ECMAScript 6 - Symbol
Today we’ll be looking at Symbol in ECMAScript 6, and truth be told, it’s kind of a weird feature. First I’ll explain a little about it, then I’ll let you know what it’s good for. I’ve had some arguments with a few other programmers about Symbol. I feel its practical uses are limited, if they exist at all, but others feel that we may yet see them. What is certain though is that Symbol in ECMAScript 6 is pretty easy so it’s worthwhile to learn it.
In principal, Symbol is a primitive data type. Unique in that it doesn’t repeat on itself and can’t be changed. We create it with Symbol (without new, since it’s primitive).
var oneSymbol = Symbol();
var twoSymbol = Symbol();
console.log(oneSymbol === twoSymbol); //false
Whatever goes into oneSymbol is the Symbol. A completely unique value that’s stored in a reference.You can’t determine anything about this value other than the fact that it exists. If you put it into twoSymbol, it won’t be the same thing—they would be two completely different values.
For debugging purposes only, you can put a description in Symbol, but it will only be available in the console.
var oneSymbol = Symbol('this is a descriptive description');
console.log(oneSymbol); //Symbol(this is a descriptive description)
This is how it would look:
If we do Symbol typeof, we’ll see that it’s just a Symbol:
var oneSymbol = Symbol();
console.log(typeof oneSymbol); //"symbol"
So this brings us to the question of “what do we need this for?” This is a great question. When the Symbol is not global, its uses are limited—pretty much just when we need something unique (and sometimes we do). There are those that use Symbol to simulate a private variable in class. Here’s an example:
class Something {
constructor(){
var property = Symbol();
this[property] = 'test';
}
}
var instance = new Something();
console.log(instance[property]); //undefined, can only access with access to the Symbol
But in my opinion this is something not worthwhile to do with Symbol. Symbol’s power actually comes on the global side of the equation.
Symbol.for is global.
Now it starts to get interesting. Let’s have a quick look at the API.
As opposed to a regular Symbol, we can set a ‘key’ to Symbol, which makes a symbol specific to the key. Here’s an example:
var oneSymbol = Symbol.for('foo');
var secondSymbol = Symbol.for('foo');
console.log(oneSymbol === secondSymbol); //true
If we have a global Symbol, we can easily find it with the forKey method:
var oneSymbol = Symbol.for('foo');
console.log(Symbol.keyFor(oneSymbol)); //foo
Pretty cool, right? But what’s really great here is that the Symbol that gets created is the the same Symbol throughout different parts of the application. In other words, if some code creates a Symbol in an iframe for example, and different code makes a Symbol in a window, it will be the same Symbol! Check it out:
var frame = document.createElement('iframe')
document.body.appendChild(frame)
console.log(Symbol.for('foo') === frame.contentWindow.Symbol.for('foo'))
In this code I’m making an iframe and sticking it into my window. I make a Symbol in the iframe and also in the application, and notice that the result is true.
That’s pretty cool and also will probably come in handy if I want to share data between the iframe and the window. But personally? I would only use it if I need a unique indicator for something.
About the author: Ran Bar-Zik is an experienced web developer whose personal blog, Internet Israel, features articles and guides on Node.js, MongoDB, Git, SASS, jQuery, HTML 5, MySQL, and more. Translation of the original article by Aaron Raizen.
Recent Stories
Top DiscoverSDK Experts
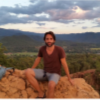
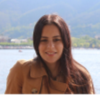
Compare Products
Select up to three two products to compare by clicking on the compare icon () of each product.
{{compareToolModel.Error}}
{{CommentsModel.TotalCount}} Comments
Your Comment