Data Classes & Sealed Classes in Kotlin
Welcome back to our Kotlin series. In this Kotlin article, we’ll talk about data and sealed classes and give you some examples. So let’s dive right into Kotlin data classes.
Kotlin Data Classes
At some point in your program, the need will almost certainly arise for a class whose primary purpose is to hold data. This will actually happen quite frequently, so we need a good way to handle this. Kotlin allows us to do this by marking as class with data. A Kotlin data class does not provide any other functionality other than holding the data.
All classes marked as data classes in Kotlin must have one primary constructor and each primary constructor should contain a minimum of one parameter. Data classes in Kotlin have some nice built-in functions such as toString(), hashCode(), and others. But Kotlin data classes may not have modifiers such as abstract and open or internal. Further, you may extend data classes to another class.
Let’s look at an example where we’ll create a data class:
fun main(args: Array<String>) {
val article: Article = Article("Kotlin Data Classes", "DiscoverSDK.com", 5)
println("Article Name: "+article.name) // "Kotlin Data Classes"
println("Published by: "+article.publisher) // "DiscoverSDK.com"
println("Article review score: "+article.reviewScore) // 5
article.reviewScore = 7
println("All info printed together: "+article.toString())
//using inbuilt function of the data class
println("hashCode function example: "+article.hashCode())
}
data class Article(val name: String, val publisher: String, var reviewScore: Int)
Output:
Article Name: Kotlin Data Classes
Published by: DiscoverSDK.com
Article review score: 5
All info printed together: Article(name=Kotlin Data Classes, publisher=DiscoverSDK.com, reviewScore=7)
hashCode function example: -669621870
In this Kotlin code example, we’ve created a single data class that will hold some of our data. We accessed all of its data members from the main function. Now let’s turn to our second topic, sealed classes.
Kotlin Sealed Classes
Sealed classes are used in Kotlin to represent a restricted class hierarchy. Using sealed classes lets programmers maintain the data type of a predefined type. In order to make a sealed class, all you need to do is use the sealed keyword as a modifier of the class.
Sealed classes in Kotlin may get their own subclasses. Note however that any subclass must be declared inside of the same Kotlin file along with the sealed class.
Now let’s have a quick example of a Kotlin sealed class:
sealed class MyExample {
class OP1 : MyExample() // the class MyExmaple can only be of two types
class OP2 : MyExample()
}
fun main(args: Array<String>) {
val obj: MyExample = MyExample.OP2()
val output = when (obj) { // we define the class's object according to the input
is MyExample.OP1 -> "You chose option #1"
is MyExample.OP2 -> "You chose option #2"
}
print
Output:
You chose option #2
In this example, there is one sealed class called MyExample, which can only be one of two types: OP1 and OP2. In the main class, we create an object in the class and then assign its type at runtime. Note that, since our MyExample class is sealed, we can apply a when clause at runtime to implement the final output.
In sealed classes in Kotlin, there’s no need for any unnecessary else statement that will just make the code more complex.
That’s all for data classes and sealed classes in Kotlin. Join us next time for a quick look at Generics in Kotlin.
Previous Article: Extension Functions | Next Article: Generics in Kotlin |
And remember to stop by the homepage to search for all the SDK, libraries, and code tools you'll need.
Recent Stories
Top DiscoverSDK Experts
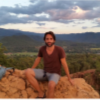
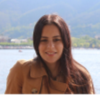
Compare Products
Select up to three two products to compare by clicking on the compare icon () of each product.
{{compareToolModel.Error}}
{{CommentsModel.TotalCount}} Comments
Your Comment