Working With Mutator Array Methods in JavaScript
An array is a mutable data structure unlike a string. Some methods on the array objects mutate the array objects and some methods on the array do not mutate the array objects. Instead, they return new array objects or values of other data types. The methods that mutate the array objects on which it is being invoked are called the mutator methods. In this article we are going to discuss these methods. A future article will look at methods that do not mutate the array on which the methods are being invoked.
Getting Started
To get started with the examples shown in this article you need to have NodeJs installed. You can run the code in browsers as well, but this is not recommended. In the first article of the JavaScript series I discussed why to use NodeJs to learn and practice JavaScript instead of using a browser.
Create a file called arr_mutator.js in your preferred working directory. Point your command line to this directory and execute the script by the command node arr_mutator.js to check if everything is alright.
Sorting With sort()
Sorting an array is a mutable operation. Sorting an array rearranges the positions of elements in the array. Let's say that we have an array of numbers that is not in proper order.
var arr = [6, 66, 7, 88, 0, 1, 12, 3];
arr.sort();
console.log(arr);
Outputs:
[ 0, 1, 12, 3, 6, 66, 7, 88 ]
So, the sort method sorts the array from small to large numbers. But what if we want to get the numbers sorted from large to small? We need a comparing function passed to the sort() method for that. The comparing function will be passed two values. If the function returns 0 both the values will be considered equal, if it returns a positive number the first value will be considered bigger, if it returns a negative number then the second value will be considered bigger. For numbers it is enough to use firstArgument - secondArgument for ascending sort and firstArgument - secondArgument for descending sort.
var arr = [6, 66, 7, 88, 0, 1, 12, 3];
arr.sort(function(x, y){
return y - x;
});
console.log(arr);
Now, it outputs:
[ 88, 66, 12, 7, 6, 3, 1, 0 ]
You can create custom comparing functions for other types of values or objects.
Making Everyone Even With fill()
If you want the whole array to be replaced with a single value and you are to lazy to run a loop over them to do so, you use the fill() method on it. This method does not extend the array but rather replaces all existing values with the one you provide as the parameter.
var arr = [6, 66, 7, 88, 0, 1, 12, 3];
arr.fill("a string");
console.log(arr);
The above block of code outputs the following result.
[ 'a string',
'a string',
'a string',
'a string',
'a string',
'a string',
'a string',
'a string' ]
Reversing the Order With reverse()
The reverse() method reverses the arrangement of the elements inside of an array. The first element becomes the last and the last element becomes the first. Let's sort an array with the sort method, with no comparing function. In this way we will get an array sorted in the ascending order. When I already have an array in ascending order I do not want to re-sort it in descending order. Sorting is a costly operation. So, we are just reversing the array with reverse() method.
var arr = [6, 66, 7, 88, 0, 1, 12, 3];
arr.sort();
arr.reverse();
console.log(arr);
Outputs:
[ 88, 7, 66, 6, 3, 12, 1, 0 ]
Popping an Element Out of the Array With pop()
If you want to remove an element from the end of an array you need to use the pop() method. This method not only removes an element from the end of an element, it also returns the removed element.
var arr = [1, 2, 3, "Four"];
var lastElem = arr.pop();
console.log("The value of the removed element is: " + lastElem);
console.log("The current array is: " + arr);
Output:
The value of the removed element is: Four
The current array is: 1,2,3
Removing the First Element From The Start of an Array With shift()
Like pop(), shift() removes an element from an array. But, it removes the first element. It also returns the removed element.
var arr = [1, 2, 3, "Four"];
var firstElem = arr.shift();
console.log("The value of the removed element is: " + firstElem);
console.log("The current array is: " + arr);
Outputs:
The value of the removed element is: 1
The current array is: 2,3,Four
Adding an Element at the End of an Array With push()
The push() method is used to add an element to the end of an array. Let's try this with an example.
var arr = [1, "two", 3];
arr.push("Four");
console.log(arr);
Outputs:
[ 1, 'two', 3, 'Four' ]
Adding an Element at the Start of an Array With unshift()
To add an element at the start of an array you need to use unshift() with the element as the parameter you need to add.
var arr = [1, "two", 3];
arr.unshift("Zero");
console.log(arr);
Outputs:
[ 'Zero', 1, 'two', 3 ]
Inserting Elements Into an Array
push() appends elements at the end of array, unshift() adds at the beginning. But, what if we need to insert element at the middle of arrays. We have splice() for that. Splice can be used for adding elements at any position of array. It can be used to remove them from any position also.
var arr = [1, "two", 3];
arr.splice(2, 0, "two 2");
console.log(arr);
Output:
[ 1, 'two', 'two 2', 3 ]
The first parameter refers to the index at which you want to insert an element, the second parameter tells not to remove the element at that position by specifying it as 0, and the third element specifies the element you want to add.
Removing Elements From Any Position of an Array
pop() is used to remove an element from the end of an array and shift() is used to remove from the start of an array. To remove elements from any position of an array, including the start and the end, you can use splice(). Put the index as the first parameter to splice() that you want to remove and as the second parameter specify how many elements you want remove.
var arr = [1, "two", "two 2", 3];
arr.splice(2, 1);
console.log(arr);
Outputs:
[ 1, 'two', 3 ]
As the second argument we told the splice() method to remove only one element.
Conclusions
In this article I gave you a demonstration of different array mutator methods. In a future article I will try to cover non-mutating methods. You are advised to continue practicing all the mutator methods before moving on to anything else.
Recent Stories
Top DiscoverSDK Experts
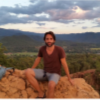
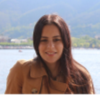
Compare Products
Select up to three two products to compare by clicking on the compare icon () of each product.
{{compareToolModel.Error}}
{{CommentsModel.TotalCount}} Comments
Your Comment