First Steps In JavaScript With Node.js
JavaScript is the language of the web. Without the help of any other programming language we can write code for both the frontend and for the backend with JavaScript. Whenever we need to bring some logic to the frontend of our website or web applications we use JavaScript and every browser supports it. To code for the server side in the past we had to use PHP, Perl, Java, Python, etc., but for many years now we’ve have Node.js to run JavaScript in the backend. But the web is not the only place where JavaScript is being used. It can be used for developing mobile applications, games, IoT application and even for developing Artificial Intelligence (AI), Machine Learning (ML), and Big Data applications. So, if you are late in the game then wait no more—now is the time to start learning JavaScript.
Before learning JavaScript (JS), many people think that it is somehow related to Java. JavaScript is neither Java, nor like Java, which is a totally different language. The names are similar for a some historical reason that we are not going to discuss in this article.
In this article I am going to use Node.js to run JavaScript. You may ask: why are you not using the browser to run JavaScript? The answer is simple: I want to teach you programming the way you should learn. Everyone does not learn JavaScript to code for browsers. Many people learn JS for doing scripting in Photoshop, or for developing web server backends; there are many different usages for it.
When we run JavaScript in web browsers, we end up using different APIs. But learning JavaScript is not about learning the different APIs of some system. It's about learning the language. When you master the language properly, you can use it for any system. Using Node.js we can go down the path of focusing on the language and not on different APIs. Again, if you have experience in a different programming language, it's most likely that you started learning your programming with command line interface. To make you comfortable in the strange world of JavaScript, using Node.js is the best way to go.
Getting Started
To get started you have to have Node.js installed on your system. You can get instructions for installing it on your system on the official website of Node.js. You can write your code in any plain text editor (GUI or command line based), and use any IDE that supports JavaScript or any other text editor. It's all up to you.
I am going to create a file named 'first.js', you can use any other name with '.js' extension at the end. Keep it empty. Make sure that your 'node' executable is available in your system path so that you can run the 'node' command through the command line.
Start your command line application in the path where you have created the .js file. Run the following command to see if everything is alright.
node first.js
Hello World Program With JavaScript
It's a common practice to write a hello world program when you first starting writing code in a programming language. Writing a Hello World program is very easy in JavaScript. To display text on command line we need to use the console.log() function. Edit your first.js file and put the following line of code:
console.log("Hello, World!");
Now, run the first.js file as before to see following output.
Hello, World!
Congrats! You have just completed writing your first program in JavaScript. Unlike many other programming languages, you do not need to write a lot of lines in JavaScript to carry out a simple task.
Remember: every statement in JavaScript ends with a semicolon as shown in the hello world program above.
Working With Variables
Unlike many other programming languages you do not need to declare type of a variable in JavaScript. But you have to put the keyword 'var' before every variable name. If you do not put the 'var' keyword then the variable will be a global variable. Polluting the global namespace is always frowned upon and it is a bad programming practice.
In JavaScript, to write a variable name, you can use characters from a-z, A-Z, 0-9, underscore _ and dollar sign $. No other characters are allowed for creating a variable name. Let's put a number in a variable called no.
Remember: a variable is like a container where you can put something. In JS or computer programming, a variable is a name by which we can point some memory location of the computer system and in which memory location we can keep some data.
var no = 6;
console.log(no);
Run the js file to see the following output:
6
Strings In JavaScript And How To Create Them
The string is one of the most important data types in JavaScript. To create a string in JS we have to surround some characters within quotation marks.
"This is a double quoted string"
You can create a string with single quotes.
'This is a single quoted string'
If we put these strings in variables then it looks like the following:
var str1 = "This is a double quoted string";
var str2 = 'This is a single quoted string';
Strings that are created by hand like this are called literal strings. There are lot of functions in JS that return strings as a result. So, this is not the only way in which you can create strings.
Numbers In JavaScript And How To Create Them
Numbers in JavaScript are like the numbers we are familiar with in our daily life. So, we can create numbers by literally putting them inside our source code.
100
We can create a decimal number by putting decimal point as a dot inside source code.
100.001
Unlike other programming languages JavaScript has only one type of number, which is a double. That means JS stores all the numbers in 8 bytes. When we write 100, JS keeps it as decimal number. But when we display a number that has no digit after the decimal point, JS will show us it like an integer. When dealing with numbers in JavaScript, you should keep this in mind.
Doing Some Math In JavaScript
When starting to learn a programming language you should learn how to do normal mathematical tasks in that language. In JavaScript you can use most of the ordinary mathematical signs.
Addition +
Let's add 1 and 1000 with the help of the addition operator in JS.
var i = 1;
var j = 1000;
var sum = i + j;
console.log("The sum of i and j is: " + sum);
Outputs:
The sum of i and j is: 1001
Note: Did you notice from the above program that we can not only add numbers but also we can add string with +.
Subtraction -
Subtraction is like addition. You just need to put the minus sign - between numbers. But, remember not to subtract strings or strings with numbers.
Multiplication *: On our normal keyboards, there is no key to input the multiplication sign. So, from the beginning of programming, programmers started to use * symbol instead. Though they could have used x instead, but what if you wanted to use x as a variable name?
Let's multiply two numbers.
var i = 4;
var j = 3;
var res = i * j;
console.log("The multiplication of i and j resulted in: " + res);
Outputs:
The multiplication of i and j resulted in: 12
Division /: Like multiplication, we do not have any luxury to use the division sign for dividing two numbers. So, programmers chose to use the forward slash /.
var i = 6;
var j = 3;
var res = i / j;
console.log(res);
Outputs:
2
And
var res = 5 / 2;
console.log(res);
Outputs:
2.5
Conclusion
Starting your career with JavaScript is a wise choice. It is so widespread everywhere that if you are good at it, you will not need to knock doors of the opportunities, instead opportunities will knock on your door. In this article I just covered some introductory topics. In future articles I will continue to teach you different aspects of JavaScript.
Need a good JavaScript framework? Check out 5 JavaScript Frameworks to learn in 2017.
*And be sure to stop by the homepage to search and compare SDKs, Dev Tools, and Libraries.
Recent Stories
Top DiscoverSDK Experts
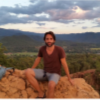
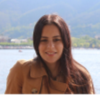
Compare Products
Select up to three two products to compare by clicking on the compare icon () of each product.
{{compareToolModel.Error}}
{{CommentsModel.TotalCount}} Comments
Your Comment