String Operations in Ruby with Operators
The String is one of the fundamental data structures without which almost no program can be written. So, it is very important to know about different string operations in any programming language. In the previous post I introduced you the string in Ruby. In this guide I would like to help you understand different string operations and how to work with them.
Before You Begin
This is a beginner’s guide so you don’t need to know or do much before you can move forward. But, check the following list before you begin:
- Make sure you have Ruby installed on your system.
- Make sure you have some level of familiarity with using command lines.
- Make sure you have a plain text editor or a code editor with Ruby support in your system. You can use IDEs if you like, but that is not recommended at this stage of learning.
- Make sure you have completed reading the previous posts on Ruby in this series and you have practiced all the code by yourself.
Preparing Your Environment
Choose a directory where you would like to keep your Ruby programs. Create a file called string_ops.rb in the directory. Start your command line and point the current working directory to the one where you have created string_ops.rb file. Run the Ruby file by invoking ruby string_ops.rb with the help of the command line to see if everything is alright. Open the file with a plain text editor or with a code editor to start coding.
String Concatenation
String concatenation is the process of joining two or more string together to form another string. In many modern popular languages string concatenation is quite painful or needs more than one line of code. In Ruby this operation is a breeze. You can concatenate two or more strings by putting a plus sign + between them. You can concatenate literal strings, string objects referenced by a variable, or a combination of them by placing a + sign between them. Look at the following example:
s = "I am the first string"
space = " "
result = s + space + "and I am the third part of the final string"
puts result
Run the string_ops.rb file by invoking ruby string_ops.rb command on the command line. You will see the following output:
I am the first string and I am the third part of the final string
String Interpolation
String interpolation is the process of inserting values of variables inside a string. To understand string interpolation better, let's try to create a string by concatenating different variables along with literal ones.
s1 = "Hello, World!"
name = "Md. Sabuj Sarker"
age = 28
result = s1 + "My name is " + name + ". My current age is " + age.to_s
puts result
The output you will get on the screen is:
Hello, World!My name is Md. Sabuj Sarker. My current age is 28
Note:
We converted number age to string by invoking to_s method on it.
It works well, but the solution above is not clean and it will really get out of control and look more dirty when we add more variables. It could be a lot better if we could put variable values inside the string in some way. The solution to such problems is string interpolation.
Let's see how it works with an example:
s1 = "Hello, World!"
name = "Md. Sabuj Sarker"
age = 28
result = "#{s1} My name is #{name}. My current age is #{age}"
puts result
Run it to see the same result:
Hello, World! My name is Md. Sabuj Sarker. My current age is 28
Look at the line: "#{s1} My name is #{name}. My current age is #{age}". This line did all the magic:
- We did not need to concatenate different variables and string values with the help of + operator.
- We did not need to worry about data conversion from other data types to strings.
- We could do the same thing in cleaner manner.
And we did all of these by putting variable names inside the curly braces of #{} inside the string. By placing variable names inside #{} Ruby will concatenate the parts of the string and convert data where needed.
String Replication
String replication is the process of replicating the same string a certain number of times and joining them together. It could be very hard and painful in many other languages, but in Ruby you can do this just by the multiplication operator * followed by a number indicating how many times you want to replicate the string. Let's say we want to create a string like below:
+++++++++++++++++++++
| I'm in the middle |
+++++++++++++++++++++
The + is repeated 21 times at the first line and another 21 times at the third line. But we do not want to write and count that many number of characters by hand. We can replicate + 21 times. Again, at runtime we want to create such boxes as above dynamically and we may need to calculate the number of + signs needed dynamically for it. Let's try to create the above string or box like string with an example:
s = "+"
multiplied_s = s * 21
middle_s = "| I'm in the middle |"
result = multiplied_s + "\n#{middle_s}\n" + multiplied_s
puts result
Run this to see the result:
+++++++++++++++++++++
| I'm in the middle |
+++++++++++++++++++++
Note the small portion of code: s * 21 - it just replicated + sign 21 times without writing any extra code.
More Operations
There are a lot more operations for strings in Ruby. Though the title of this post refers to string operation in Ruby, it mainly focuses on the operations that do not need any method to perform. The other operations will be demonstrated with the help of different string methods.
Conclusion
It is recommended that you go through this guide as many times as you need to better understand all of these beginner concepts. In future articles we’ll move forward and cover other string operations that need invocation of different string methods.
Check out the homepage for tons of SDKs, Libraries, and other Dev Tools.
Recent Stories
Top DiscoverSDK Experts
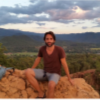
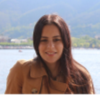
Compare Products
Select up to three two products to compare by clicking on the compare icon () of each product.
{{compareToolModel.Error}}
{{CommentsModel.TotalCount}} Comments
Your Comment