Useful JNI Snippets
Some useful snippets for working with JNI in application development and AOSP developmenta
Simple C++ code
static jint add(JNIEnv *env, jclass clazz, jint a,jint b) {
return a+b;
}
static jint sub(JNIEnv *env, jclass clazz, jint a,jint b) {
return a-b;
}
static JNINativeMethod method_table[] = {
{ "add", "(II)I", (void *) add },
{ "libSub", "(II)I", (void *) sub },
};
jint JNI_OnLoad(JavaVM* vm, void* reserved) {
JNIEnv* env;
if (vm->GetEnv(reinterpret_cast<void**>(&env), JNI_VERSION_1_6) != JNI_OK) {
return JNI_ERR;
} else {
jclass clazz = env->FindClass("com/example/simpcpp/MainActivity");
if (clazz) {
jint ret = env->RegisterNatives(clazz, method_table, sizeof(method_table) / sizeof(method_table[0]));
env->DeleteLocalRef(clazz);
return ret == 0 ? JNI_VERSION_1_6 : JNI_ERR;
} else {
return JNI_ERR;
}
}
}
The Java code
public static native int add(int a,int b);
public static native int libSub(int a,int b);
static
{
System.loadLibrary("SimpCpp");
}
Using Log
__android_log_print(ANDROID_LOG_DEBUG, "myfile.c", "add(%lld)", n);
// Add to Android.mk:
// LOCAL_LDLIBS += -llog
Thread function:
static void *threadfn(void *p)
{
jlong res = fib((long)p);
JNIEnv *env;
int n=javaVM->AttachCurrentThread(&env,NULL);
jmethodID m=env->GetStaticMethodID(cl,"printToLog","(J)V");
env->CallStaticVoidMethod(cl,m,res);
javaVM->DetachCurrentThread();
return (void*)res;
}
static jlong fibNR11(JNIEnv *env, jclass clazz, jlong n) {
pthread_t t1;
pthread_create(&t1,NULL,threadfn,(void*)n);
return 0;
}
Using Reflection
JNIEXPORT jint JNICALL Java_com_example_testjni_Nlib_sub
(JNIEnv *env, jobject obj, jint a, jint b)
{
jclass cl=env->GetObjectClass(obj);
jmethodID m=env->GetMethodID(cl,"getNum","(I)I");
jint num=env->CallIntMethod(obj,m,a+b);
return num;
}
Recent Stories
Top DiscoverSDK Experts
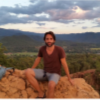
Mendy Bennett
Experienced with Ad network & Ad servers.
Mobile | Ad Networks and 1 more
View Profile
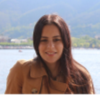
Karen Fitzgerald
7 years in Cross-Platform development.
Mobile | Cross Platform Frameworks
View Profile
X
Compare Products
Select up to three two products to compare by clicking on the compare icon () of each product.
{{compareToolModel.Error}}Now comparing:
{{product.ProductName | createSubstring:25}} X
{{CommentsModel.TotalCount}} Comments
Your Comment