Visibility Modifiers in Kotlin
Welcome back to our series on Kotlin! In our last article, we looked at class & object in Kotlin and today we’ll cover the various types of visibility modifiers that are available to you in the Kotlin programming language.
What Are Visibility Modifiers in Kotlin?
In Kotlin, visibility modifiers are keywords that set the accessibility, or visibility, of classes, objects, constructors, functions, properties, interfaces, and all of their respective setters. Note we said setters and not getters. In Kotlin, you can’t set the visibility modifier of getters since they will always take that of the property. Kotlin has four types of visibility modifiers: private, protected, internal and public. Public is the default if no other visibility modifier is set.
Visibility modifiers are used to limit the usage of a variable, method or class being used in an application. And just like in other object-oriented languages they can be used in places like the class header or in a method declaration.
Now let’s take a closer look at all four types.
Private
When you declare something with a private modifier, it will then be accessible in its immediate scope. For example, a private package is accessible only in that particular file. A private class or a private interface is also only accessible by its data members and so on.
Let’s take a look at a quick example:
private class privateClassExample {
private val i = 1
private val doesSomething() {
}
}
In this example, the class “privateClassExample” and the variable ‘i’ can only be accessed in the same Kotlin file, where they are all declared as private in the declaration block.
Protected
The second visibility modifier we’ll look at is protected. The first thing to know here is that this modifier cannot be set on top-level declarations. Declarations that are protected in a class are accessible only in their subclasses, and when overridden would have the same protected modifier in the subclass unless explicitly changed.
So, a protected class, interface, etc., is only visible to its subclass. Here’s an example:
class A() {
protected val i = 1
}
class B : A() {
fun getValue() : Int {
return i
}
}
Here, the variable ‘i’ is declared as protected and is therefore only visible to its subclass.
Internal
The internal modifier is relatively new in Kotlin. When something is set as internal, then that specific field will be in an internal field. Internal packages are only visible inside the module under which they are implemented. For instance, an internal class is only visible to another class that is present inside the same module or package.
Here’s an example where we’ll look at the implementation of an internal method:
class internalExample {
internal val i = 1
internal fun doesSomething() {
}
}
In this example, both the doesSomething method and the variable ‘i’ are set as internal. As such in this case these two fields can only be accessed inside the package in which they were declared.
Public
Our last type of visibility modifier in Kotlin is public, which as we said earlier is the default. The public modifier can be accessed from anyplace inside of the project workspace. That means, so far in this series on Kotlin, since we never set the modifier to anything else, every code example has been in the public scope.
Here’s an example of what a public class would look like:
class publicExample {
val i = 1
fun doSomething() {
}
}
Well, it doesn’t look like anything special at all since it is of course public by default—no keyword is needed to make it public.
That’s all for this one. Check back soon for the next article in our Kotlin basics series where we’ll look at extensions.
Previous Article: Constructors &Inheritance | Next Article: Extension Functions |
Recent Stories
Top DiscoverSDK Experts
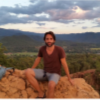
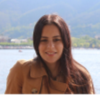
Compare Products
Select up to three two products to compare by clicking on the compare icon () of each product.
{{compareToolModel.Error}}
{{CommentsModel.TotalCount}} Comments
Your Comment