Generics in Kotlin
As you probably know, Kotlin and Java have their similarities. One way in which this is true is how they allow for a type or method to operate on objects of various types. This is known as Generics. So in this article, we’ll take a quick look at how to implement generics in Kotlin and how developers can use the functionality found in the generics library.
Generics in Kotlin are implemented in a similar vein to those of Java. However, in Kotlin there are two new keywords: out and in. This makes the code more readable.
Classes and types are two completely different concepts in Kotlin. Here we’ll give you an example where List is a class and List<String> is a type. This example shows how generics are implemented in Kotlin.
fun main(args: Array<String>) {
val integer: Int = 5
val number: Number = integer
print(number)
}
In this example, we declared an integer and then assigned the variable to a number. We can do this because Int is a subclass of the Number class. This is important to note because the type conversion will occur automatically at runtime and give us our output of 5.
Here’s a great generics tip in Kotlin: It’s better to use a generic data type is you’re not sure about the data type that you’re going to use in the app.
In Kotlin, generics are usually defined by <T> with the T meaning template, which can be dynamically determined by the Kotlin compiler. Now, here’s an example where we’ll use generic data types:
fun main(args: Array<String>) {
var object = genericsExample<String>("JAVA")
var objetc1 = genericsExample<Int>(88)
}
class genericsExample<T>(input:T) {
init {
println("I'm being called with a value of "+input)
}
}
In this Kotlin code example, we create a class with generic return type, represented by <T>. Now have a look at the main method. Notice that we have defined its value dynamically at runtime by giving the value type when creating the object of this class. That’s how generics are interpreted by the Kotlin compiler. This code example gives us the following output:
I'm being called with a value of JAVA
I'm being called with a value of 88
If we want to assign a generic type to one of its supertypes, all we need to do is “out” keyword. The same holds true for sub-types, but instead we use the keyword “in.” We’ll look at an example using “out” but you can also try for yourself and use “in.”
fun main(args: Array<String>) {
var objet1 = genericsExample<Int>(10)
var object2 = genericsExample<Double>(10.00)
println(objet1)
println(object2)
}
class genericsExample<out T>(input:T) {
init {
println("I am getting called with the value "+input)
}
}
This block of code will return the following output:
I'm being called with a value of 22
I'm being called with a value of 22.0
genericsExample@28a418fc
genericsExample@5305068a
That's all for this time. Check back again soon for the next article in our Kotlin series.
Previous Article: Data & Sealed Classes | Next Article: Delegation in Kotlin |
And remember to stop by the homepage to search for all the SDK, libraries, and code tools you'll need.
Recent Stories
Top DiscoverSDK Experts
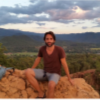
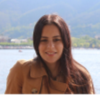
Compare Products
Select up to three two products to compare by clicking on the compare icon () of each product.
{{compareToolModel.Error}}
{{CommentsModel.TotalCount}} Comments
Your Comment