Writing a fault handler
#include <unistd.h>
#include <signal.h>
#include <stdio.h>
#include <stdlib.h>
#include <sys/types.h>
#include <sys/wait.h>
#include <signal.h>
#define __USE_GNU
#include <sys/ucontext.h>
#define REG_PC 31
void setHandler(void (*handler)(int,siginfo_t *,void *))
{
struct sigaction action;
action.sa_flags = SA_SIGINFO;
action.sa_sigaction = handler;
if (sigaction(SIGFPE, &action, NULL) == -1) {
perror("sigusr: sigaction");
_exit(1);
}
if (sigaction(SIGSEGV, &action, NULL) == -1) {
perror("sigusr: sigaction");
_exit(1);
}
if (sigaction(SIGILL, &action, NULL) == -1) {
perror("sigusr: sigaction");
_exit(1);
}
if (sigaction(SIGBUS, &action, NULL) == -1) {
perror("sigusr: sigaction");
_exit(1);
}
}
void catchit(int signo, siginfo_t *info, void *extra)
{
ucontext_t *p=(ucontext_t *)extra;
int x;
printf("Signal %d received from parent\n", signo);
x= p->uc_mcontext.gregs[REG_PC];
printf("address = %x\n",x);
printf("siginfo address=%x\n",info->si_addr);
setHandler(SIG_DFL);
//abort();
}
int main()
{
int x=9,y=0,z;
int *p=20;
setHandler(catchit);
//*p=100;
z=x/y;
printf("%d\n",z);
return 0;
}
Recent Stories
Top DiscoverSDK Experts
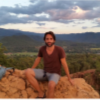
Mendy Bennett
Experienced with Ad network & Ad servers.
Mobile | Ad Networks and 1 more
View Profile
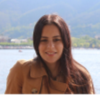
Karen Fitzgerald
7 years in Cross-Platform development.
Mobile | Cross Platform Frameworks
View Profile
X
Compare Products
Select up to three two products to compare by clicking on the compare icon () of each product.
{{compareToolModel.Error}}Now comparing:
{{product.ProductName | createSubstring:25}} X
{{CommentsModel.TotalCount}} Comments
Your Comment