Big Numbers in JavaScript
One of the best things that the new JavaScript standard ES2020 brings with it is BitInt, which allows us—finally—to work with big numbers.
Until today, the only type of number object we had in JavaScript was Number, whose maximum value was 9007199254740992. With Number, you couldn’t go any higher than this. Don’t believe me? Run the following code and see what happens:
let myNumber = 9007199254740992;
myNumber++;
console.log('myNumber', myNumber); //9007199254740992
We would have to get access to this numeric constant through Number.MAX_SAFE_INTEGER. For instance:
const max = Number.MAX_SAFE_INTEGER;
console.log(max); //9007199254740991
One less than 9007199254740992—since with 9007199254740991 we can still perform all of the normal actions including increasing.
This wasn’t a problem in the olden days of JavaScript. But as JavaScript started to be implemented in different places and using it for increasingly complex tasks, this issue started to be very problematic. It was possible to work with big numbers in JavaScript but we needed to use all kinds of external libraries and hacks. The new ES2020 standard allows us to work with a new data type called BigInt which can handle the big numbers. Working with Bigint in JavaScript is easy as can be. Check it out:
let myNumber = BigInt(9007199254740992);
myNumber++;
console.log('myNumber', myNumber.toString()); //9007199254740993
Alternatively, attaching the letter ‘n’ to the number automatically turns it into a BigInt:
let myNumber = 9007199254740992n;
myNumber++;
console.log('myNumber', myNumber.toString()); //9007199254740993
console.log(typeof myNumber); //bigint
It’s important to note that we’re talking about a primitive data type that’s completely different. If you try to compare 42 to 42n, it will return false. This is just like if you tried to compare a text string to a number. And if you want to make a comparison? Well, you’ll have to convert the type—or the number to BigInt, or the BigInt to a number …
console.log(42 == 42n); //true
console.log(42 === 42n); //false
console.log(42 === Number(42n)); //true
console.log(BigInt(42) === 42n); //true
With BigInt, you can perform mathematical operations or whatever you want. If you want to add a text string or a number, first you’ve got to convert it. Also note, decimals aren’t supported with BigInt—if there are any they’ll be rounded up.
const rounded = 5n / 2n;
console.log('rounded', rounded.toString()); //2
Long story short, BigInt in JavaScript is very useful when performing complex time calculations that require the use of large numbers, and with other calculations as well. So if you happen to come across a mysterious ‘n’ somewhere in your code, now you’ll know exactly where it came from. Currently, this code works in Firefox and Chrome. Using Edge? Well, looks like your still stuck in the middle ages 🙂
About the author: Ran Bar-Zik is an experienced web developer whose personal blog, Internet Israel, features articles and guides on Node.js, MongoDB, Git, SASS, jQuery, HTML 5, MySQL, and more. Translation of the original article by Aaron Raizen.
Recent Stories
Top DiscoverSDK Experts
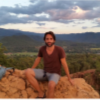
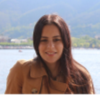
Compare Products
Select up to three two products to compare by clicking on the compare icon () of each product.
{{compareToolModel.Error}}
{{CommentsModel.TotalCount}} Comments
Your Comment