ECMAScript 6 - Lambda Expressions
In the last article we covered classes in ECMAScript 6 and I called the article Keepin’ it Classy , 'cause I’m a ‘dad jokes’ kind of guy. This time, we’ll be looking at Lambda Expressions (aka lambda functions or arrow functions), and despite being really really tempted, I’ve refrained from making any bad puns about the Lambada.
So what is a lambda expression? If we open up Wikipedia or a dusty old computer science book, we’ll learn that lambda expressions are a way of defining an anonymous function (meaning with no name), and they are especially useful when we want to define functions that return other functions.
OK, well that sounds about as intuitive quantum mechanics. The truth is, it’s pretty simple and straightforward—I’ll even demonstrate. Let’s say I want to put the sum of two numbers into a variable called a. We’ll say 1 and 2. With ECMAScript 5 I have to do this with a simple function like this one:
var sum = function (arg1, arg2) {
var result = arg1 + arg2;
console.log(result);
}
let a = sum(1,2); //3
In ECMAScript 6 I can do it with a lambda expression, something like this:
let sum = (arg1, arg2) => { console.log( arg1 + arg2) };
let a = sum(1,2); //3
Seems like something simple and perhaps a bit unnecessary, right? Sure, it could save a line or two, but what do we really need it for? Well, there are a few answers to that question. The first is that lambda expressions are very useful when building JavaScript objects. Building various types of JSON objects is a critical component of JavaScript applications, for anything above the ‘Hello World’ level. Imagine that we need to create an object that contains an id and a name. In ECMAScript 5 it will look like this:
var setObject = function (id, name) {
return {
id: id,
name: name
};
};
setObject (4, "Moshe"); //Object {id: 4, name: "Moshe"}
But if we use a lambda expression it will look like this:
var setObject = (id, name) => ({ id: id, name: name });
setObject (4, "Moshe"); //Object {id: 4, name: "Moshe"}
Looks better, doesn’t it?
Let continue and imagine that we have an array that contains a phones object, something like this:
var phones = [
{ name:'iphone', price:9999 },
{ name:'Android', price:200 },
{ name:'Nokia 4ever', price:1 }
];
We need to write a function that will returns only the telephone numbers—a common task in JavaScript, going over an object and take only one specific thing from it. How do we do this? We’ll use the map function (don’t be alarmed, it’s just a function that goes over each member of the array) and do something a little like:
var prices = phones.map(
function(phone) {
return phone.price;
}
);
console.log(prices); // [9999, 200, 1]
Let’s compare this to the lambda expression of ECMAScript 6.
var phones = [
{ name:'iphone', price:9999 },
{ name:'Android', price:200 },
{ name:'Nokia 4ever', price:1 }
];
var prices = phones.map(phone => phone.price);
console.log(prices); // [9999, 200, 1]
Now that really looks a lot better and is also more intuitive to understand. Just remember: wherever we find an anonymous function, we can use an arrow function. This is really useful for any map or filter functions that we use frequently. Instead of making a for each or a for loop, we can just use our lambda expression, which is pretty awesome.
Lambda expressions are also useful when it comes to callbacks or promise. Here’s an example that I didn’t write, but it’s really useful for understanding the concept:
// ES5
aAsync().then(function() {
returnbAsync();
}).then(function() {
returncAsync();
}).done(function() {
finish();
});
// ES6
aAsync().then(() => bAsync()).then(() => cAsync()).done(() => finish);
One of the most powerful aspects of the lambda expression is the lexical this. Everyone that codes JavaScript knows that this loses its meaning, and because of this we need use ridiculous things like this=that. Allow me to demonstrate:
function FooCtrl (FooService) {
var that = this;
that.foo = 'Hello';
FooService
.doSomething(function (response) {
that.foo = response;
});
}
As soon as we pass an anonymous function (very common with callbacks) inside another function (in this case we call doSometing inside of FooCtrl), the this loses it constant to that of the function that we called. Because of this we need to pass the variable this to a different source, in this case to that. With lambda expressions, the this remains this of the function that called it and we can do something like:
function FooCtrl (FooService) {
this.foo = 'Hello';
FooService
.doSomething((response) => { // woo, pretty
this.foo = response;
});
}
I admit, it can be a bit confusing. In general, wherever you see an anonymous function, it’s a sign that you can convert it to a lambda expression—particularly useful if it’s a map or filter function. If we need to create an object from another object or from zero, lambda expressions are an excellent solution. If we’re forced to use that as part of promise or a callback, we can definitely consider using a lambda expression.
Next article: coing soon! |
About the author: Ran Bar-Zik is an experienced web developer whose personal blog, Internet Israel, features articles and guides on Node.js, MongoDB, Git, SASS, jQuery, HTML 5, MySQL, and more. Translation of the original article by Aaron Raizen.
Recent Stories
Top DiscoverSDK Experts
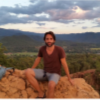
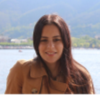
Compare Products
Select up to three two products to compare by clicking on the compare icon () of each product.
{{compareToolModel.Error}}
{{CommentsModel.TotalCount}} Comments
Your Comment