Optional Chaining in JavaScript ES2020
One of the most oft-requested and important features has finally made it into JavaScript in the new ES2020 standard. It’s called “optional chaining” and it’s super useful.
This feature helps solve the problem of script errors when we try to access a property or method that’s located deep within the object hierarchy. For example, something along these lines:
const myData = obj.prop1.prop2.prop3
That’s all well and good, but what if I receive an object that doesn’t contain prop1? I get a nasty error that kills my script. Try and run this code on your machine:
const myObject = {};
const myVar = myObject.prop1.prop2;
console.log('myVar', myVar); // Uncaught TypeError: Cannot read property 'prop2' of undefined
I don’t think there’s a single JavaScript programmer that hasn’t encountered this issue. It’s a pretty annoying error and it forces you to do something like this to get around it:
const myObject = {};
let myVar;
if (myObject.prop1) {
myVar = myObject.prop1.prop2;
}
console.log('myVar', myVar); // myVar undefined
Either that or to use a try/catch each time we try to access anything deep within the object hierarchy. It’s pretty frustrating because we often receive objects that we’re not really sure if they’re whole—either from the server or from somewhere else.
Luckily, just so we don’t have to deal with this issue, TC39 decided to give ES2020 the “optional chaining” feature. So how does it work? Couldn’t be easier—just a simple little question mark. If the property or method exists, we’ll receive it. If it doesn’t, we’ll get an undefined. And how does this look? Like so:
const myObject = {};
const myVar = myObject.prop1?.prop2;
console.log('myVar', myVar); // myVar undefined
This is just like the previous example but with a conditional statement. And, of course, I’m free to do this at any level of nesting that I want and on methods as well. Have a look:
const myObject = {};
const myVar = myObject.prop1?.prop2?.prop3?.prop4.?();
console.log('myVar', myVar); // myVar undefined
Now for the million-dollar question: Does it work in browsers? In a word … no. Neither Chrome nor Firefox supports it yet, but it does work just fine if you’re using Babel—just like all the best new JavaScript features. Still not up to date about Babel? Just check out my introductory article. You’ll see just how easy it is to make use of all the latest and greatest JavaScript features.
In the meantime, here’s a Codepen where you can see how this whole optional chaining thing works in JavaScript:
That’s all for this time. Check back soon for more great JavaScript ES2020 features :) See you then!
About the author: Ran Bar-Zik is an experienced web developer whose personal blog, Internet Israel, features articles and guides on Node.js, MongoDB, Git, SASS, jQuery, HTML 5, MySQL, and more. Translation of the original article by Aaron Raizen.
Recent Stories
Top DiscoverSDK Experts
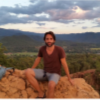
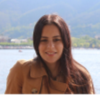
Compare Products
Select up to three two products to compare by clicking on the compare icon () of each product.
{{compareToolModel.Error}}
{{CommentsModel.TotalCount}} Comments
Your Comment