10 Angular 2 Modules and Tools you need to learn in 2017
Angular 2 is a very popular JavaScript framework for building rich web sites and mobile applications. As a result of the big community, the source code that is open, and the framework design, developers all over the world build 3rd parties modules and tools to support the development. They add features, make other developers lives easy, and save the world from nuclear war! ☺
Prerequisites for Angular 2 development:
- Learn JavaScript (ES5, ES6)
- Learn TypeScript
- Learn Angular 2 basics (modules, components, templates, views, routes, http, …)
- Learn 3rd party tools, modules and libraries
In this post I’ll go over 10 angular 2 modules every developer needs to learn and use (the order is random).
IONIC is an open source framework for creating amazing mobile apps that also works seamlessly on the desktop. You can write your code once and deploy it to IOS , android, windows and more. It has many native plugins for many device features like Bluetooth, fingerprint sensors and more. It performs well and is extremely easy to use.
Features:
- Open source
- Full cross-platform support - Android, iOS, Windows, Linux, OSX
- CLI tools and IONIC creator
- Many UI components
- Fully documented
- Extensible
- License: MIT
See the site for beautiful examples and documentation.
Material design for Angular 2
Site: https://material.angular.io
Github : https://github.com/angular/material2
Material design is an Angular 2 module that provides a rich set of UI components to integrate into your applications. All the components are modern UI components that work across the web, mobile and desktop.
Features:
- Cross-platform support
- Fast and consistent interface
- Themable
- Highly optimized
- Input controls
- Layouts support
- Navigation controls
- Popups and dialogs
- License: MIT
To use material design components you need to install it using npm and add a reference to your app.module.ts file:
import { MaterialModule } from '@angular/material';
// other imports
@NgModule({
imports: [MaterialModule.forRoot()],
...
})
export class PizzaPartyAppModule { }
For more examples and great documentation see the site/github repository.
Angular in memory web API
https://www.npmjs.com/package/angular-in-memory-web-api
While developing an angular 2 application, the developer is depended on a server app/service for data management. If you don’t want to rely on the server side developer and still be able to test your code, this module will be very helpful for you. You can create http requests and get an observable object the same way you get from a real server
Features
- Simulate a live web environment for testing your app
- Handle http request in memory
- Allows pass-through of certain requests
- Includes timing delays if desired, to simulate real response and traffic
- License: MIT
To use the module app a reference in your app.module.ts file:
import { HttpModule } from '@angular/http';
import { InMemoryWebApiModule } from 'angular-in-memory-web-api';
import { InMemHeroService } from '../app/hero-data';
@NgModule({
imports: [
HttpModule,
InMemoryWebApiModule.forRoot(InMemHeroService),
...
],
...
})
export class AppModule { ... }
See the site for more information and code examples
Angular Meteor
Angular meteor is a framework for building realtime and full stack applications with Angular and meteor. It provides real time data sync/store and let you write Angular code also on the server. It is also integrated with IONIC framework mentioned above
Features
- Use existing frameworks (such as Blaze) with Angular2.
- Easy integration
- Easy deployment
- Cordova integration
- Full documentations and examples
- License: MIT
https://angular-meteor.com/tutorials/whatsapp2-tutorial is a nice tutorial that shows you the power of the combination to build a whatsapp application.
Angular Doc
Documenting your code can be very painful – as developers we want to write code, not documentation. A good software development process must include a documentation step, and with this tool, you can make the process a lot easier. Just point angulardoc at your github repo and go!
Features:
- Repository management with a dashboard
- Architecture diagrams
- Class browser
- Modules diagram
- Route tree diagram
- Import diagram
See the site for examples and more information
Ng2 Charts
http://valor-software.com/ng2-charts/
If you want to include graphs in your applications you can use Chart.js
Ng2 charts brings the popular Chart.js functionality to Angular2, and helps you generate many types of graphs to visualize your data.
