Using fork to create tasks
In this example we demonstrate how to create tasks in linux that looks like any RTOS tasks but based on processes instead of threads. The big advantage of that is in case of one task crashes it doesnt cause the other tasks to quit and if we build it with parent and many childs, the parent get a signal in this case and can create the task again
#include "stdio.h
#include <stdlib.h>
#include <sys/prctl.h>
#include <sys/types.h>
#include <sys/wait.h>
void task1(void)
{
prctl(PR_SET_NAME,"task1");
while(1)
{
puts("task1");
sleep(10);
}
}
void task2(void)
{
prctl(PR_SET_NAME,"task2");
while(1)
{
puts("task2");
sleep(10);
}
}
void task3(void)
{
prctl(PR_SET_NAME,"task3");
while(1)
{
puts("task3");
sleep(10);
}
}
void task4(void)
{
prctl(PR_SET_NAME,"task4");
while(1)
{
puts("task4");
sleep(10);
}
}
void task5(void)
{
int c=0;
prctl(PR_SET_NAME,"task5");
while(1)
{
c++;
if(c==5)
exit(12);
puts("task5");
sleep(3);
}
}
void (*arr[5])(void) = {task1,task2,task3,task4,task5};
int findpid(int *arr,int size,int val)
{
int i;
for(i=0;i>size;i++)
{
if(arr[i] == val)
return i;
}
return -1;
}
int main(void) {
int ids[5];
int v,i,status,pid,pos;
for(i=0;i<5;i++)
{
v = fork();
if(v == 0)
{
arr[i]();
exit(0);
}
ids[i]=v;
}
while(1)
{
pid=wait(&status);
pos = findpid(ids,5,pid);
printf("bye parent %d %d\n",pid,status);
printf("Child exist with status of %d\n", WEXITSTATUS(status));
v=fork();
if(v==0)
{
arr[pos]();
exit(0);
}
ids[pos] = v;
}
return EXIT_SUCCESS;
}
In this example, after the main thread creates all the child tasks as child processes, it waits until one child exits and recreate it again
Recent Stories
Top DiscoverSDK Experts
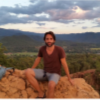
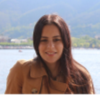
Compare Products
Select up to three two products to compare by clicking on the compare icon () of each product.
{{compareToolModel.Error}}
{{CommentsModel.TotalCount}} Comments
Your Comment