Types & Variables in TypeScript
In the first article in our TypeScript series, we provided an introduction and overview of this increasingly popular language. Today, we’re going to dive into making use of the language and take our first steps by examining types and variables in TypeScript. Let’s get into it!
TypeScript Types
In TypeScript, the type system is the various types of values that the language supports. The type system checks to see if a value is valid before it is stored or used in any way in the program. This is to ensure that all the code will run as expected. TypeScript’s type system allows for excellent code auto-completion and automated documentation.
The type system is based on structural subtyping. That means that a type is defined by the collection of properties and their types, rather than just the type’s name. This is perhaps a bit too much information so don’t worry if you didn’t exactly catch that.
Built-in Types in TypeScript
Here are the built-in types in TypeScript:
boolean |
Double precision 64-bit floating point values. Can be used to represent integers or fractions |
number |
Represents a sequence of Unicode characters |
string |
Represents logical values: true and false |
void |
Used with function return types to represent a non-returning function |
null |
The intentional absence of an object value |
undefined |
The value given to any uninitialized variable |
These should all be familiar to you—no big surprises here. Did you notice something missing though? Take note that there is no integer type in TypeScript.
The Any Type in TypeScript
There is a data type in TypeScript called any. This is used to describe the type of a variable when you don’t know what it will be when writing the program. These values will probably come from some kind of dynamic content like the user or an outside library. Using the any type lets you opt out of type checking in a particular variable.
It looks something like this:
let unKnown: any = 100;
unKnown = "could be a string";
unKnown = false; // turns out it’s a boolean
Any is also useful if you only know part of the type. For example, if you have a mixed array like so:
let list: any[] = [1, false, "a string"];
list[1] = 100;
Null vs Undefined
Many are often confused by the difference between null and undefined in TypeScript. What they have in common is that they cannot be used to reference the type of a variable and can only be assigned as a value to a variable.
Their difference is that when a variable is initialized with undefined it doesn’t have a value or object assigned to it. However, with null it means the variable has been set to an object with a value that is undefined.
User Defined Types in TypeScript
These include Enums, classes, interfaces, arrays, and tuple. We’ll actually examine these in a future article.
TypeScript Variables
Variables are defined as a named space in the memory that stores a value. This means that variables act as a container for values in a program. In TypeScript, variables follow the same naming conventions as in JavaScript:
- Variable names are made up of letters and digits
- They may not contain spaces or special characters, except the underscore (_) and the dollar ($) sign
- Variable names may not begin with a digit
Variables must be declared before they are used. We use the keyword var in order to declare a variable. The syntax for setting a variable type in TypeScript is the use of a colon after the variable name, and then the type.
There are four ways to declare a variable in TypeScript. Let’s examine them:
Declare the value and type in one statement:
var [identifier] : [type-annotation] = value ;
E.g. var name:string = ”Arjun”
Declare the type but not the value, which will be set to undefined:
var [identifier] : [type-annotation] ;
E.g. var name:string;
Declare the value but not the type, which will be set to the data type of the assigned value:
var [identifier] = value ;
E.g. var name = ”Arjun”
Declare neither the value nor the type. Here, the type will be set to any and initialized to undefined:
var [identifier] ;
E.g. var name;
Let’s see a few more examples so you can get a clearer picture. Here is some TypeScript code:
var name:string = "Arjun";
var score1:number = 60;
var score2:number = 45.50
var sum = score1 + score2
console.log("name: "+name)
console.log("first score: "+score1)
console.log("second score: "+score2)
console.log("sum of the scores: "+sum)
This code will generate the following JavaScript code:
var name = "Arjun";
var score1 = 60;
var score2 = 45.50;
var sum = score1 + score2;
console.log("name: " + name);
console.log("first score: " + score1);
console.log("second score: " + score2);
console.log("sum of the scores: " + sum);
This in turn will give us the following output:
name: Arjun
first score: 60
second score: 45.5
sum of the scores: 105.5
If you try to assign a value to a variable that is not of the same type, TypeScript’s compiler will give you an error. This makes TypeScript a strongly typed language. This makes it so that the types that are specified on either side of the assignment operator i.e. the equals sign (=) are the same. As such code like that in the example to follow will result in a compilation error:
var num:number = "Oops!" // This will return a compilation error
Type Assertion
In TypeScript, what is known as type assertion is more or less what you may be familiar with as type casting in other languages such as Java. The simplest way to think about it is changing a variable from one type to another. It’s used in cases where the programmer knows more about what the type should be than TypeScript does.