Features:
- Generates many types of charts
- Allows interaction with the charts via event callbacks
- Familiar interface for Chart.js users
- Easy integration
- Full documentation
- License: MIT
For example, to create a simple chart:
HTML:
<div style="display: block">
<canvas baseChart
[data]="doughnutChartData"
[labels]="doughnutChartLabels"
[chartType]="doughnutChartType"
(chartHover)="chartHovered($event)"
(chartClick)="chartClicked($event)"></canvas>
</div>
TypeScript
import { Component } from '@angular/core';
@Component({
selector: 'doughnut-chart-demo',
templateUrl: './doughnut-chart-demo.html'
})
export class DoughnutChartDemoComponent {
// Doughnut
public doughnutChartLabels:string[] = ['Download Sales', 'In-Store Sales', 'Mail-Order Sales'];
public doughnutChartData:number[] = [350, 450, 100];
public doughnutChartType:string = 'doughnut';
// events
public chartClicked(e:any):void {
console.log(e);
}
public chartHovered(e:any):void {
console.log(e);
}
}
For more information and examples see the site
Ng2 CKEditor
https://www.npmjs.com/package/ng2-ckeditor
If you build a site that users need to write text using a smart editor (writing comments, blog etc.) you can integrate CKEditor and you’ll get it easy. Ng2-ckeditor is an Angular 2 CKEditor component.
Features:
- Open source
- WYSIWYG editor
- Supports all the standard formatting
- Plugins support
- Easy to use and integrate
- License: MIT
Example:
import { CKEditorModule } from 'ng2-ckeditor';
@NgModule({
// ...
imports: [
CKEditorModule
],
// ...
})
export class AppModule { }
import { Component } from '@angular/core';
@Component({
selector: 'sample',
template: `
<ckeditor
[(ngModel)]="ckeditorContent"
[config]="{uiColor: '#99000'}"
(change)="onChange($event)"
(ready)="onReady($event)"
(focus)="onFocus($event)"
(blur)="onBlur($event)"
debounce="500">
</ckeditor>
`
})
export class Sample{
constructor(){
this.ckeditorContent = `<p>My HTML</p>`;
}
}
For more information and live demo see the site
AngularFire 2
https://github.com/angular/angularfire2
Firebase is a great toolset and infrastructure for building applications. AngularFire 2 is the official library for firebase and Angular 2. It helps with realtime binding, rxjs, authentication and more.
Features:
- Open source
- Observable based - use rxjs, Firebase, or Angular2
- Synchronized database collections
- Authentication
- Retrieve data as objects
- License: MIT
Example:
import {Component} from '@angular/core';
import {AngularFire, FirebaseListObservable} from 'angularfire2';
@Component({
selector: 'project-name-app',
template: `
<ul>
<li *ngFor="let item of items | async">
{{ item.name }}
</li>
</ul>
`
})
export class MyApp {
items: FirebaseListObservable<any[]>;
constructor(af: AngularFire) {
this.items = af.database.list('/items');
}
}
Ng2 Smart Table
https://akveo.github.io/ng2-smart-table/
This simple component help managing data in tables. Use it for free to display and edit data in place, filter by criteria, sort, and much more. It integrates easily with your Angular 2 components and fully documented:
Features:
- Local data source (Server/API DataSource is on its way)
- Filtering
- Sorting
- Pagination
- Inline Add/Edit/Delete
- Flexible event model
- License: MIT
Example:
@NgModule({
imports: [
// ...
Ng2SmartTableModule,
// ...
],
declarations: [ ... ]
})
and inside the component:
@Component({
template: `
<ng2-smart-table [settings]="settings"></ng2-smart-table>
`
})
Live demo is on the github page or on:
https://akveo.github.io/ng2-smart-table/demo
PrimeNG
http://www.primefaces.org/primeng/
PrimeNG is a collection of rich UI components for Angular 2. PrimeNG is a sibling of the popular JavaServer Faces Component Suite. It’s open source, free to use and will upgrade your applications’ look and feel.
Features:
- Very large set of widgets
- Simple and powerful
- Themable
- Active community
- Touch screen optimizations
- Well-integrated
- License: MIT
Its also well documented with many samples online.
Recent Stories
Top DiscoverSDK Experts
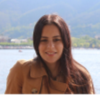
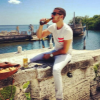
Compare Products
Select up to three two products to compare by clicking on the compare icon () of each product.
{{compareToolModel.Error}}
{{CommentsModel.TotalCount}} Comments
Your Comment