Working With Different Array Methods in Ruby
Arrays—being a very important data structure in Rub—need special attention. Arrays in Ruby have a whole bunch of method and in this guide we’ll will explore the most important ones.
Before You Begin
- Make sure that you have latest version of Ruby installed on your system.
- You should have basic knowledge of using command lines.
- A plain text editor or code editor is needed for writing Ruby code. You can use IDEs if you want.
- You should have read the previous posts on Ruby and practiced the examples properly.
Preparing Environment
- You should choose or create a directory where you want to put your Ruby files.
- Open the command line and change the current working directory to this one.
- Create a file called arrmeths.rb in this directory.
- Run the Ruby file with command ruby arrmeths.rb to check if everything is alright.
first and last
These two methods are used to get the first and the last elements of arrays. Let's try with an example:
arr = ["first element", 2, 3, 4, "x", "last element"]
puts arr.last
puts arr.first
Outputs:
last element
first element
fetch
When we access some element of an array that does not exist we get nil as the return value. But that may cause a bug in our code. Instead, we want to get some exception by accessing invalid index, we can use fetch.
arr = ["first element", 2, 3, 4, "x", "last element"]
puts arr.fetch 99
Outputs:
arrmeths.rb:2:in `fetch': index 99 outside of array bounds: -6...6 (IndexError)
from arrmeths.rb:2:in `<main>'
Again, if you want to get some predefined value if your code accesses invalid index you can specify that default predefined value as the second parameter:
arr = ["first element", 2, 3, 4, "x", "last element"]
puts arr.fetch 99, "I am here because you specified an invalid value"
Outputs:
I am here because you specified an invalid value
sample
Sample method is used to get one or more random entries from array. In games and such applications we often need random elements to place before users. Creating random numbers in the range of the maximum index and then getting those elements is not as easy as calling a simple method like sample. Let's clarify with an example:
arr = ["first element", 2, 3, 4, "x", "last element"]
puts arr.sample
puts arr.sample
puts arr.sample
puts arr.sample
puts arr.sample
puts arr.sample
Outputs:
2
3
4
last element
4
first element
If we want an array returned with random elements we can do this by passing an argument to the sample method. An array will be returned with that number of random elements:
arr = ["first element", 2, 3, 4, "x", "last element"]
print arr.sample 2
print arr.sample 3
print arr.sample 2
print arr.sample 4
print arr.sample 1
print arr.sample 2
Outputs:
[3, "x"]["last element", 4, 2][2, "last element"][3, "first element", "x", "last element"]["x"][2, "last element"]
pop, push
Adding and removing elements from array is a very common operation. We can add elements at the end, at the middle or at the end of an array. In case of removing we can do this at any position.
push is used to add or append element at the end of arrays.
arr = ["first element", 2, 3, 4, "x", "last element"]
arr.push 990
print arr
Outputs:
["first element", 2, 3, 4, "x", "last element", 990]
pop removes element from the end of an array. But, it does one additional thing along with that—it returns the removed element due to its invocation.
arr = ["first element", 2, 3, 4, "x", "last element"]
e = arr.pop
puts e
print arr
Outputs:
last element
["first element", 2, 3, 4, "x"]
shift, unshift
shift and unshift are cousins of push and pop. shift is used to remove an element at the beginning of an array and unshift is used to add element from the beginning of arrays. Like pop, shift also returns the removed element.
arr = ["first element", 2, 3, 4, "x", "last element"]
e = arr.shift
puts e
print arr
Outputs:
first element
[2, 3, 4, "x", "last element"]
sort, reverse
It is not a good thing to present randomly arranged data to users. We need to sort ordered collections often. For this purpose we can use the sort method on arrays:
arr = [5, 6, 1, 4, 0, 8, 10, 9]
arr2 = arr.sort
print arr2
Outputs:
[0, 1, 4, 5, 6, 8, 9, 10]
You can also pass a code block as a comparing code to sort the array according to your logic of sorting.
Reversing is also a common operation. Let's try be reversing the arr2 in our previous example.
arr = [5, 6, 1, 4, 0, 8, 10, 9]
arr2 = arr.sort
arr3 = arr2.reverse
print arr3
Outputs:
[10, 9, 8, 6, 5, 4, 1, 0]
uniq
In an order collection there may be repeated values. In our work we often need to duplicate values. In Ruby we can do this by a simple method. uniq is used to removed duplicates and return a an array with unique elements.
arr = [1, 1, 1, 1, 2, 2, 3, 4, 55, 5, 5, 6]
arru = arr.uniq
print arru
Outputs:
[1, 2, 3, 4, 55, 5, 6]
include?
include? is used to test whether an array contains an object or not. It return boolean true or false. It searches through the array and when it finds the element it does not go further and returns true. If it does not find match till the end, it returns false.
arr = [1, 1, 1, 1, 2, 2, 3, 4, 55, 5, 5, 6]
puts arr.include? 88
Outputs:
false
and More
I have not covered some methods in this post. I also have not covered some advanced usages of some methods covered here. Those uses need more advanced Ruby knowledge. I also skipped some unimportant methods here.
Conclusion
I will write another one or more guides in future to illustrate advanced usages of various array methods. At this stage of your learning, I recommend you to go over the examples again and again before moving on to more advanced topics.
Be sure to stop by the homepage to search and compare SDKs, Dev Tools, and Libraries.
Recent Stories
Top DiscoverSDK Experts
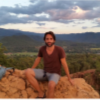
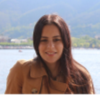
Compare Products
Select up to three two products to compare by clicking on the compare icon () of each product.
{{compareToolModel.Error}}
{{CommentsModel.TotalCount}} Comments
Your Comment