Using Lists In Python
Lists are the best way to store values and loop results in Python. They are containers of things, which are organized from the first to the last. In this article, I will go over the ways to use lists.
First of all, this is how to create and view a list:
>>> YourListName = [‘Value1’, ‘Value2’, ‘Value3’]
>>> list(YourListName)
[‘Value1’, ‘Value2’, ‘Value3’]
Basic Commands:
The ‘append’ command:
>>> YourListName. append(‘Value4’)
>>> list(YourListName)
[‘Value1’, ‘Value2’, ‘Value3’, ‘Value4’]
Another command is ‘extend’, which will also add a new value at the end of the list:
>>> YourListName.extend(‘Value4’)
>>> list(YourListName)
[‘Value1’, ‘Value2’, ‘Value3’, ‘V’, ‘a’, ‘l’, ‘u’, ‘e’, ‘4’]
The ‘insert’ command:
>>> YourListName.insert(2, ‘Value4’)
>>> list(YourListName)
[‘Value1’, ‘Value2’, ‘Value4’, ‘Value3’]
Keep in mind that the counting start from 0 and not from 1.
The ‘remove’ command:
>>> YourListName.remove(‘Value2’)
>>> list(YourListName)
[‘Value1’, ‘Value3’]
If no such item is found in the list, an error message will be displayed.
The ‘pop’ command:
>>> YourListName.pop(1)
‘Value2’
>>> list(YourListName)
[‘Value1’, ‘Value3’]
The ‘clear’ command:
>>> YourListName.clear()
>>> list(YourListName)
[]
The ‘index’ command:
>>> YourListName.index(‘Value3’)
2
If no such item is found in the list, an error message will be displayed.
The ‘count’ command:
>>> YourListName.count(‘Value3’)
1
The ‘sort’ command:
>>> list(YourListName)
[‘2’, ‘5’, ‘3’, ‘6’, ‘1’, ‘4’]
>>> YourListName.sort()
>>> list(YourListName)
[‘1’, ‘2’, ‘3’, ‘4’, ‘5’, ‘6’]
You can use the parentheses for customization (For example: (key=None, reverse=False)), but it’s not a requirement.
You can also use the ‘sorted’ function, in case you need the original list in addition to the sorted one:
>>> sorted(YourListName)
[‘1’, ‘2’, ‘3’, ‘4’, ‘5’, ‘6’]
>>> list(YourListName)
[‘2’, ‘5’, ‘3’, ‘6’, ‘1’, ‘4’]
The ‘reverse’ command:
>>> list(YourListName)
[‘1’, ‘2’, ‘3’, ‘4’, ‘5’, ‘6’]
>>> YourListName.reverse()
[‘6’, ‘5’, ‘4’, ‘3’, ‘2’, ‘1’]
The ‘copy’ command:
>>> list(YourListName)
[‘Value1’, ‘Value2’, ‘Value3’]
>>> YourListName.reverse()
[‘Value1’, ‘Value2’, ‘Value3’]
The ‘del’ command:
>>> list(YourListName)
[‘Value1’, ‘Value2’, ‘Value3’]
>>> del YourListName[1]
>>> list(YourListName)
[‘Value1’, ‘Value3’]
And you can also use this command to delete several items in the same time:
>>> list(YourListName)
[‘1’, ‘2’, ‘3’, ‘4’, ‘5’, ‘6’]
>>> del YourListName[1:3]
>>> list(YourListName)
[‘1’, ‘4’, ‘5’, ‘6’]
Additional Coommands:
The ‘len’ command to check a list’s length:
>>> len(YourListName)
3
The ‘+’ command for the concatenation of several lists:
>>> list(YourListName)
[‘1’, ‘2’, ‘3’]
>>> list(ListName2)
[‘4’, ‘5’, ‘6’]
>>> YourListName + ListName2
[‘1’, ‘2’, ‘3’, ‘4’, ‘5’, ‘6’]
The ‘*’ command for repetition of a list:
>>> list(YourListName)
[‘1’, ‘2’, ‘3’]
>>> YourListName * 4
[‘1’, ‘2’, ‘3’, ‘1’, ‘2’, ‘3’, ‘1’, ‘2’, ‘3’, ‘1’, ‘2’, ‘3’]
You can also use the ‘*’ command to multiply the items inside a list (if they are numerical):
>>> YourListName = [0, 1, 2]
>>> ListName2 = [x*3 for x in a1]
>>> ListName2
[0, 3, 6]
Or
>>> YourListName = [x*3 for x in a1]
>>> YourListName
[0, 3, 6]
The ‘in’ command for membership checking:
>>> list(YourListName)
[‘1’, ‘2’, ‘3’]
>>> ‘3’ in YourListName
True
>>> ‘159’ in YourListName
False
The ‘for x in YourListName: print x’ command for iteration:
>>> for x in YourlistName:
… print (x)
…
1
2
3
The ‘[]’ command, for displaying a certain item buy it’s location in the list:
>>> list(YourListName)
[‘1’, ‘2’, ‘3’]
>>> YourListName[2]
‘3’
Keep in mind that the counting starts from 0.
