PHP Tutorial - Part 1
PHP is a server-side scripting language designed and used mostly for web development, but can also be used for general-purpose programming.
PHP Opening And Closing Tags:
PHP codes always begin with an Opening PHP Tag. PHP codes can end with a Closing PHP Tag, but it is only needed in cases where the PHP code is being used together with HTML codes. This is how they look:
<?php
YourCode
?>
In PHP there are constants and variables, each can contain one of these data types:
- Boolean - Can have only one of two possible values - True or False.
- Integer - Positive and negative simple numbers (without any points or other complications).
- Float - Positive and negative numbers which include a point in them.
- String - Verbal phrases only.
- Array - Stores multiple values in a single variable.
- Resource - It is the storing of a reference to functions and resources outside PHP.
- Object - Stores data and information about how to process it.
- NULL - Can have only one value - NULL, which means there is no value assigned to it at all.
Keep in mind that variables in PHP are case-sensitive.
There are three ways to write comments in PHP:
- // C++ Comment Style
- /*
C-Style Comment Block
*/ - # Shell Style Comment
Functions:
Functions are pieces of code programmed to perform a specific task defined by the programmer. They are meant for using over and over throughout the code, in order to make the programming faster and easier. Functions are created and used this way:
<?php
Function YourFunctionName(OptionalVariable1, OptionalVariable2)
{
DesiredActions
}
YourFunctionName(OptionalVariable1, OptionalVariable2);
?>
Keep in mind that the variables are optional.
Returning Values:
Sometimes we want to return values into variables so we can use them. It is done by putting a ‘return’ command in a function, and defining a variable’s value as the function itself. For example:
<?php
function YoutFunctionName()
{
return SomeValue;
}
$VariableName = YoutFunctionName();
echo $VariableName;
?>
Variable Functions:
In PHP we are also able to call functions by using variable names. It is done like this:
<?php
Function YourFunctionName()
{
DesiredActions
}
$VariableName = “YourFunctionName”;
$VariableName();
?>
Variable Scope And Global Variables:
In PHP, the variables defined inside a function are only available there, and have no connection and/or effect on the variables outside of the function (Even if they both have the exact same name) - This is called a “Variable Scope”.
But, It is possible to turn a variable inside a function into a global variable - that way it will have an effect on the variable outside the function which has the identical name to the global variable. It is done like this:
<?php
$VariableName = SomeValue1
Function YourFunctionName()
{
Global $VariableName;
$VariableName = SomeValue2;
}
YourFunctionName();
echo $VariableName;
?>
Keep in mind that this feature will be effective only for variables which are inside a function (It will work on variables outside a function as well, but in that case it just won’t change anything).
Single And Double Quoted Strings:
You can create strings using single quotes, but there are a few cons to it. It prints what’s between the quotes as is - in other words, it is impossible to use the ‘\n’ command for printing a single string to multiple lines or combine variables in the string. Another disadvantage with single quoted strings is that using the single quote as a character in the string can be a bit confusing - you have to put a backslash right before the single quote in order for PHP to recognize it as part of the string, instead of its end. Beside that, using single quoted strings will give the same results as using double quoted strings. Here’s how to work with single quoted strings:
<?php
$VariableName = ‘It\’s My Site!’
echo $VariableName
?>
And the output will be:
It’s My Site!
In double quotes strings there are no issues with printing the single string to multiple lines, nor with combining variables within the string itself. There is an issue printing a double quote into a double quoted string, but the solution for this problem is the same solution for the single quote problem with single quoted strings - you just put a backslash right before the double quote, and everything is back in order. Another problem we have with combining a an integer right before a character in the string (for example, the phrase “3rd generation’ when the 3 is an integer). There are two solutions for this problem:
The first solution is putting ‘{}’ before and after the integer in the string:
<?php
$VariableName = 3
echo “{$VariableName}rd Generation”;
?>
The second solution is writing the integer right before the string itself, when there is a ‘.’ between them:
<?php
$VariableName = 3
echo $VariableName.“rd Generation”;
?>
In both solutions the output will be :
3rd Generation
Keep in mind that the first solution will also work for single quoted strings, while the second solution will not.
Continue In Part 2
Recent Stories
Top DiscoverSDK Experts
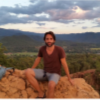
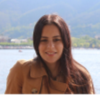
Compare Products
Select up to three two products to compare by clicking on the compare icon () of each product.
{{compareToolModel.Error}}
{{CommentsModel.TotalCount}} Comments
Your Comment