String Methods in Ruby
We can do a lot of things with strings in Ruby. In the previous guide I showed you different string operations with various operators in Ruby. In this post I am going to show you other important operations with the help of string methods.
Before You Begin
We have advanced quite far in our journey with Ruby programming and strings in Ruby. If you have read all the previous four posts on Ruby, you should be familiar with what you need to move forward. Here is a quick reminder of what you need:
- You should have Ruby installed on your system.
- You should be comfortable working with a command line.
- You should have a plain text editor or code editor in your system.
Preparing Working Environment
- Create or choose a directory where you want to put your Ruby files.
- Create a file called string_meths.rb in this directory.
- Point your command line's working directory to your chosen directory. You can do this with cd command or by opening the command line in the directory if your system provides some facility to that with context menu or file browser in use.
- Open the ruby file with the help of your plain text editor or the code editor of your choice.
- Run the file from the command line with the command: ruby string_meths.rb
String Length and Empty Strings
Often we need to know how many characters a string holds. We can find the length of a string by invoking the length method on it. Let's take input from a user and display how many characters that string contain.
puts "Enter some characters: "
i = gets
print "Characters you provided: #{i}"
print "The number of characters the user provided is: "
print i.length
It provides the following output:
Characters you provided: hello
The number of characters the user provided is: 6
Note:
The string hello consists of 5 characters, but we got 6 as result. When a user presses enter for gets method, a newline is appended at the end of the input string.
An empty string is the string that has no character inside of it. So, we can create an empty string by a couple of single or double quotes without any character inside of them. So, the length of an empty character is zero (0). Without checking the emptiness of a string with the length method, we can invoke empty? method on it—it will return a boolean, true or false, as a result.
empty_s = ''
print empty_s.empty?
Outputs:
true
Note:
You may be moved by seeing a question mark at the end of a method name. In Ruby we use question marks for methods that returns a boolean value.
Converting Cases
It is a very common operation to transform the cases of texts. We may need to transform strings to uppercase, lowercase, title case, etc. We have some convenient string methods for carrying out such tasks in Ruby.
To convert all the characters of a string to uppercase we need to use the upcase method on the string. It will transform all the characters to uppercase regardless in which case they are currently in.
s = "I am a miXed case string"
su = s.upcase
print su
Outputs:
I AM A MIXED CASE STRING
In the similar fashion we can transform all the characters of a string to lowercase by invoking downcase on string objects or values.
s = "I am a miXed case string"
sd = s.downcase
print sd
Outputs:
i am a mixed case string
We can also swap cases of characters in a string with the help of swapcase method in Ruby. This means that the characters that are in uppercase will be converted to lowercase and the characters in lowercase will be converted to uppercase.
s = "I am a miXed case string"
ss = s.swapcase
print ss
Outputs:
i AM A MIxED CASE STRING
Another common operation is capitalizing a string. In this case the first character of the first word in the string will be capitalized and all the rest will be transformed to lowercase.
s = "I am a miXed case string"
sc = s.capitalize
print sc
Outputs:
I am a mixed case string
String to Array of Characters
A string is nothing but a sequential collection characters. We may sometime need to get all those characters separated as an array of characters instead of a full string. To do this you need to invoke chars method on the string.
s = "I am a miXed case string"
print s.chars
Outputs:
Accessing Characters Individually or in Bulk
A string is like an array of characters. So, we can access characters of a string by their position. Or we can also get a substring of a string by referring to its start and end position. Positioning of characters inside a string starts from zero. We need to use the slice method to get an individual or a set of characters in a string.
s = "I am a cute string :*"
print s.slice(0) + "\n"
print s.slice(0, 4) + "\n"
print s.slice(5, 7) + "\n"
print s.slice(5..11) + "\n"
Outputs:
I
I am
a cute
a cute
Searching Through Strings
We can search through a string or test whether a string or character is present in another string. Let's start by checking whether a string contains the world lucky:
s = "I am fortunate"
puts s.include? "lucky"
puts s.include? "fortunate"
Outputs:
false
true
The second line of the code evaluates to false as the string does not contain the substring lucky but the third line evaluates to true as the string has fortunate inside of it.
We can also find the index of a character or substring inside a string:
s = "I am fortunate"
puts s.index("am")
Outputs:
2
We can also check whether some string starts with or ends with another substring.
s = "http://sabuj.me"
puts s.start_with? "http://"
puts s.end_with? ".com"
Outputs:
true
false
Replacing Strings
We often need to replace one string with another one. Ruby provides two very convenient methods for that. One is sub and the other is gsub. sub replaces the first occurrence and the gsub replaces all the occurrences. Both of the methods takes two parameters. The first one refers to the string/substring that we want to find and the second is the one that we want to place in place of the first one.
s = "You are a bad kid. Number of bad kids is increasing in our area."
puts s.sub "kid", "guy"
puts s.gsub "kid", "guy"
Outputs:
You are a bad guy. Number of bad kids is increasing in our area.
You are a bad guy. Number of bad guys is increasing in our area.
Notice that in the first line of the output the second occurrence of kid is not replaced with guy as we used sub there and in the second line both of the occurrences were replaced as we used gsub.
Conclusion
If you are just starting your career with programming and Ruby is your very first language, some of the examples may seem hard or somewhat incomprehensible at the first glance. Keep practicing since pacticing is the best way to overcome these difficulties. Check back soon for more articles on Ruby and keep practicing till you comprehend the way of programming in Ruby.
Check out the homepage for tons of SDKs, Libraries, and other Dev Tools.
Recent Stories
Top DiscoverSDK Experts
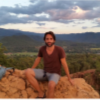
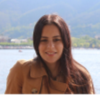
Compare Products
Select up to three two products to compare by clicking on the compare icon () of each product.
{{compareToolModel.Error}}
{{CommentsModel.TotalCount}} Comments
Your Comment