Python Tutorial for Beginners
Python Tutorial
Unlike Matlab, Python is not dependent any development environment.
First enter into the shell chosen from the introduction.
Now We'll make a small examples:
The print function, (\n allows linebreak):
In [1]: print "a\nb\nc\n"
Out [1]:
a
b
c
You can also write several rows code in IPython, and activate whole
At once by pressing ctrl-enter at the end of every line, and than press enter for activate
The code section:
In [2]: print "Discover"
print "SDK"
Out [2]:
Discover
SDK
What we do When IPython stuck?
L-Ctrl -> erases the commande window, and fix the stuck.
.-Ctrl - initializes the IPython without closing the user interface.
Numbers:
Basically you can perform arithmetic operations on the command line:
#In [2]: 5 * (10 + 1)
Out[2]: 55
Notice that unlike other programming languages, there is no need to set numbers by type. On the one hand it’s more user-friendly and allows easy operation. and on the other hand it’s necessary to define variables with significant names that Include the numbers type.
For example:
Modulo with float type:
In [3]: 7.24 % 3
Out[3]: 1.2400000000000002
power of two numbers:
In [4]: 5**2
Out[4]: 25
Automatic conversion:
>>> a=3 {int}
>>> b=5.3 {float}
>>> c=a+b
>>> c =>8.3 {c type is float}
>>> a=2.0
>>> b=3.0
>>> (a+b)/2
2.5
Application of Variables:
In [5]: a = 2
In [6]: a /= 2
In [7]: a
Out[7]: 1
The following function receives a variable and returns its type:
>>> type(13.4)
<type 'float'>
>>> type('hello')
<type 'str'>
Lists:
In [1]: lst = [1, 2, 3,5,6,7]
In [2]: type(lst)
Out[2]: list
Create List Can be done by the function range():
In [3]: range(7)
Out[3]: [0, 1, 2, 3, 4, 5, 6]
In [4]: lst = range(10, 30, 3)
In [5]: lst
Out[5]: [10, 13, 16, 19, 22, 25, 28]
Keeping in mind that starting from zero, the range does not include the target index:
In [6]: lst[3:9]
Out[6]: [19, 22, 25, 28]
Connecting between the two lists is defined as a combination of list:
In [7]: a=[21, 22, 23, 24, 25]
In [8]: b=[26, 27, 28, 29,29]
In [9]: a + b
Out[9]: [21, 22, 23, 24, 25,26, 27, 28, 29,29]
Tuples:
At first sight behave like a list, only with round brackets instead
Square.
In [1]: b = (7, 6,5, 4)
In [2]: b
Out[3]: (7, 6, 5, 4
The main difference is they are immutable:
b[1]=2
TypeError: 'tuple' object does not support item assignment
Call by refernce:
The most programming languages adopt this method.
No need to write a special way to achieve Speed and efficiency.
You have to remember only one thing - Mounting variables) as well as transfer
Arguments to functions (no copy, if you want a copy, must be made It explicitly.
In Matlab situation is reversed. Always being copied, if you want to improve
Speed or save memory, need to learn techniques,(for example, Local functions).
In [1]: a = [a, 2, 'cmd']
In [2]: b = a
In [3]: b[1] = 400 ------------------------> a changed
In [4]: a
Out[4]: [10, 400, 'cmd']
Strings:
Can be viewed in two equivalent main forms
In [1]: "Discover SDK"
In[2]: 'Discover SDK'
Connect strings with each other but not with a number
In [3]: g = 'Dsicover'
In [4]: f = 'SDK'
In [5]: g + f
Out[5]: 'DiscoverSDK'
In [6]: g + ' ' + f
Out[6]: 'Discover SDK'
In [6] a="sdk"
Out[6] a+5
TypeError: cannot concatenate 'str' and 'int' objects
Multiplication:
In [42]: 'sDk' * 3
Out[42]: 'sDksDksDk'
Conditions:
One special features of the Python language is Running condition code by indentation.
if b > a:
print '"a" is small and equal to %s' %a
else:
print '"b" is small and equal to %s' %b
For several conditions(Note that displacement Is crucial) :
>>> x=100
>>> y=200
>>> c=300
>>> if x<y and y<c:
print "the maximum number is c"
elif y<x and c<x:
print "the maximum number is x"
elif x<y and c<y:
print "the maximum number is y"
the maximum number is c
loops:
For:
Different from other languages, for-loops do not promote the loop index. But going through the parts list:
In [1]: a=['a','b','c','d','e']
In [2]: for i in a:
print(i)
Out[2]:
a
b
c
d
e
In [1]: for i in range(5):
print "%d\n" %i
Out[1]:
0
1
2
3
4
While:
While loop ran continuously until the Loop condition return True value.
>>> A=7
>>> while A < 10:
A+=1
>>> A
10
Another common use is to create an infinite loop:
>>> a=4
>>> b=5
>>> while True:
if a==b:
break
a+=1
>>> a
5
break,continue :
continue, promoting to the next iteration.
Break command, goes out of the loop.
>>> a=4
>>> b=5
>>> while a<b:
a+=1
if (b==a):
break
>>> a
5
>>> for st in ['Discover','SDK','Cloud']:
if st!='SDK':
continue
else:
break
>>> st
'SDK'
Functions:
Now we use more IDE - Write code to the file by editor. You can
Write in IDLE, PyScripter, spyder or pycharm and run through.
You can also run through a DOS window of Windows, or through the IPython.
As usual indentation is necessary:
>>> def Num_plus_1(num):
num+=1
print "%d" %num
>>> Num_plus_1(4)
5
>>>def max1(a,b):
if a>b:
return a
return b
>>> max1(1,9)
9
Global and local Variables
Variables defined in a function are local variables.
To define a global variable we use the following command.
Definition of a global variable:
global Var
If we'll try to change a global variable in a function, the verianble will not change and a local variable with the same value will created:
>>> def Local(a):
b=34
a=b------> Local variable
return a
>>> local(a)
34
>>> a-------> The vlaue of the global variable has'nt changed
54
It is possible to change a global variable in the function:
>>> global a
>>> a=54
>>> def glob():
global a
a=34
return
>>> a
54
>>> glob()
>>> a
34
>>>
In case "a" does not exist. It will created inside the function.
- Note that the use of global variables in such a manner is not recommended
Logical Expressions
simple use of logical expressions
>>> 2!=4
True
>>> 3>2
True
>>> 2>3
False
>>> 2!=2
False
the Not operator:
>>> not 10<5
True
>>> not 5!=5
True
Easy and Effective way to find out if an organ is on the list:
>>> list1=[0,1,2,3,4,5,6,7,8,9]
>>> 10 in list1
False
>>> 2 in list1
True
A similar implementation for strings:
>>> str= "Discover SDK"
>>> 'SDK' in str
True
>>> 'discover' in str
False
Python defines a relationship between strings, and a relationship based on
Organ between lists and tuples:
>>> 'e'>'d'>'a'
True
>>> 'H'>'G'>='A'=='A'
True
>>> [1,5,8]>[1,5,6]
True
>>> [50] > [1,2,3,4,5,6,7]
True
Recent Stories
Top DiscoverSDK Experts
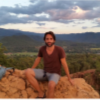
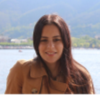
Compare Products
Select up to three two products to compare by clicking on the compare icon () of each product.
{{compareToolModel.Error}}
{{CommentsModel.TotalCount}} Comments
Your Comment