String Manipulation In JavaScript With Node.js
Manipulating strings is a daily chore for a web programmers. No matter whether you develop frontend web application or backend web application in JavaScript, string manipulation is one of the most common tasks. In a previous article I taught you how to start programming with JavaScript. I also have shown you the benefits of practicing JavaScript with Node.js instead of with browsers. In this article I’ll cover various string manipulation methods and functions.
Getting Started
You can practice this code the with browser dev console. But as we discussed before, Node.js is the recommended way. To get started with today's coding you need to have Node.js installed on your system. Make sure that the node command/binary is available in your system's path. Create an empty file called js_strings.js in your preferred working directory. Start your command line in the directory where the newly created file lives.
You can use any plain text editor to edit code or you can use any code editor or IDE if you like. I am going to use Sublime Text for writing code for this article.
Creating String
In JavaScript we can create string by enclosing characters inside double quotation or single quotation marks. In JavaScript there is no existence of character type. If you want to represent a character, you will have to create a string with single character inside it. A string created by putting characters inside quotation marks is called literal string.
A lot of functions or methods in JavaScript return string as a result. The string manipulation methods also return new string. These are not literal strings.
var str1 = "I am string inside double quotation marks.";
var str2 = 'I am a string inside single quotation marks';
String Escaping
We cannot put every type of characters inside those quotation marks to create strings with the help of our regular keyboard keys. Whenever we need to put some special characters inside those quotation marks we need to use a mechanism called escaping. Every escaped characters is created by preceding one or more regular characters with a backslash character. Escaping is also necessary when we need to put quotation marks inside the literal string (this does not apply to strings created dynamically).
Let's say we want to put a newline inside of our string. We cannot just do this just by pressing the Enter key on the keyboard. That will just create a newline inside the js plain text file and break the syntax of the string. To create a newline character we need to precede the n character with a backslash: \n
var str3 = "This is the first line.\nThis is the second line.";
Now, if you want to put a double quotation mark inside a double quoted string you need to escape that double quotation mark with a backslash. So, it will become:
\"
var srt4 = "She said, \"Shut up!\"";
Length Of A String
The length of a string is the number of characters a string contains. The length attribute on a string tells us the length of that string.
var str = "This is a sample string";
console.log(str.length);
Outputs:
23
Case Conversion
It is very easy to transform the cases of characters of a string. To transform a string to lowercase invoke toLowerCase() on the string object.
var str = "Once upon a time there was a king called The STRING KING";
var strL = str.toLowerCase();
console.log(strL);
Outputs:
once upon a time there was a king called the string king
Notice, every character in the string has been transformed to lowercase characters.
To transform a string into all uppercase invoke the toUpperCase() method on the string object.
var str = "Once upon a time there was a king called The STRING KING";
var strU = str.toUpperCase();
console.log(strU);
Outputs:
ONCE UPON A TIME THERE WAS A KING CALLED THE STRING KING
Searching
Searching for a substring inside a target string is a very common operation in JavaScript programming. Let's say we want to search for 'cure' inside the string 'Scientiests are trying to find cure for cancer for a long time, but still no luck!'. We invoke the search() method on the string to search for a sub-string in it. If this method is successful in finding the substring in the target, it will return zero or a positive number. This number indicates the starting index of the substring in the target string. It returns -1 if it does not find any match.
var target = "Scientiests are trying to find cure for cancer for a long time, but still no luck!";
var sub = "cure";
if (target.search(sub) == -1){
console.log("`cure` was not found!");
}else{
console.log("`cure` found!!");
Outputs:
`cure` found!!
Stripping
To remove unwanted whitespace characters from the left and right side of a string we can use the trim() method on it. Let's say you want to remove all the white spaces from the left and right side of a string.
var str = " JavaScript ";
var strStripped = str.trim();
console.log("Original string: " + str);
console.log("Stripped string: " + strStripped);
Outputs:
Original string: JavaScript
Stripped string: JavaScript
Replacing
To replace a substring from a target string invoke the replace() method on it with two arguments passed to it. The first one is the sub-string that you want to be replaced and the second one is the string that will be used in place of the first one.
var str1 = "You are a bad boy.";
var strR = str1.replace("bad", "good");
console.log("Original string: " + str1);
console.log("Stripped string: " + strR);
Outputs:
Original string: You are a bad boy.
Stripped string: You are a good boy.
Splitting
We often need to split string to individual parts. We have the split() method for that. It accepts another string with which yor to split the string. It will return an array of strings. In the example below, we pass in a black space:
var str1 = "You are a bad boy";
var words = str1.split(" ");
console.log(words);
Outputs:
[ 'You', 'are', 'a', 'bad', 'boy' ]
startsWith(), endsWith()
We often need to check whether a string starts with something or ends with something. Let's check whether a string starts with https:// or not.
var url = "http://sabuj.me";
if (url.startsWith("https://")){
console.log("The url is secure");
}else{
console.log("The url is not secure")
}
Outputs:
The url is not secure
Let's see an example on endsWith():
var url = "https://sabuj.me/";
if (url.endsWith("/")){
console.log("The url ends with a forward slash");
}else{
console.log("Forward slash is not at the end of the url")
}
Outputs:
The url ends with a forward slash
Conclusion
The life of a JavaScript programmer is impossible without the knowledge of string manipulation and various methods used. Practice these string manipulation methods as much as possible until you reach the level of perfection. JavaScript did not support unicode to it's full extent, however since ES6 there is much improvement in unicode in JavaScript. I suggest learning to work with unicode in JavaScript. Also, learn regular expression in JavaScript to get the full potential and power of strings.
Stop by the homepage to search for and discovery tons of JavaScript Livraries and SDKs.
Recent Stories
Top DiscoverSDK Experts
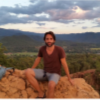
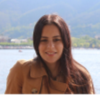
Compare Products
Select up to three two products to compare by clicking on the compare icon () of each product.
{{compareToolModel.Error}}
{{CommentsModel.TotalCount}} Comments
Your Comment