JavaScript Tutorial Part 1
This tutorial is meant for total beginners, even for those who have never learned any other programming languages. Those who are more experienced with programming or with JavaScript may get bored by reading it. It is intended for those who want to learn the basics of JavaScript and not for those who want to learn more about it. It is also a good guide for people who want to start in programming and have never coded before in any other language. Besides teaching some of the basics of JavaScript we will also teach you the basics of programming.
Many of the concepts in JavaScript are common to many other programming or scripting languages so, if you have already coded in C for example you probably know what a loop or a nested if structure is, but since this guide is written for the total beginner we want to also teach these concepts.
So, welcome if you are a total beginner. There is something you need to know before getting started with JavaScript. First thing, it is a programming language and it takes time to learn and master. The most common mistake for beginners learning any kind of programming language is to try to do too much too fast. Programming is learned step by step and you are very far away from creating cool websites with a lot of features.
Pace yourself, give yourself a break and don’t get in a rush to make too many things too fast. It is better to learn the basics very well, even if it takes time to do so, and then move to the next step of the ladder than moving so fast and then get stuck because there is something you don’t understand.
GETTING STARTED
Any modern browser supports JavaScript so, you don’t need anything but the browser and a text editor, for example notepad or Dreamweaver. Dreamweaver is much more specific to web development and includes many tools that will make the task easier but for now, notepad will be enough.
A program is a series of lines of code that are executed sequentially. Or said in another words, one after another. A program can be just one line of code or can take thousands or hundreds of thousands lines of codes.
So, you are up to make your first program. There is a non-written tradition that reads every beginner must make the “Hello World” program as his first creation. If you don’t know what a “Hello World” program is, you are in the right place.
A “Hello World” program is a program that just displays the message “Hello World” in the screen. That’s it. You weren’t thinking about creating a next generation search engine in your first program did you? As said previously, it is better to start with the simplest tasks and get moving in the ladder.
HELLO WORLD
The only thing you need to make this program is a text editor like notepad and any modern web browser.
- Open your notepad and type the following lines of code (don’t worry, we will explain them later).
<!doctype html>
<title>JavaScript Test</title>
<script>
alert('Hello World!');
</script>
- Now save the file as .html
- And open the file!
- Congratulations, you have a blank website displaying the message “Hello World!”
Now, let’s explain a bit this code:
Actually, the only JavaScript line of the 5 you wrote is the 4th one. The rest are HTML codes, necessary for the program to run in a web browser. This alert line makes the browser to display a message box containing whatever you write between the “”. Yes, we said “” instead of ‘’. This is because for JavaScript “ and ‘ are exactly the same and can be used indistinctly. However, it is a good practice to use always the same one to make the code look more beautiful and tidy.
You may have noticed that semicolon in the end of our JavaScript line. It tells the browser the statement ended and it is supposedly optional. Many languages use the semicolon as an end to a statement like C or Java, but in JavaScript this is optional. Usually. And we say usually because sometimes if you leave off the semicolon you will get a problem so, it may sound old school but… get used to finish your statements with a semicolon just in the case.
As a standard good practice when programming, it is good to keep your code as tidy as possible. Sure, this 5 lines program will look tidy nonetheless but when you are making more complex programs, keeping them tidy is essential. To make them tidy you should follow some good practices we will point out in this tutorial as well. For now, remember to use ‘ or “ but not both and if you want to prevent problems, always end the statement with a ;.
USE OF VARIABLES AND GETTING INPUT FROM USERS
Sooner or later, you will want to do something else than displaying messages in the screen. Usually the first thing you want is to get some input from the user. Users like to interact with programs, and not just watch them do things without any interactivity.
So, let’s make a program to tell the user the temperature in Fahrenheit when the user inputs the temperature in Celsius. This will involve creating variables and making some simple calculations. Remember to modify the program between the <script> and </script> because the rest of the lines are used to let the browser run the program. Sort of.
Let me introduce you the concept of variable. A variable is like a box where you store some data to be used later. Sometimes, a lot of times actually, you will need to use these boxes to store some content on them and use it later to perform calculations, display it or many other uses.
First, we will use the command prompt and we will put its value into a variable. One of the many good things of JavaScript is it doesn’t need to initialize and define variables. You can just create them on the fly while you are using them. For example, you can make this line:
var temperature = prompt('What is the temperature in Celsius?');
Prompt will show a box for the user to input the temperature and will store it into the variable named, not surprisingly, temperature. It will also display the message What is the temperature in Celsius? By doing so, we have stored the value entered in a box named temperature.
But if we store a value in a variable but we don’t do anything else with it, it is pretty much useless. So, let’s add this line of code just below the previous one:
alert((temperature * 1.8) + 32);
By doing this, the content in the variable temperature (whatever the user has entered) will be multiplied by 1.8 and then add 32 to the result, displaying it in the box.
Here you are, you have made a simple Celsius to Fahrenheit calculator in a few lines of code.
The same can be done as well with characters or, as we like to call them, strings. Strings actually are a series of characters. If we spell the word “Hello” a string will be composed of the characters H + e + l + l + o and will be put all together. It may look strange for those who have never programmed, but maths work for characters of words the same as for numbers.
In the following program we will greet the user, depending on the name he has entered. First we need to create a dialog box to ask for the name of the user and later, we will greet him. Now the line should look like:
var name = prompt('What is your name?');
Now our box will be full of letters with the name the user has entered. And after that, we want to greet him:
alert('Hello ' + name + ', nice to meet you!');
Now a bit of syntax. Whatever we want to display and is not a variable, must be between ‘ ‘ or “ “, but the variables are displayed directly so no need to use the “ “ or ‘ ‘. Also, notice the space after the Hello word. If you don’t put a space there, the name of the user will appear just next to the Hello word without any space between it.
