JavaScript Tutorial Part 2
This is the second part of javaScript tutorial, before you can start working with javascript and javascript frameworks we need to learn all the basic concepts. If you are already familiar with that , jump to next tutorial
FUNCTIONS
There is something really important you need to learn if you want to code. Functions are the core of most programs. As you have seen in the sample codes, many of the lines have the “function” word on it. Basically a function is a program itself that does something useful for us. When we execute a function it is usually said invoking, applying or calling the function. In this book we will use the word calling a function when we mean we want to execute it. For example, we already reviewed the alert function. Alert is a function already made by the environment and it shows a dialog box with buttons to click. In the dialog box, we can write whatever we want and it will be shown.
What we are giving to the function is a value, in the form of a string, a number or a variable for it to show. We call these values, arguments and they are between parentheses when we pass them to the function. The alert function we already explored needs one argument but there are other functions that need more arguments or arguments of other types.
function addnumebers(num1, num2) {
return num1 + num2;
}
var res = addnumbers(100,200);
THE CONSOLE.LOG FUNCTION
Now let’s go for a special function named the console.log function. We have already used the alert function and for now it is ok to use it, however it is pretty annoying for the user to have to click OK or Cancel all the time. Probably you have not seen these dialog boxes many times because well, they are annoying. They are so annoying that most modern browsers nowadays restrict that function and create a special dialog box asking you if you want to prevent the website from creating more dialog boxes. This is because in the past (and some sites still do) created an infinite number of these boxes or at least a lot of them to keep the user busy in the website.
The console.log function works almost the same as the alert, but instead of prompting the result in a dialog box we need to click, it prompts it to the JavaScript console in any modern browser. This console is hidden to the user and can be accessed usually by pressing F12. If you are using a Mac, it can be accessed by pressing Command – Option – I. However you can also look in the options of your browser by searching “developer tools” or “web console”.
Let’s make a simple example
var number = 17;
console.log (" the value of x is " , x ) ;
This program will just prompt the message “the value of x is 17” in the JavaScript console into your browser. You may wonder why should you make a message intended to be hidden from the user? Because users don’t want to see all the messages you want to prompt, and they don’t want to click on OK and Cancel buttons either. The alert function is fine to test, but usually you will use another way to prompt the information to the user and not the alert one, that is quite invasive.
FUNCTIONS RETURNING VALUES AND SIDE EFFECTS
Side effects are the main reason we call functions. For example the side effect of the alert function is to show a dialog box. But not all functions have side effects, some of them produce values and we use them when we want that value. It’s time to introduce you a new function called Math.max. This function needs as many numbers as we want as arguments and produces the greatest of them as an output. When a function gives us a value, it is called to return the value.
console.log (Math.max (2 , 4) ) ;
As we already reviewed in the console.log function, it needs an argument that can be a string, a number, a variable… but also can be another function! In this case it will prompt in the console the return value of the Math.max function that returns the greatest of all the values given between the parentheses and separated by commas. So, if you run this program, it will return a 4.
Just for you to know, there is another function called Math.min that does exactly the opposite. It returns the lesser of all the values given.
MORE POP-UP COMMANDS
Alert is not used much in modern programs because you have no control on how the windows look like, and these windows are usually annoying by the way. However, when it comes to experiment and learning programs, they are a good way to teach so, we will introduce two more functions on creating annoying popups.
The function confirm prompts a message and lets the user answer by a simple OK or Cancel. This function returns a Boolean value. True if user clicked OK and false when the user clicked Cancel. For example:
confirm ("Do you like this tutorial?") ;
This will return True if user clicked OK and False if user clicked Cancel.
The name of the second function is Prompt. It does more or less the same but also lets the user to write whatever he wants in the dialog box (same as the alert function) and even lets us input the starting characters. For example:
prompt ("Why do you like this tutorial? " , "I like it because ") ;
As you can see, this function asks for two arguments, the text to be shown in the dialog box and the text the user will start with. And then it returns the full message as a string.
CONDITIONAL EXECUTION AND CONTROL FLOW
Basically we have seen for now that programs are executed from top to bottom. So, the first functions or commands are executed first and then the next and so on, but it is not always like this.
For example in our previous program:
var temperature = prompt('What is the temperature in Celsius?');
alert((temperature * 1.8) + 32);
What if the user types “potatoes” instead of a number? Well, the program will have nothing to do and will return nothing. This is because JavaScript has the NaN value we already explained. If the value is not a number, it will store NaN instead. This will prevent the program to crash when trying to multiply the word “potatoes”.
So, we can use some conditional execution to warn the user he didn’t give us a number to convert to Fahrenheit.
var temperature = prompt('What is the temperature in Celsius?');
if (! isNaN (temperature) )
alert((temperature * 1.8) + 32);
else
alert (‘Hey you!! Give me a number!!!’);
Welcome to conditional execution. The most common way to do it is to use the word if. After the if, we place a statement between parentheses so if, and only if, the condition is met the following code will be executed. In this case we used the function isNaN. This function checks of the value of a variable is NaN (Not a Number) so, if this is the value inside the temperature value (because the user entered “potatoes” or his favorite expletive). The ! symbol reverts the meaning of isNaN so, we could say that this means “unless temperature is not a number, calculate the Fahrenheit and else let the user know he can’t scam us.
