Routing in React Applications
In the last post, we talked about how to set up a real React environment and make a real React application. But a real React application is not really complete without routing. What’s this whole routing thing you ask? You know when you navigate your way to an internet site and the address changes? Well, that’s because the site is routing you—routing you from one address to another when the page loads. On Wordpress as well as on other “traditional” website, the page is loaded for each page. In React applications, however, we have routing that doesn’t require a page reload.
In contrast to other standard websites that we are familiar with, in React (and Angular BTW) you can choose whichever routing system you want. For educational purposes and to make examples for this article, I’ll use the popular React routing system call… (wait for it…) React Router. Guess you didn’t fall out of your chair, right? What’s good about this React routing system is that its simple. In order to practice with it, we’ll build an application that contains a home page and an about page.
To get started, we’ll create a React application using create react app. The command that follows is for installing the routing. And no, it’s not terribly complicated. All it is is a very simple node module. Go to the command line and run:
npm install react-router-dom --save
The save is designed to keep the react-router-dom inside of the package.json, which is our installation file. After we’ve done this, we can start using React Router. That’s right, it’s just that simple!
In order to start using React Routing, we need to surround our entire application or at least the part that will use the routing.
import React from 'react';
import { Switch, Route, Link } from 'react-router-dom';
import Home from './Home.jsx';
import About from './About.jsx';
class MainApp extends React.Component {
render() {
return <div>
<nav>
<ul>
<li><Link to='/'>Home</Link></li>
<li><Link to='/about'>About</Link></li>
</ul>
</nav>
<h1>This is my App</h1>
<main>
<Switch>
<Route exact path='/' component={Home} />
<Route path='/about' component={About} />
</Switch>
</main>
</div>
}
}
export default MainApp;
There are a few router components here. The first is the main in which all of the content changes according to the routing we choose. Inside the main there is the switch which has the routing components inside of it—one for each path which in turn have other components inside of them. These components will be loaded when the path changes. How do we change the path? Notice the link component that takes the the ‘to’ property.
You can see how this works in the demo that I made. It’s a little wacky, ‘cause the routes that I wrote aren’t really the best, but notice that it doesn’t really matter because there isn’t any page reload. I just wanted to take care of the routing—the application is very basic so that anyone who wants to can learn from it.
So now it’s time to build your own practice application and play around with it a bit so you can better understand the basics of routing. It’s pretty easy really, once you grasp the idea of the components.
Previous Article: Setting Up a React Environment | Next article: Automated Testing in React |
About the author: Ran Bar-Zik is an experienced web developer whose personal blog, Internet Israel, features articles and guides on Node.js, MongoDB, Git, SASS, jQuery, HTML 5, MySQL, and more. Translation of the original article by Aaron Raizen.
Recent Stories
Top DiscoverSDK Experts
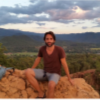
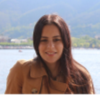
Compare Products
Select up to three two products to compare by clicking on the compare icon () of each product.
{{compareToolModel.Error}}
{{CommentsModel.TotalCount}} Comments
Your Comment