So, this is your first greeting program and for now you know how to create variables, operate with them and you know in the variables you can put numeric or alphabetic values. There are other values you can store into variables, but more about that later.
CREATING AND USING BUTTONS
One of the main uses of a website is to create buttons. Users love to press buttons and besides that, they are useful to let us know what the user wants without having to ask for input all the time. Users prefer to press (or click) buttons rather than typing because users, as well as coders and most humans, are lazy.
You have noticed, in many websites you have buttons. And buttons may look like a button or they may look as something completely different, but the whole point is you can click on them and the website will do something. For now we will stick to classic buttons and we will make a very simple program with them.
This program will have two buttons, one will change the background color of the website and the other one will reset it to the default color (white).
This is the code (don’t panic, we are going to explain it carefully after it).
<!doctype>
<script>
var oldColor = 'black';
function changeColor() {
oldColor = document.body.style.backgroundColor;
var newColor = prompt('What color would you like?');
document.body.style.backgroundColor = newColor;
}
function undoColor() {
var newColor = oldColor;
oldColor = document.body.style.backgroundColor;
document.body.style.backgroundColor = newColor;
}
</script>
<button onclick='changeColor()'>Change color</button>
<button onclick='undoColor()'>Undo color</button>
The first line just defines a variable, named oldcolor and stores the value black on it. This is done because later we are going to use it, and we want it to have a value.
var oldColor = 'black';
This piece of code is a bit trickier. Then, locate the final lines of it:
<button onclick='changeColor()'>Change color</button>
<button onclick='undoColor()'>Undo color</button>
This creates the buttons and the onclick means the button will act when the button is clicked. Actually what it really does is what we call “calling a function” functions are small programs themselves. They perform something for us as a part of the main program. And the functions are these 2:
function changeColor() {
oldColor = document.body.style.backgroundColor;
var newColor = prompt('What color would you like?');
document.body.style.backgroundColor = newColor;
}
function undoColor() {
var newColor = oldColor;
oldColor = document.body.style.backgroundColor;
document.body.style.backgroundColor = newColor;
}
Now, let’s analyze more the above code.
This just defines the function we already defined in the button:
<button onclick='changeColor()'>Change color</button>
So, when the button is clicked the function changecolor will run.
This changes the value to the variable variable, like the ones you have used before and then stores the actual color of the website in it. JavaScript is quite cool and by using document.body.style.backgroundColor it knows exactly the background color of the website.
So, the next line of the code reads:
var newColor = prompt('What color would you like?');
This also creates a new variable and stores whatever the user inputs on it.
document.body.style.backgroundColor = newColor;
This line does exactly the opposite as the line
oldColor = document.body.style.backgroundColor;
Instead of storing the actual background color inside a variable, it stores the value of the variable into the background color of the website. By doing this, the background color will be changed to whatever color the user typed. JavaScript is really nice and recognizes most of the colors without you having to do anything else. However, if the user doesn’t input a color and inputs, let’s say, “potatoes” the function will do nothing. But won’t report an error! This is quite great for programmers, because we don’t like errors.
Now, we want to see what the undocolor function does.
The value of the newColor variable is changed to the previous one used. Remember we initialized the variable oldColor before? This is because if not, there will be no old color now.
This stores the actual background color of the website as oldColor. So, if the user wants to get back to this color, it is already stored!
And this line is the same as in the previous function. It stores the value of the variable into the background color of the website.
With this, you have learned to create buttons and what can be done with them.
TIME FOR A BIT OF THEORY ABOUT VARIABLES
There are a few things you must know in order to program well with JavaScript. First thing is the reserved words list. Reserved words are special words used by the environment and you should not use them unless you are stating them into ‘ ‘. So, the name of a variable or a function should never have this name because it could produce errors. The reserved word list in JavaScript is a bit long so, don’t try to memorize it but bear it in mind and keep this as a reference for the future.
List of Reserved Words in JavaScript
break case catch class const continue debugger default delete do else enum export extends false finally for function if implements import in instanceof interface let new null package private protected public return static super switch this throw true try typeof var void while with yield.
Another thing, while you can put almost any name to variables, you may not use . or , or ‘ or any other special symbol. Actually some special symbols are allowed like _ but as a rule of thumb, the less special symbols you use, the better as it may lead to errors in the future.
What if a variable hasn’t got a good value? For example when we are talking about numbers, there are 3 special numbers in JavaScript. Infinity and -Infinity are the first two. These represent the two infinities, positive and negative. We do not recommend to use them a lot, because infinity is an odd number that will usually lead to the third special number, called NaN.
NaN means actually “not a number” but it is treated as a number. This is because in order to avoid crashes in the system, when a mathematical operation does not get a number like for example dividing something into zero, the variable has still to have a value or it will crash. Then it gets NaN.
More types of variables
We already worked with characters or strings and numbers but there are more types like for example the Boolean type. Booleans can only get two results. True or False. These are used for logical calculations like for example:
3 > 2
This expression is true so, if linked to a variable or output to the screen it will show TRUE while this other:
3 < 2
Will return FALSE.
more examples:
var m=200;
var s1=’val’;
var s2=”var”;
var b1=true;
And if we want to declare an object:
var ob1 = {};
ob1.prop1 = 100;
ob1.prop2 = "great";
console.log(ob1.prop1 + " " + ob1.prop2);
continue to Part 2
Recent Stories
Top DiscoverSDK Experts
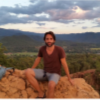
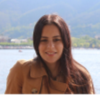
Compare Products
Select up to three two products to compare by clicking on the compare icon () of each product.
{{compareToolModel.Error}}
{{CommentsModel.TotalCount}} Comments
Your Comment