The syntax is pretty simple—just put the target type in side the greater than/less than symbols < > and put it in front of the variable or expression. Have a look at this TypeScript code example:
var str = '200'
var str2:number = <number> <any> str //str is now of the type ‘number’
console.log(str2)
Here is the JavaScript that it compiles to:
var str = '200';
var str2 = str; //str is now of the type ‘number’
console.log(str2);
And finally, the result printed in the console:
200
A quick note: The reason that it’s not called type casting as in other languages is that it has no runtime impact. Type assertions in TypeScript are strictly used by the compiler.
Inferred Typing
Since TypeScript is strongly typed, this is an optional feature. In TypeScript, you can also employ the dynamic typing of variables meaning you can declare a variable without a type. In cases such as this, the compiler with take it upon itself to determine the variable type based on the value assigned to it. It will find the first time that it’s used in the code, determine the type that it was initially set to, and then use this same type for the variable in the remainder of the code block.
Here’s an example to clarify:
var num = 55; // data type inferred as a number
console.log("value of num "+num);
num = "123";
console.log(num);
In the code above, we declare a variable and set it to a value of 2. Notice that the type is not set. This means the program is inferring the type of the variable, in this case num is set to ‘number.’ Then, when the code tries to set the variable to ‘string’ we get the following error:
main.ts(3,1): error TS2322: Type '"123"' is not assignable to type 'number'.
Variable Scope
A variable’s scope specifies where it has been defined and determines that variable’s availability. There are three types of scope that TypeScript variables fall into:
- Global Scope — Global variables are declared outside the programming constructs and can be accessed from anywhere in the code.
- Class Scope — Aka fields, these variables are declared within the class but outside of the method. They may be accessed via the class’s object. Fields can also be static and static fields can be accessed using the class’s name.
- Local Scope — Local variables are declared within the constructs of methods, loops, etc. Local variables are accessible only within the construct where they are declared.
Here’s an example to clarify:
var global_num = 22 //a global variable
class Numbers {
num_val = 333; //a class variable
static sval = 4444; //a static field
storeNum():void {
var local_num = 1; //a local variable
}
}
console.log("Global num: "+global_num)
console.log(Numbers.sval) //a static variable
var obj = new Numbers();
console.log("Global num: "+obj.num_val)
Here is the compiled JavaScript:
var global_num = 22; //a global variable
var Numbers = /** @class */ (function () {
function Numbers() {
this.num_val = 333; //a class variable
}
Numbers.prototype.storeNum = function () {
var local_num = 1; //a local variable
};
Numbers.sval = 4444; //a static field
return Numbers;
}());
console.log("Global num: " + global_num);
console.log(Numbers.sval); //a static variable
var obj = new Numbers();
console.log("Global num: " + obj.num_val);
And finally the output to the console:
Global num: 22
4444
Global num: 333
That’s all for this time. Check back soon for the next article in the series where we’ll have a look at operators in TypeScript.
Recent Stories
Top DiscoverSDK Experts
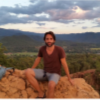
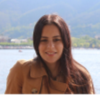
Compare Products
Select up to three two products to compare by clicking on the compare icon () of each product.
{{compareToolModel.Error}}
{{CommentsModel.TotalCount}} Comments
Your Comment