Case Study - Guess The Number
In this article I will give you an overview of and quick introduction to the Python 3 programming language through a simple game example. This will not be a thorough or comprehensive examination of Python—that wouldn’t fit in a simple article. Here, I just plan to give you a taste of Python 3 and if you like what you see you can dig deeper.
The example code will be the following:
__author__ = 'GHajba'
import random
def get_max_number():
while True:
try:
max_number = int(input('Should the secret number between "1 and 100" or "1 and 1000"? (Enter "100" or "1000") '))
except ValueError:
print("This was not a number!")
continue
if max_number != 100 and max_number != 1000:
continue
return max_number
while True:
max_number = get_max_number()
if max_number == 100:
guess_count = 7
else:
guess_count = 10
print('You have chosen {}, you will have {} guesses to find the secret number.'.format(max_number, guess_count))
secret_number = random.randint(1, max_number)
print('I have chosen the secret number...')
guesses = 0
while guess_count - guesses:
try:
guesses += 1
guessed = int(input("What's your guess? "))
except ValueError:
continue
if guessed == secret_number:
print('Congrats, you have Won!')
break
elif guessed > secret_number:
print('The secret number is lower...')
else:
print('The secret number is higher...')
else:
print("Sorry, you lose.")
print("The secret number was ", secret_number)
answer = ''
while answer.lower() not in ['yes', 'no', 'y', 'n']:
answer = input("Do you want to play another round? (yes / no) ")
if 'no' == answer or 'n' == answer:
break
As you can see, this is a simple "Guess the Number" game where the application thinks of a number and you have to guess it.
Now let’s dig into deeper and see what each code block does.
__author__ = 'GHajba'
This is a simple documentation text which discloses the author of the script. In this case GHajba has written the script. The apostrophes (') around the name indicate that it is a string.
import random
This line imports the random module of Python. Modules enable you to break down big codebases into smaller chunks and store them in separate files. Every Python file containing Python source code is a module too.
In this case random is a general module shipped with your Python distribution and is always available for use and you do not need to specify where it is because Python knows its libraries.
The random module will be later used to generate random numbers for the game.
def get_max_number():
This line starts a function definition, it is indicated by the keyword def. This function does not have any arguments but other function definitions may have arguments passed to the function between the brackets.
The body of the function is the intended block after the definition line.
Functions in Python always return a value however you do not need to explicitly write a return statement. If you do not specify something with return Python automatically returns the None object.
while True:
while represents a loop which continues until its expression evaluates to the boolean True value. In the case of the example the expression is always True meaning that this loop will never terminate from itself (this is called an infinite-loop) but we take care of the termination. In the function get_max_number the loop terminates when we call return. In the main script block it terminates when we call break when the user answers "no" to the question if he/she wants to play more.
With continue we ignore the following lines in the loop's body and jump back to evaluate the termination expression. In some cases you won't break but silently skip processing any further.
input
With input you can ask your user to enter something to your application. As you might guess input is a function and as such it returns a value which is a string—even if the user enters a number like 100. Since input is a function you can provide arguments to it, but you do not have to. What you provide as an argument will be displayed for the user before the prompt sign where he/she can enter something. So I would always add an argument when using input or your user will wait forever.
Another use case for this function is the familiar "Press any key to continue..." text. In this case you can prompt the user to press any key on the keyboard but you do not use the return value.
int
As I mentioned in the previous section input returns a string. However we need an integer value. For Python this request is natural and it provides a function called int which gets a string parameter and converts it to a numeric value we can use further for calculations. If the provided argument cannot be converted to a numeric value the function raises a ValueError.
try + except
Error handling is always a core duty of programmers. In Python you can achieve it through the try and except keywords. As their name already suggests you try to execute a block of code and except if something erroneous happens.
In the example we expect that a ValueError may occur if the user enters something that cannot be converted to an integer. And if this happens, we handle that case with the next code block. If other exceptions occur in the try block we do not handle it and it will eventually stop the application from running. I say eventually because it can happen that a caller of our code may catch other exception. In the example script however no other exceptions are caught so the application will terminate if something else occurs.
You do not have to provide an error type for the except keyword:
try:
some code block
except:
exception handling block
In the example above any error is handled in the except block.
if and else
Sometimes you want to do something based on a specific condition. For this you can use the if-else construct in most high level languages and in Python too. As in other cases these keywords execute the block of code after them—and this block has to be indented.
if takes a boolean expression as a parameter and executes the indented block below itself if this expression evaluates to True. If this expression evaluates to False and there is an else block after the if statement the contents of that else block are executed.
You can use an if block without an else but for an else you have to have an if. If you have multiple if blocks and one else at the end that else will belong to the if prior to it.
elif is the in-between conditional you can use if the first if is not True but you need another condition to check before applying some other code block.
An application is in that case worth using if it gives the user some output of the progress. To have something written to the console in Python 3 you have to use the print function which takes an infinite amount of parameters and displays their string representation on the console separated by a whitespace character and at the end it adds a newline.
If you do not provide any parameters you get only a new line.
None
None is a special type in Python which represents "nothing". As mentioned earlier it is returned from functions implicitly. None behaves like null in Java: you can verify if one variable holds this value but if you try to use methods on it you get an error.
Tabs and spaces
As you could see in the example and the detailed description, Python is written in code blocks. Unlike many higher level programming languages (like C++ or Java for example) Python does not uses brackets to distinguish between code blocks, improving readability.
However you have to take care not to mix tabs and spaces for indenting your Python script. If you do this, you will encounter a runtime exception:
TabError: inconsistent use of tabs and spaces in indentation
I won't tell you which one to use: tabs or spaces. There are some developer "wars" raging in the Internet and in some companies. I can only give you a suggestion: use one and stick to it. If you want to use spaces make sure your development environment converts tabs to spaces.
Want to play?
If you want to try out this simple application just save the script into a file named guess_the_number.py and open up a terminal / command line, navigate to the folder where you created the script file and enter the following:
python guess_the_number.py
Note that you have to install and use Python 3.
Recent Stories
Top DiscoverSDK Experts
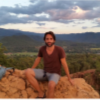
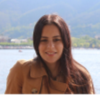
Compare Products
Select up to three two products to compare by clicking on the compare icon () of each product.
{{compareToolModel.Error}}
{{CommentsModel.TotalCount}} Comments
Your Comment