The Reducer Function in JavaScript
The reducer function in JavaScript is hardly advanced material—it’s quite basic. But I was surprised recently to discover that there are many JavaScript programmers, even experienced ones, who aren’t really familiar with it. In general, this method is found in arrays and allows us to convert an array to string, number, or object. That’s right, if you want to convert an array to an object, this is the method for you.
Let’s start with a boring example straight from the MDN. A reducer method that’s alone in an array, allows us to pass a function with four arguments:
- The value received from running the reducer function on the previous member (if there is one)
- The current member
- The index
- The array with the reducer
The function goes over every member of the array. We can return a value that will pass to the argument and that will be passed to the next function in the queue (which will run on the next member).
It sounds a little complicated, so let’s look at an example of a function that brings together all the members to one long text string:
function reducerFunction(lastResult, currentValue) {
return lastResult + currentValue;
}
const myArray = ['avi', 'itzhak', 'yaki'];
const reducedValue = myArray.reduce(reducerFunction);
console.log(reducedValue); // aviitzhakyaki
What’s going on here? First, we’re defining the reducer function. What does it do? It takes the last result (lastResult) which is also called the accumulator. Then it adds to it the value of the first member.
During the first iteration, there is no lastResult, so the reducer returns the array’s current value (the first), avi.
During the second iteration, avi is the lastResult, so the reducer connects it to the value of the first member (the second) which in this case is itzhak. So it returns aviitzhak.
On the third time around, the lastresult is aviitzhak, which is what was returned last time. The reducer takes it and connects it to the current member’s value (the third) giving us aviitzhakyaki.
Whatever the last iteration returns is what the reducer returns.
We can set the value of lastResult even on the first iteration, if we pass an additional argument. Here a continuation of the previous example. This time I’m passing a second argument in the reduce function:
function reducerFunction(lastResult, currentValue) {
return `${lastResult} ${currentValue}`;
}
const myArray = ['avi', 'itzhak', 'yaki'];
const reducedValue = myArray.reduce(reducerFunction, 'Names:');
console.log(reducedValue); // Names: avi itzhak yaki
And what’s going on here?
In the first iteration, it appears we have no lastResult. But, we used the second argument when we called myArray.reduce. So the reducer takes that value, put it into a text string together with the value of the first member (avi), and returns a result of Names: avi.
In the second iteration, lastResult is Names: avi. The reducer takes that value and puts it into a text string with the value of the second member (itzhak) and so on.
Once you start to understand the reducer function in JavaScript, you realize just how powerful it can be. We can use it for all kinds of things. For instance, imagine I get an array with data from the server that looks like this:
function reducerFunction(accumulator, currentValue) {
accumulator[currentValue.name] = currentValue;
return accumulator;
}
const mySites = [{ name: 'Google', url: 'google.com' },
{ name: 'Internet Israel', url: 'internet-israel.com' },
{ name: 'Haaretz', url: 'haaretz.co.il' },
{ name: 'GOV IL', url: 'gov.il' },];
const reducedValue = mySites.reduce(reducerFunction, {});
console.log(reducedValue);
This allows me to convert the array to an object. Notice that I’m setting it so that the first object is empty by passing it as a second argument when we execute reduce on the array.
It’s a pretty useful function. So anytime you need to convert an array, remember to consider using the reducer function. It’s definitely something worth knowing about.
About the author: Ran Bar-Zik is an experienced web developer whose personal blog, Internet Israel, features articles and guides on Node.js, MongoDB, Git, SASS, jQuery, HTML 5, MySQL, and more. Translation of the original article by Aaron Raizen.
Recent Stories
Top DiscoverSDK Experts
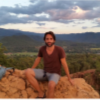
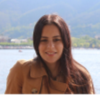
Compare Products
Select up to three two products to compare by clicking on the compare icon () of each product.
{{compareToolModel.Error}}
{{CommentsModel.TotalCount}} Comments
Your Comment