The ‘[-]’ command, for displaying a certain item buy it’s location in the list when starting the counting from the right:
>>> list(YourListName)
[‘1’, ‘2’, ‘3’]
>>> YourListName[-2]
‘2’
Keep in mind that in this case, the counting starts from 1.
The ‘[:]’ command, for displaying the items in the list starting and/or ending at a certain position:
>>> list(YourListName)
[‘1’, ‘2’, ‘3’, ‘4’, ‘5’, ‘6’]
>>> YourListName[1:]
[‘2’, ‘3’ ‘4’, ‘5’, ‘6’]
Keep in mind that the counting starts from 0.
You can also use the ‘[:]’ to display the items in the list when skipping a certain amount of items each time:
>>> list(YourListName)
[‘1’, ‘2’, ‘3’, ‘4’, ‘5’, ‘6’, ‘7’, ‘8’, ‘9’, ‘10’]
>>> YourListName[1:8:2]
[‘2’, ‘4’, ‘6’, ‘8’]
The ‘min’ command for receiving the item with the lowest value in the list:
>>> list(YourListName)
[‘1’, ‘2’, ‘3’, ‘4’, ‘5’, ‘6’, ‘7’, ‘8’, ‘9’, ‘10’]
>>> min(YourListName)
‘1’
The ‘max’ command for receiving the item with the highest value in the list:
>>> list(YourListName)
[‘1’, ‘2’, ‘3’, ‘4’, ‘5’, ‘6’, ‘7’, ‘8’, ‘9’, ‘10’]
>>> max(YourListName)
‘10’
List Comprehensions:
>>> YourListName = []
>>> for x in range(10):
… YourListName.append(x**2)
…
>>> YourListName
[0, 1, 4, 9, 16, 25, 36, 49, 64, 81]
Nested List Comprehensions:
>>> YourListName = [
… [1, 2, 3, 4],
… [5, 6, 7, 8],
… [9, 10, 11, 12],
… ]
>>> YourListName
[[1, 2, 3, 4], [5, 6, 7, 8], [9, 10, 11, 12],]
And you can also transpose rows and columns:
>>> [[row[i] for row in YourListName] for i in range(4)]
[[1, 5, 9], [2, 6, 10], [3, 7, 11], [4, 8, 12]]
This can also be done easily with the built-in ‘zip’ function:
>>> list(zip(*YourListName))
[(1, 5, 9), (2, 6, 10), (3, 7, 11), (4, 8, 12)]
Recent Stories
Top DiscoverSDK Experts
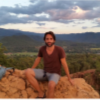
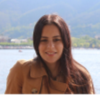
Compare Products
Select up to three two products to compare by clicking on the compare icon () of each product.
{{compareToolModel.Error}}
{{CommentsModel.TotalCount}} Comments
Your Comment