Numpy Tutorial
Numpy:
Numpy is one of the six libraries comprising the python science package.It provides commands for definition and operation arrays. Also performing various of mathematical operations.
numpy works quickly and consumes less memory.
To import the Numpy lets open the IDE and write the next command in the command line:
In [6]: import numpy as np
define a one-dimensional array:
In [20]: a = np.array([1, 2, 3])
In [21]: a
Out[21]: array([1, 2, 3])
Arithmetic operations between arrays:
In [22]: a*5
Out[22]: array([ 5, 10, 15])
b=a*5
In [24]: b=a*5
In [25]: a+b
Out[25]: array([ 6, 12, 18])
we can change the type of the elements of an array, regardless of their current type by following command:
For example, here is the 64-bit float:(Python's float equivalent to float64 of numpy)
In [26]: a=np.array([1, 2, 3], dtype=np.float64)
In [27]: a
Out[27]: array([ 1., 2., 3.])
the 8-bit int:
In [39]: a=np.array([1.6, 2.4, 3.9], dtype=np.int8)
In [40]: a
Out[40]: array([1, 2, 3], dtype=int8)
In [50]: np.array([1, 2, 3], dtype='f2')
Out[50]: array([ 1., 2., 3.], dtype=float16)
Another use to the type operators is to creat arrays:
np.float64([1, 2, 3])
Out[52]: array([ 1., 2., 3.])
Creating arrays with np.arange() equivalent to range() of python:
In [55]: np.arange(5)
Out[55]: array([0, 1, 2, 3, 4])
In [59]: np.arange(5, dtype=float)
Out[59]: array([ 0., 1., 2., 3., 4.])
In [61]: np.arange(5, 10)
Out[61]: array([5, 6, 7, 8, 9])
The third variable defines the distance between each element
In [62]: np.arange(5,6,0.2)
Out[62]: array([ 5. , 5.2, 5.4, 5.6, 5.8])
In [65]: np.arange(5, 4, -0.3)[:4]------>The last digit “4” is the number of elements displayed(slice).
Out[65]: array([ 5. , 4.7, 4.4, 4.1])
The following function performs a similar operation. note that if putting only two arguments the function will display 50 elemnts by default.
In [71]: np.linspace(2,3,5)
Out[71]: array([ 2. , 2.25, 2.5 , 2.75, 3. ])
In [82]: np.linspace(1,50)
Out[82]: array([ 1., 2., 3., 4., 5., 6., 7., 8., 9., 10., 11.,
12., 13., 14., 15., 16., 17., 18., 19., 20., 21., 22.,
23., 24., 25., 26., 27., 28., 29., 30., 31., 32., 33.,
34., 35., 36., 37., 38., 39., 40., 41., 42., 43., 44.,
45., 46., 47., 48., 49., 50.])
We can also get the distance between two organs by:
In [103]: a, dist = np.linspace(2, 3, 5,retstep=True)
In [104]: a
Out[104]: array([ 2. , 2.25, 2.5 , 2.75, 3. ])
In [105]: dist
Out[105]: 0.25
Help function:
max?
Docstring:
max(iterable[, key=func]) -> value
max(a, b, c, ...[, key=func]) -> value
With a single iterable argument, return its largest item.
With two or more arguments, return the largest argument.
Type: builtin_function_or_method
range?
Docstring:
range(stop) -> list of integers
range(start, stop[, step]) -> list of integers
Return a list containing an arithmetic progression of integers.
range(i, j) returns [i, i+1, i+2, ..., j-1]; start (!) defaults to 0.
When step is given, it specifies the increment (or decrement).
For example, range(4) returns [0, 1, 2, 3]. The end point is omitted!
These are exactly the valid indices for a list of 4 elements.
