Constructors & Inheritance in Kotlin
In our last Kotlin article, we looked at class and object. Today, we’ll first talk about constructors and then turn to the subject of inheritance.
There are two types of constructors in Kotlin: the primary and the secondary. A Kotlin class can have one primary constructor and several secondary constructors. However, as opposed to Java where a constructor initializes the member variable, the primary constructor in Kotlin initializes the class while the secondary constructor will contain some additional logic and also initialize the class. The primary constructor is declared at the level of the class header. Let’s demonstrate this in an example:
class Person(val firstName: String, var age: Int) {
// class body
}
In the example above, we first declare the primary constructor inside the parentheses. There are two fields: firstName which is read-only and declared as a val and age which can be edited. Here’s an example in which we’ll use the primary constructor:
fun main(args: Array<String>) {
val person1 = Person("DiscoverSDK.com", 25)
println("First Name = ${person1.firstName}")
println("Age = ${person1.age}")
}
class Person(val firstName: String, var age: Int) {
}
This code block will automatically initialize the two variables. Here is the output it will return:
First Name = DiscoverSDK.com
Age = 25
As we said above, Kotlin lets you create one or more secondary constructors for your class. This is done by using the constructor keyword. It’s required anytime you want to create more than one constructor or when you want to include extra logic in the primary constructor but you can’t because it may have been called by another class. In the example below, we’ve created a secondary constructor and are using the example above to implement it.
fun main(args: Array<String>) {
val HUman = HUman("DiscoverSDK.com", 25)
print("${HUman.message}"+"${HUman.firstName}"+
"Here is the Secondary constructor example -- Your Age is: ${HUman.age}")
}
class HUman(val firstName: String, var age: Int) {
val message:String = "Hello!"
constructor(name : String , age :Int ,message :String):this(name,age) {
}
}
You should take note that you can create as many secondary constructors as you like. However, any secondary constructor you create in Kotlin must call the primary constructor either directly or indirectly. The code above will return the following output:
Hello!DiscoverSDK.comHere is the Secondary constructor example -- Your Age is: 25
That’s about it for the basics of constructors. Now let’s turn our attention to the topic of inheritance. If you have experience in Java or another OOP language, you probably know that inheritance means a child class will take some of the properties of a parent class. In Kotlin, we call the base class ‘any’ which is the super class of the ‘any’ default class declared in Kotlin. Just like in many other OOP languages, Kotlin lets you use the colon operator to use inheritance.
In Kotlin, everything is final by default. As such, we need to use the keyword “open” before the class declaration to make inheritance possible for the class. Have a look at this example to see how inheritance works in Kotlin:
import java.util.Arrays
open class ABC {
fun think () {
print("Here is the Kotlin inheritance example ")
}
}
class BCD: ABC(){ // inheritance occurred using the default constructor
}
And here is what the code above will return:
Here is the Kotlin inheritance example
But what happens if we want to override the method think() that’s in the child class. Have a look at the next example in which we’ll create two classes and then override one of its functions into the child class:
import java.util.Arrays
open class ABC {
open fun think () {
print("Hey!! i am thinking ")
}
}
class BCD: ABC() { // inheritance happens using default constructor
override fun think() {
print("I Am from Child")
}
}
fun main(args: Array<String>) {
var a = BCD()
a.think()
}
The code above calls the method inherited into the child class and will give back the results below.
I come from the child class
Note that, just as in Java, Kotlin does not allow for multiple inheritance.
Previous Article: Control Flow in Kotlin | Next Article: Visibility Modifiers |
Recent Stories
Top DiscoverSDK Experts
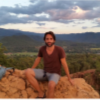
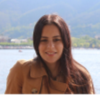
Compare Products
Select up to three two products to compare by clicking on the compare icon () of each product.
{{compareToolModel.Error}}
{{CommentsModel.TotalCount}} Comments
Your Comment