Using MongoDB with Node.js
In the last article we talked about using MongoDB with PHP, and today we’ll look at MongoDB with Node.js. Over the years, MongoDB has been more and more used together with Node.js. It’s not really a big surprise—both use JavaScript, and it’s quite easy to skip back and forth between them. Node.js also has a module language that takes care of and works with MongoDB. The popularity of the MEAN stack also contributed to this.
There are a number of Node.js modules that help with connecting to MongoDB, two of which are very popular. The first is node-mongodb-native which is Node.js’ natural driver for MongoDB. The second is Mongoose which sits on MongoDB Native and is a kind of object-relational mapping (ORM). In this article I’ll explain a bit about MongoDB Native—the examples will be based on the github for MongDB Native.
Before we start, we need to get the MongoDB server running. What is this server? Basically the service that allows other software to connect to MongoDB. This is just like if we use a server to get commands via a socket from other programs.
mongod --dbpath=/data --port 27017
This process needs to run if we want to work with MongoDB. Note that you need to have permission to access \data.
Another important note: if you’re working with MongoDB with PHP on the same machine as with Node.js, and you installed the MongoDB Driver via PEAR like in the last article, you won’t need to take this step since the server should already be working.
How do we check that everything is OK? Go to localhost:27017 in the browser and see if:
It looks like you are trying to access MongoDB over HTTP on the native driver port.
The next step is to install the module via npm. Here’s how you do it locally:
npm install mongodb
And now we can start working. Truth is, the actual work we’ll do here is pretty easy. Let’s say we want to connect to a database called test. We can set this in the initial connection.
var MongoClient = require('mongodb').MongoClient;
// Connect to the db
MongoClient.connect("mongodb://localhost:27017/test", function(err, db) {
//Async function
});
There’s nothing here that should give you any trouble to understand. If you’re connecting to a remote server, you put the parameters in connect. If it’s the same machine where the code is running then put it in localhoast. This is just an asynchronous function where the CRUD will run. If you try to do something before the database is ready… well I think you know how that’s going to end.
After we pick the database, we need to pick which collection we’re going to work with. This is pretty easy too:
var MongoClient = require('mongodb').MongoClient;
// Connect to the db
MongoClient.connect("mongodb://localhost:27017/test", function(err, db) {
if(err) { return console.dir(err); } //handling errors
var collection = db.collection('users'); //selecting the collection
});
Here I’m using the users collection. Simple and easy. From here we can start to execute commands. The find starts from the collection, just like in the console. If you went through the previous articles and worked on the material a bit, you’ll know that we need to use find. So let’s us it to find x:19 in our users (we practiced on this database in the last articles and there you can find instruction for creating it).
var MongoClient = require('mongodb').MongoClient;
// Connect to the db
MongoClient.connect("mongodb://localhost:27017/test", function(err, db) {
if(err) { return console.dir(err); } //handling errors
var collection = db.collection('users'); //selecting the collection
collection.find({"x":19}).toArray(function(err, items) { //foreach
console.log(items);
});
});
In this case we’re printing what we receive inside an array and printing the result. It’s important to make sure that, if the x is a string, to put it in quotes!
And so how do we enter in data? With the insert method, we pass a JSON object:
var MongoClient = require('mongodb').MongoClient;
// Connect to the db
MongoClient.connect("mongodb://localhost:27017/test", function(err, db) {
if(err) { return console.dir(err); } //handling errors
var collection = db.collection('users'); //selecting the collection
var doc = {"x" : 999, "name" : "Ran" }; //valid JSON object
collection.insert(doc,function(err, result) {
if(err) throw Error;
console.log(result); //we are getting back the object inserted
});
collection.find({"x":999}).toArray(function(err, items) { //foreach
console.log(items);
});
});
And to update? Pretty much the same way:
var MongoClient = require('mongodb').MongoClient;
// Connect to the db
MongoClient.connect("mongodb://localhost:27017/test", function(err, db) {
if(err) { return console.dir(err); } //handling errors
var collection = db.collection('users'); //selecting the collection
collection.update({"x":999}, {$set:{"name":"moshe"}}, function(err, result) {
console.log(result); //return true in case of success
});
});
And updating more than one value? Where we need to pass {multi: true}?
var MongoClient = require('mongodb').MongoClient;
// Connect to the db
MongoClient.connect("mongodb://localhost:27017/test", function(err, db) {
if(err) { return console.dir(err); } //handling errors
var collection = db.collection('users'); //selecting the collection
collection.update({"x":999}, {$set:{"name":"moshe"}}, <strong>{multi: true}</strong>, function(err, result) {
console.log(result); //return true if success
});
});
In principle, this is how we pass additional objects—with insert or find.
var MongoClient = require('mongodb').MongoClient;
// Connect to the db
MongoClient.connect("mongodb://localhost:27017/test", function(err, db) {
if(err) { return console.dir(err); } //handling errors
var collection = db.collection('users'); //selecting the collection
collection.remove({"x":999}, function(err, result) {
console.log(result); //number of deleted items
});
});
It’s not an issue of luck here, especially when it comes to basic operations, and those with a good command of MongoDB will be more than fine with the basics operations. And if we need more than the basics? There is documentation with explanations on all the additional operations out there.
If you’re still with me at this point and have followed the other guides closely, you’ll have a good command of basic MongoDB—to execute CRUD, work with binary files, model collections, make indexes, and import and export. Which is not bad. The next articles will be dedicated to more advanced topics.
Next up, MongoDb: Aggregations.
About the author: Ran Bar-Zik is an experienced web developer whose personal blog, Internet Israel, features articles and guides on Node.js, MongoDB, Git, SASS, jQuery, HTML 5, MySQL, and more. Translation of the original article by Aaron Raizen.
Recent Stories
Top DiscoverSDK Experts
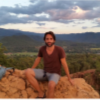
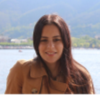
Compare Products
Select up to three two products to compare by clicking on the compare icon () of each product.
{{compareToolModel.Error}}
{{CommentsModel.TotalCount}} Comments
Your Comment