MIPS Assembly Examples with gcc
Simple function
.globl addfn;
.type addfn, @function;
.ent addfn
addfn:
add $a0,$a0,$a1;
move $v0,$a0;
.end addfn;
jr $ra;
Another Example
.globl myfn1;
.type myfn1, @function;
.ent myfn1
.set reorder
myfn1:
lw $t9,16($sp);
add $a0,$a0,$t9;
add $a0,$a0,$a1;
add $a0,$a0,$a2;
add $a0,$a0,$a3;
move $v0,$a0;
.end myfn1;
jr $ra;
Using the Functions
#include<stdio.h>
extern int myfn1(int,int,int,int,int);
extern int addfn(int,int);
int main()
{
printf("hello world!!! %d\n",myfn1(1,2,3,4,5));
return 1;
}
Example 3
.globl exe1;
.type exe1, @function;
.ent exe1
.set reorder
exe1:
move $t0,$a0;
move $t1,$a1;
li $v0,0;
loop:
lw $t2,0($t0);
add $v0,$v0,$t2;
addi $t0,$t0,4;
sub $t1,$t1,1;
bnez $t1,loop;
.end exe1;
jr $ra;
System Call
.globl myfn1;
.type myfn1, @function;
.ent myfn1
.set reorder
myfn1:
li $v0,4020;
syscall;
.end myfn1;
jr $ra;
Inline Assembly
#include<stdio.h>
#include<unistd.h>
extern int myfn1();
int assemfunction(int x,int y)
{
asm volatile (
"add $v0,$a0,$a1"
);
}
int add2(int x,int y)
{
int z;
asm volatile(
"move $t1,%2\n\t"
"add $t1,$t1,%1\n\t"
"move %0,$t1"
: "=r" (z)
: "r" (x),"r" (y)
: "t1"
);
return z;
}
void mymb()
{
asm volatile(
".set push\n\t"
".set noreorder\n\t"
".set mips2\n\t"
"sync\n\t"
".set pop"
:::"memory");
}
int getpid2()
{
asm volatile(
"li $v0,4020\n\t"
"syscall\n\t"
);
}
int main()
{
int f;
int r,t,q;
f=getpid2();
t=getpid();
printf("pid=%d pid2=%d\n",f,t);
r=9;
mymb();
q=5;
printf("res=%d\n",add2(5,8) );
return 1;
}
Recent Stories
Top DiscoverSDK Experts
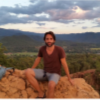
Mendy Bennett
Experienced with Ad network & Ad servers.
Mobile | Ad Networks and 1 more
View Profile
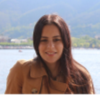
Karen Fitzgerald
7 years in Cross-Platform development.
Mobile | Cross Platform Frameworks
View Profile
X
Compare Products
Select up to three two products to compare by clicking on the compare icon () of each product.
{{compareToolModel.Error}}Now comparing:
{{product.ProductName | createSubstring:25}} X
{{CommentsModel.TotalCount}} Comments
Your Comment