the function, we will need to provide is the file name, and as the second parameter pass the callback function. The callback function will accept two parameters, the first parameter is used for errors that will report about any error during file reading process, and the second parameter is to provide the read data from the file.
Before we can read a file, it must exist in the file system. We do not want to read random files for our project. We want to read an HTML file that we will use to send a response to the user agent. Let's create a file called home.html in our chosen directory. Put the following contents in the HTML file.
<!doctype html>
<html>
<head>
<title>Learning Node.js Part 2</title>
</head>
<body>
<h1>My Header for Node.js Learning</h1>
<p>This is my paragraph that you will surely not appreciate.</p>
</body>
</html>
Let's get back to JavaScript code to read the file contents.
var fs = require('fs');
fs.readFile('home.html', function (error, file_data){
// do some processing here.
});
Reading is not our ultimate goal. Our ultimate goal is to serve it to the user agents. Again, this function is asynchronous. So, we will need to wrap the header and data sending code inside the callback function. So, we will get:
fs.readFile('home.html', function (error, file_data){
response.writeHead(200,
{
'Content-Type': 'text/html'
}
);
response.end(file_data);
});
So, our simple http server code will look like below:
var http = require('http');
var fs = require('fs');
http.createServer(
function (request, response) {
fs.readFile('home.html', function (error, file_data){
response.writeHead(200,
{
'Content-Type': 'text/html'
}
);
response.end(file_data);
});
})
.listen(5000);
Fire up the command line, run the script, go to the browser and see the result.
Now delete the home.html file to see what happens. The browser is empty. No 404, no 500, nothing. This happened because we did not handle the error properly. We did not check whether there are any errors or other types of system failure. In this case, the file was not found. So, let's play with some if-else blocks. Let's just check the error and send an error report to the browser by saying Something Went Terribly Wrong.
var http = require('http');
var fs = require('fs');
http.createServer(
function (request, response) {
fs.readFile('home.html', function (error, file_data){
if (error){
response.writeHead(500,
{
'Content-Type': 'text/html'
}
);
response.end("<b>Something Went Terribly Wrong</b>");
}else{
response.writeHead(200,
{
'Content-Type': 'text/html'
}
);
response.end(file_data);
}
});
})
.listen(5000);
Restart the script and rename or delete home.html to see that terrible message.
To serve binary files, our approach will be a bit different. We will learn about them later when we get more mature in our backend web development journey with Node.js.
I hope you have enjoyed the journey of learning backend web development with Node.js so far. You will need to write all the code by your own hand. If you want all the code in a cohesive fashion, you can go to my github repository. Now, keep practicing with your own ideas and wait for the next article in this series.
About the Author
My name is Md. Sabuj Sarker. I am a Software Engineer, Trainer and Writer. I have over 10 years of experience in software development, web design and development, training, writing and some other cool stuff including few years of experience in mobile application development. I am also an open source contributor. Visit my github repository with username SabujXi.
Recent Stories
Top DiscoverSDK Experts
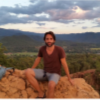
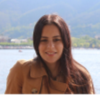
Compare Products
Select up to three two products to compare by clicking on the compare icon () of each product.
{{compareToolModel.Error}}
{{CommentsModel.TotalCount}} Comments
Your Comment