Class and Style Binding in Angular
In the previous article I discussed on property and attribute bindings. In this article I am going to discuss class binding and style binding. I hope by this stage of your learning you can already guess what we are going to do and how we are going to do. First of all let's level the ground by cleaning our code. In the last article we introduced tables and to show you attribute binding with colspan I added some code that is unnecessary for this article. We also want to use the list instead of table as we used before. So, our initial HTML angular template should look like this:
<h1>My profile</h1>
<b> Hello World </b> <br/>
My name is Md. {{ name }} <br/>
I am a {{ profession }}. <br/>
Do not forget to visit me at: {{ website }} <br/>
My Social Links:
<ol>
<li *ngFor="let link_obj of social_links" [cccclass]="link_obj.title">
<a *ngIf="link_obj.is_active; else elseTemplate" [href]="link_obj.link"> {{ link_obj.title }} </a>
<ng-template #elseTemplate>
<s> This link is not active </s>
</ng-template>
</li>
</ol>
Also, you can fall back to the list we were using before. The app.component.ts should look like the same for now if you do not have other requirements or need to customize some part of it.
import { Component } from '@angular/core';
interface ISocialLink{
title: string;
link: string;
is_active: boolean;
}
@Component({
selector: 'app-root',
templateUrl: './app.component.html',
styleUrls: ['./app.component.css']
})
export class AppComponent {
name = "Md. Sabuj Sarker";
profession = "Software Engineer";
website = "http://sabuj.me";
social_links: ISocialLink[] = [
{ title: "Facebook", link: "https://facebook.com/SabujXiP", is_active: true },
{ title: "Twitter", link: "https://twitter.com/SabujXi", is_active: false },
{ title: "Github", link: "https://github.com/SabujXi", is_active: true }
]
constructor(){
}
}
Class Binding
We often uses classes for styling the elements with the class or classes. A class can be used on multiple elements unlike id which should only be present on a single element in the entire document. To do some styling we need to write some CSS code. Where do we put it? We can put it in style.css that is present at the root of our application or we can use app.component.css—later I will write one or more detailed articles on styling showing the difference between component wise styling and global styling. For now, let's use app.component.css. We will create and use a few classes. white class will be used to make the text of an element white, yellowgreen will make texts yellow green. green_bg will be used to set the background color to green. red_border will be used to set a red colored border around elements. So, our app.component.css should look like this:
.white{
color: white;
}
.yellowgreen{
color: yellowgreen;
}
.green_bg{
background-color: green;
}
.red_border{
border: 1px solid red;
}
Now, how do we set classes? We can do that with pure HTML syntax as we always used to do. Let's try the simplest way.
<h1>My profile</h1>
<b class="white green_bg"> Hello World </b> <br/>
My name is Md. {{ name }} <br/>
I am a {{ profession }}. <br/>
Do not forget to visit me at: {{ website }} <br/>
My Social Links:
<ol>
<li *ngFor="let link_obj of social_links">
<a class="red_border" *ngIf="link_obj.is_active; else elseTemplate" [href]="link_obj.link"> {{ link_obj.title }} </a>
<ng-template #elseTemplate>
<s> This link is not active </s>
</ng-template>
</li>
</ol>
Go to the browser to see the changes. It's colorful now. But in this way we will not be able to make changes to the colors in the Angular way at runtime. We need property binding. We can use property binding syntax with class. We not only want to change the syntax to property binding syntax, but also we want to change the text color of hello world to yellow green after 2 seconds. To make this happen I am going to create another variable inside the component class called text_color_class. Our HTML looks like this:
<h1>My profile</h1>
<b [class]="text_color_class"> Hello World </b> <br/>
My name is Md. {{ name }} <br/>
I am a {{ profession }}. <br/>
Do not forget to visit me at: {{ website }} <br/>
My Social Links:
<ol>
<li *ngFor="let link_obj of social_links">
<a [class]="'red_border'" *ngIf="link_obj.is_active; else elseTemplate" [href]="link_obj.link"> {{ link_obj.title }} </a>
<ng-template #elseTemplate>
<s> This link is not active </s>
</ng-template>
</li>
</ol>
Notice the class value in a tag is "'red_border'" instead of "red_border"? This is because in property binding syntax the right side of the equal sign is evaluated. So, we need to provide a string there, otherwise Angular will mistake that as a variable and an undefined will be placed there.
Our component class will look like below.
class AppComponent {
name = "Md. Sabuj Sarker";
profession = "Software Engineer";
website = "http://sabuj.me";
social_links: ISocialLink[] = [
{ title: "Facebook", link: "https://facebook.com/SabujXiP", is_active: true },
{ title: "Twitter", link: "https://twitter.com/SabujXi", is_active: false },
{ title: "Github", link: "https://github.com/SabujXi", is_active: true }
];
text_color_class = "white green_bg";
constructor(){
setTimeout(() => {
this.text_color_class = "yellowgreen green_bg";
}, 2000);
}
}
Notice that we have repeated the class green_bg in two places to make that persistent. We will improve that in some future lesson if we face this again. For now, it will be easy for you to understand. We will also talk about dot notated class binding later.
Style Binding
style is a global property of every DOM element. We can directly bind to it with binding syntax. Let's remove underline from our anchors with style binding. To do so, let's create a variable in our class named link_style, so our component class will have this additional line of code.
link_style = "none";
And our a tag in the HTML will be changed to this:
<a [style.text-decoration]="link_style" [class]="'red_border'" *ngIf="link_obj.is_active; else elseTemplate" [href]="link_obj.link"> {{ link_obj.title }} </a>
Notice that we have used dot notation to specify which style we want to change in the property binding syntax.
To see dynamic change after 4 seconds we can add these lines of code below inside the constructor.
setTimeout(() => {
this.link_style = "underline";
}, 4000);
Go to the browser to see the changes happening.
Conclusion
Angular made interactive changes in styling our HTML a lot easier. If it seems hard at this point, it will seem very enjoyable once you grasp all the angular specific concepts properly. Next we will be discussing event binding with Angular.
About the Author
My name is Md. Sabuj Sarker. I am a Software Engineer, Trainer and Writer. I have over 10 years of experience in software development, web design and development, training, writing and some other cool stuff including few years of experience in mobile application development. I am also an open source contributor. Visit my github repository with username SabujXi.
Recent Stories
Top DiscoverSDK Experts
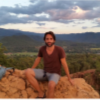
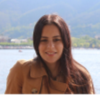
Compare Products
Select up to three two products to compare by clicking on the compare icon () of each product.
{{compareToolModel.Error}}
{{CommentsModel.TotalCount}} Comments
Your Comment