Automated Testing in React
A significant part of professional software development is writing automated testing. Why do we need them? Because automation tests are a big part of a process called ‘continuous integration’ which is the practice of integrating old code with new code.
The point of this article is not to convince you to write automated test—that would be an issue for a separate article. I’m just going to assume that you already know that automated tests are important or you’re willing to take my word for it. So in this article and in the following, I’ll explain how to write automated tests in React.
Testing in React works best with a framework & runner called Jest. Jest is based on Jasmine, which pretty much became the standard for JavaScript unit testing. Jest is a framework and runner, meaning that it also runs the tests. As opposed to other runners, it uses JSDOM and it’s very fast. In this article, we’ll learn to write basic tests and in the next one, we’ll take a look at debugging them.
The command create react app, which we learned about in a previous article, is built into Jest. As such we don’t need to set it (anyway, setting it is pretty simple). In that article, I explained how to put components in. The one I choose to put in is as simple as could be:
import React from 'react';
class MyComponent extends React.Component {
render() {
return <p>Hello world!</p>
}
}
export default MyComponent;
Note that we’re talking about a component of ‘class.’ I could even create a component that does the same thing, but as a function:
import React from 'react';
const MyComponent = () => (
<p>Hello world!</p>
);
export default MyComponent;
Either way, we need to check it. So how do we write a testing file for it? First we make a file with the name of the component in the folder where we created the component, but with a .test.js or .spec.js ending. If we made a component called src/components/MyComponent/MyComponent.jsx, then we’ll create the testing file src/components/MyComponent/MyComponent.spec.js in the same folder.
This file will always contain two lines:
import React from 'react';
import ReactDOM from 'react-dom';
and then the actual test where we call MyComponent and check if it’s rendering and returning the result we want:
import React from 'react';
import ReactDOM from 'react-dom';
import MyComponent from './MyComponent';
it('renders without crashing', () => {
const div = document.createElement('div');
ReactDOM.render(<MyComponent />, div);
expect(div.innerHTML).toEqual('<p>Hello world!</p>');
});
You can see it’s pretty easy—nothing to be afraid of. All I’m doing here is creating an element in which I render my component. The final line is the one that is a little scary, especially if you never wrote tests before:
expect(div.innerHTML).toEqual('<p>Hello world!</p>');
What’s going on here? It’s exactly what it looks like. I’m expecting that the InnerHTML of the div will contain whatever the component produces. Easy, no? OK, but where did that toEqual come from? If you’re familiar with Jasmine you would know. And if you’re not? Just check out the Jest documentation that explains everything, including the toEqual.
So, how do we run the test? We simply use the command npm t in the console or CMD:
This is a very short little taste of testing in React with Jest just to quell your fears a bit. In the next article, we’ll get more serious and talk about things like testing components with a state, for example. Plus we’ll look at debugging which is a lot easier than you may think. But, if you’ve never had any experience with writing automated tests, give the examples here a try. See how fun and easy it really is!
Previous Article: Routing in React Applications | Next article: Testing React Components with Jest & Enzyme |
About the author: Ran Bar-Zik is an experienced web developer whose personal blog, Internet Israel, features articles and guides on Node.js, MongoDB, Git, SASS, jQuery, HTML 5, MySQL, and more. Translation of the original article by Aaron Raizen.
Recent Stories
Top DiscoverSDK Experts
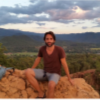
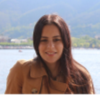
Compare Products
Select up to three two products to compare by clicking on the compare icon () of each product.
{{compareToolModel.Error}}
{{CommentsModel.TotalCount}} Comments
Your Comment