Type: builtin_function_or_method
Change array dimensional:
In [9]: a = np.arange(9)
In [10]: a
Out[10]: array([0, 1, 2, 3, 4, 5, 6, 7, 8])
In [11]: a.shape = (3, 3)
In [12]: a
Out[12]:
array([[0, 1, 2],
[3, 4, 5],
[6, 7, 8]])
Short definition of a two-dimensional array:
In [21]: a = np.arange(9).reshape(3, 3)
In [22]:a
Out[21]:
array([[0, 1, 2],
[3, 4, 5],
[6, 7, 8]])
For undo:
In [45]: a.shape = (-1)
In[46]: a
Out[46]: array([0, 1, 2, 3, 4, 5, 6, 7, 8])
The following command provides the shape of the original array but does not change the actual value of “a”:
In[47]: a.reshape(-1,)
Out[47]: array([0, 1, 2, 3, 4, 5, 6, 7, 8])
In [18]: a
Out [18]:
array([[0, 1, 2],
[3, 4, 5],
[6, 7, 8]])
Two-dimensional array analysis:
In [23]:a
Out[23]:
array([[0, 1, 2],
[3, 4, 5],
[6, 7, 8]])
In [24]: a[0,1]
Out[24]: 1
Two-dimensional array is an array of arrays so we can write:
In [25]: a[1]
Out[25]: array([3, 4, 5])
The following function behavior similar like Mathlab:
In [28]: a.item(8)
Out[28]: 8
In [29]: a.item(0)
Out[29]: 0
Create a square matrix:
Identity matrix:
In [32]: np.eye(3)
Out[32]:
array([[ 1., 0., 0.],
[ 0., 1., 0.],
[ 0., 0., 1.]])
You can chose other diagonal by choosing the value k. For example:
In [33]: np.eye(3, k=0)
Out[33]:
array([[ 1., 0., 0.],
[ 0., 1., 0.],
[ 0., 0., 1.]])
In [34]:np.eye(3, k=1)
Out[34]:
array([[ 0., 1., 0.],
[ 0., 0., 1.],
[ 0., 0., 0.]])
In [35]:np.eye(3, k=-1)
Out[35]:
array([[ 0., 0., 0.],
[ 1., 0., 0.],
[ 0., 1., 0.]])
Create vector of zeros:
In [43]: np.zeros(5)
Out[43]: array([ 0., 0., 0., 0., 0.])
In [44]: np.zeros((2, 2))
Out[44]:
array([[ 0., 0.],
[ 0., 0.]])
define identical none zero organs array:
In [58]: a = np.full((3, 3), 5, dtype=float)
In [59]: a
Out[59]:
array([[ 5., 5., 5.],
[ 5., 5., 5.],
[ 5., 5., 5.]])
In [60]: a = np.full((3), 5, dtype=float)
In [61]: a
Out[61]: array([ 5., 5., 5.])
In [60]: a = np.full((3), 5, dtype=float)
In [61]: a
Out[61]: array([ 5., 5., 5.])
Boolean Indexing:
Filtration array organs by Boolean expressions:
a = np.arange(5)
b = np.array([True, True,False,False,False])
b
Out[73]: array([ True, True, False, False, False], dtype=bool)
a[b]
Out[74]: array([0, 1])
For two-dimensional array (each organ is one-dimensional array):
a = np.arange(4).reshape(2, 2)
a
Out[83]:
array([[0, 1],
[2, 3]])
b = np.array([True,False])
a[b]
Out[85]: array([[0, 1]])
Another nice option is filtering by size:
Out[88]: array([0, 1, 2, 3, 4, 5, 6, 7, 8, 9])
a>7
Out[89]: array([False, False, False, False, False, False, False, False, True, True], dtype=bool)
a[a>7]
Out[90]: array([8, 9])
Note that the filtering is by index:(In this example, the output is an array containing the indexes of numbers that greater than 5 in array "a")
a=np.arange(5)
a
Out[95]: array([0, 1, 2, 3, 4])
a*3
Out[96]: array([ 0, 3, 6, 9, 12])
a[a * 3 > 5]
Out[97]: array([2, 3, 4])
For example, we can also get the number of the elements that are larger than 2 at "a":
In [99]:a
Out[99]: array([0, 1, 2, 3, 4])
In [100]:[a>2].size
Out[100]: 2
Another way to do it:
In [113]:(a > 2).sum()
Out[113]: 2
As part of the arithmetic action, the value of True is one and of False is zero:
In [116]: True+True
Out[116]: 2
In [117]: False+False
Out[117]: 0
In [118]: False+True
Out[118]: 1
Recent Stories
Top DiscoverSDK Experts
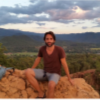
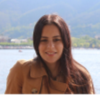
Compare Products
Select up to three two products to compare by clicking on the compare icon () of each product.
{{compareToolModel.Error}}
{{CommentsModel.TotalCount}} Comments
Your Comment