NESTED IFS STRUCTURE
Now you know how to make a conditional execution, but this is pretty simple. Usually there will be more than two choices and then you will need more tools. One of the tools is the nested ifs. To put it simple, a nested if structure is a series of ifs one into another so if a condition matches, it runs something and if not goes to the next if and so on. You can make as many ifs as you want. Don’t worry, you will get tired before your computer crashes.
So, now we want the popup window to return if it is hot, or it is cold or it is nice depending on the temperature. How to do this?
We will reuse the code we wrote before. Reusing code is something every coder does. It saves us the task of having to write it again and also, we already checked it out so, there are no mistakes. There is also a legend saying if you type too much your fingers will rot and your head will explode so, heed my word and reuse as many code as you can.
var temperature = prompt("What is the temperature in Celsius?");
if (! isNaN (temperature) )
if (temperature < 15)
alert("It’s cold!");
else if (temperature < 30)
alert ("It’s nice!");
else
alert ("It’s hot!");
else
alert ("Hey you!! Give me a number!!!");
Now let’s see the flow of the program. First it prompts the annoying popup asking for the temperature in Celsius. It doesn’t calculate the Fahrenheit temperature now and instead it checks first if what the user entered was a number. If he didn’t enter a number it will jump to the message saying that was not a number. In the case it was a number it will first check if the number is below 15. If that’s the case, it will say it’s cold. If not, will jump to the else and another if opens to check if the temperature is below 30. If that’s the case it will say it’s nice. Finally, if it is not less than 30 it must be 30 or over and then it will say it is hot.
INDENTATION
Now you may have noticed there is a series of spaces before some lines. Why I did that? To keep the code tidy and understandable. By using this convention it is easy to see at a first glance that, if the first condition (not to be NaN) is not met, it will jump to the last line of code. This is just for visual purposes and to keep the code tidy and it is not necessary for JavaScript to understand it. Actually, JavaScript doesn’t even need the line breaks and you can write all the program on a single line, but that will look like a mess and so difficult to understand take a look:
var temperature = prompt("What is the temperature in Celsius?"); if (! isNaN (temperature) ) if (temperature < 15) alert("It’s cold!"); else if (temperature < 30) alert ("It’s nice!"); else alert ("It’s hot!"); else alert ("Hey you!! Give me a number!!!");
This will do exactly the same but now you look at it and you have no idea about the flow of the program. And this is just a small piece of code, imagine a code of several thousands lines. If you don’t keep it tidy not even you will be able to know what is going on in your program. To put it simple. Machines don’t need a tidy code but humans do. And you are probably a human so, keep your code as tidy as possible. This is what we call indentation and, even though it is optional for JavaScript, it is mandatory as a good practice in programming.
LOOPS
Loops are another way of conditional execution. A loop basically is a sentence or a series of sentences that is repeated several times, usually until a condition is met. Then, the loop is over and the program will keep running after it. For example we want a program showing the odd numbers from 1 to 10. This could be done like this:
console.log (1) ;
console.log (3) ;
console.log (5) ;
console.log (7) ;
console.log (9) ;
Nice. We did it. But what if I want all the odd numbers up to 10000? Writing all that code will be tedious and you will have to type a lot and remember what the legend says to programmers who type a lot? We don’t want our heads to pop so, we will use a loop instead.
var number = 1;
while (number <= 10000) {
console.log (number) ;
number = number + 2;
}
var arr = [5,7,8];
for (var i=0; i < arr.length; i++) {
console.log(arr[i]);
}
So, let’s check out the flow of this program. First we create a variable and we name it number. Then we initialize it to 1 because it is the first odd number to the long way to 10000. Then the next is the keyword while. This keyword creates a loop and repeats whatever is between curly braces { } as long as the condition between brackets ( ) is met.
So, our number is 1 and then is checked to be less or equal to 10000. Since it is lesser, we prompt the number in the console because we don’t want annoying popups to the user and we add 2 to the number. The curly braces are over so, the flow goes back to the check up again. The number is now 3 and again is lesser or equal to 10000. So again, it is prompted to the console and added 2 more. The loop goes back to the condition check and now the value is 5 so it is printed and added 2… and so on until 9999 which will be the last number to be prompted. If we now check the console we will have all the odd numbers from 1 to 9999 and just with 4 lines of code. We are safe for now.
CONCLUSION
This was a very basic tutorial not only for JavaScript but also for programming. We have already learned a few functions in JavaScript like prompt, alert and console.log. But we also learned the very basics of programming like indentation (to keep your code tidy at all times) and the basic flow of a program. Conditional flow with ifs and nested ifs and loops with a while structure. We have also learned we should not type more code than strictly necessary for our own good and the fact that popups are annoying but good to be used as sample codes for teaching or toying around.
This was meant to be a very basic tutorial on JavaScript but also a guide for those who are learning to code and want to start doing it with good practices. Once you start coding in JavaScript you will find out that coding is not so difficult and even though you may look overwhelmed at first, it is just a matter to get used to the syntax of the programming language you are using (although technically JavaScript is a scripting language).
Recent Stories
Top DiscoverSDK Experts
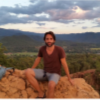
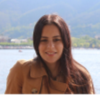
Compare Products
Select up to three two products to compare by clicking on the compare icon () of each product.
{{compareToolModel.Error}}
{{CommentsModel.TotalCount}} Comments
Your Comment