Understanding Strings in Ruby
A string is a sequence of characters. Unlike many other popular and modern programming languages, strings in Ruby are mutable. This means you can modify a string object.
Strings are everywhere in programming and it's almost impossible to write a program without strings. A sting is a human friendly data structure. To communicate with users from inside the program we use string to represent the information in human friendly manner.
Strings in Ruby support Unicode. So, applications developed with the help of Ruby support different human languages by birth. You can also encode and decode strings to and from different encodings.
Before You Begin
There are not many prerequisites before you begin working with Ruby strings. But, I recommend you read the first two posts on it before getting started with this third one. The first article discusses why you should and should not choose Ruby for your programming career. The second one covers installation, hello world, taking input and outputting to the command line. Make sure you have the following before you begin:
- Have Ruby installed on your system.
- Have a plain text editor or a code editor to edit Ruby code. You may also use an IDE, but that is not recommended for beginners.
- Have working knowledge of command line and know how to change the working directory and execute commands through the command line.
Getting Started
Create a file called ruby_string.rb in your preferred working directory. Point your command line or terminal emulator to this directory. When you need to run ruby_string.rb invoke the following command:
ruby ruby_string.rb
Creating a String
If you completed the tutorial on hello world in Ruby, you already know how to create strings in Ruby. Recall, you placed "Hello humans, this is my first ruby program." in front of the puts method. That was a string. So, you can create string by putting characters inside double quotes. But, that is not the end of the whole story. We will learn about different methods of creating and working with strings.
Ruby strings can be created literally and dynamically. Literal strings are the strings that you hard code in your Ruby source code and that is not a result of another code or method invocation. The characters we wrote inside double quotes is one kind of literal string out of a few. We wrote it manually. Literal strings can be created using single quotes too. So, we can rewrite the same string as 'Hello humans, this is my first ruby program.' or "Hello humans, this is my first ruby program."
Note:
Literal strings are no different than strings created in any other way. The difference is just in the creation process, the behavior is the same among all types of strings once they are created.
So, single quotes and double quotes are just delimiters for creating a string by putting characters inside of those delimiters. Ruby is a very flexible and fun to code in language. Unlike other languages, we are not limited to single and double quotes delimiters. We can define our own delimiters. Precede a delimiter with % to delimit a string. For example,
s = %=Hello, genius in front of me!=
puts s
Run it with ruby ruby_string.rb to get the following output:
Hello, genius in front of me!
You can also use parentheses, braces, and square brackets as delimiters as below:
s1 = %(String 1)
s2 = %(String 2)
s3 = %(String 3)
puts s1
puts s2
puts s3
Outputs:
String 1
String 2
String 3
There is another way of creating a string in Ruby, that is here doc. Here doc is a free style of creating literal strings. In this style you need not care about putting characters on the same line. You can press enter to put them on multiple lines and the newlines will be preserved. Here doc needs a << at the beginning followed by a delimiter string (just a pair of consecutive characters in this case). The string must end with the delimiter. Let's say that we want to use HDOC as a delimiter. For example,
hs = <<HDOC
Hi, I am an entity called string.
I have no obligation of being written on the same line.
I am also not obliged to put special characters in some creepy manner.
And now I end.
HDOC
puts hs
Outputs:
Hi, I am an entity called string.
I have no obligation of being written on the same line.
I am also not obliged to put special characters in some creepy manner.
And now I end.
Strings can be generated in runtime. They can be returned from another method invocation or as a result of a block of code. A new string can be created by joining two or more other strings. Other types of Ruby objects, when a representation in string is needed, can be serialized or converted to strings. For example, if we invoke to_s on a number, we get a string:
s = 1001.to_s
puts s
Outputs:
1001
We will see demonstrations of different ways of getting or creating strings dynamically, instead of creating them as literal, in our long journey through the land of the Ruby programming language.
Escaping Characters in Strings
Usually we write strings by delimiting characters with single or double quotes. But, what happens if we want to put a single quote inside a single quoted string and a double quote inside a double quoted string? Let's see with an example.
s = 'I can\'t do this'
puts s
Running this causes a disaster. Following is the output on the command line:
ruby_string.rb:1: syntax error, unexpected tIDENTIFIER, expecting end-of-input
s = 'I can't do this'
^
You cannot just put a single quote inside a single quoted string. You need to tell Ruby that the single quote you are putting there is not a delimiter, instead it is just a character that is a part of that string body. In Ruby we use backward slash \ as the escape character. Let's rewrite the example in the following manner:
s = 'I can\'t do this'
puts s
Run it and now it works as expected. In the same manner, if you want to put a double quote inside a double quoted string, you need to place a backward slash in front of that.
Now, if you want to put a newline inside a string, you cannot just press enter unless you are using a here doc. To put a newline, tab, carriage return, and some other control characters, you need a escape sequence. The escape character sequence for a newline is \n, for tab \t, for carriage return \r and so on. Try the following example:
s = "I am on the first line.\nI am the second line"
puts s
Outputs:
I am on the first line.
I am the second line
But try the same with single quotes:
s = 'I am on the first line.\nI am the second line\tI am at one tab distance'
puts s
Outputs:
I am on the first line.\nI am the second line\tI am at one tab distance
That means, special character sequences do not work with single quoted strings.
Conclusion
This article was to introduce you to strings in Ruby. In future articles I will cover different string operations on strings in Ruby and will familiarize you with different string methods.
Recent Stories
Top DiscoverSDK Experts
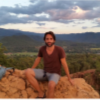
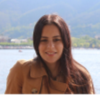
Compare Products
Select up to three two products to compare by clicking on the compare icon () of each product.
{{compareToolModel.Error}}
{{CommentsModel.TotalCount}} Comments
Your Comment