React Native - Styling Components
In previous lesson, we saw how to build basic React components like Image and Text. Another skill to master is styling components, without which few apps can go very far.
Declaring and Modifying Styles
In React for the Web, we usually make use of modular stylesheet files, which can be written in CSS, SASS, or LESS. React Native takes a completely different path, taking styles entirely into the world of JavaScript and forcing us to link style objects explicitly to components.
Instead of stylesheets, in React Native we work with JavaScript-based style objects. One of React’s greatest strengths is that it forces us to keep our JavaScript code (our components) modular. By bringing styles into the realm of JavaScript, React Native also pushes us to write modular styles.
Inline Styles
Inline styles are the simplest way (in CSS as well), syntactically, to style a component in React Native, though they are not usually the best way. The syntax for inline styles in React Native is the same as for React for the browser. Let’s quickly look at an example:
<Text>
The quick <Text style={{fontStyle: "italic"}}>brown</Text> fox
jumped over the lazy <Text style={{fontWeight: "bold"}}>dog</Text>.
</Text>
Inline styles have some advantages. They’re quick and dirty, allowing us to rapidly experiment.
Though they look pretty straightforward, inline styles should be avoided as much.
Styling with Objects
If we take a look at the inline style syntax, we will notice that it’s simply passing an object to the style attribute. There’s no need to make the style object in the render call though; instead, we can separate it out, as shown in following code snippet:
var italic = {
fontStyle: 'italic'
};
var bold = {
fontWeight: 'bold'
};
...
render() {
return (
<Text>
The quick <Text style={italic}>brown</Text> fox
jumped over the lazy <Text style={bold}>dog</Text>.
</Text>
);
}
Style Concatenation
Can we combine two or more styles?
Recall that earlier we said that we should prefer reusing styled components over styles. That’s true, but sometimes style reuse is also useful. For instance, if we have a button style and an accentText style, we may want to combine them to create an AccentButton component.
If the styles look like this:
var styles = Stylesheet.create({
button: {
borderRadius: '8px',
backgroundColor: '#99CCFF'
},
accentText: {
fontSize: 18,
fontWeight: 'bold'
}
});
Then we can create a component that has both of those styles applied through simple concatenation. Let’s look at such an example:
var AccentButton = React.createClass({
render: function() {
return (
<Text style={[styles.button, styles.accentText]}>
{this.props.children}
</Text>
);
}
});
As we can see, the style attribute can take an array of style objects. We can also add inline styles here, like:
var AccentButton = React.createClass({
render: function() {
return (
<Text style={[styles.button, styles.accentText, {color: '#FFFFFF'}]}>
{this.props.children}
</Text>
);
}
});
In the case of a conflict, such as when two objects both specify the same property, React Native will resolve the conflict for us. The rightmost elements in the style array take precedence, and falsy values (false, null, undefined) are ignored.
Organization and Inheritance
In most of the samples yet, we append our style code to the end of the main JavaScript file with a single call to Stylesheet.create. For sample code, this works well enough, but it’s not something you’ll likely want to do in a real app. How should we actually organize styles? In this section, we will take a look at ways of organizing your styles, and how to share and inherit styles.
Exporting Style Objects
As our styles grow more complex, we will want to keep them separate from your components’ JavaScript files. One common mechanism is to have a separate folder for each component. If we have a component named <ComponentName>, we would create a folder named ComponentName/ and structure it like so:
- ComponentName
|- index.js
|- styles.js
Within styles.js, you create a stylesheet, and export it:
'use strict';
var React = require('react-native');
var {
StyleSheet,
} = React;
var styles = Stylesheet.create({
text: {
color: '#FF00FF',
fontSize: 16
},
bold: {
fontWeight: 'bold'
}
});
module.exports = styles;
Within index.js, we can import our styles like so:
var styles = require('./styles.js');
Now we can use them in our component. Here is how:
var React = require('react-native');
var styles = require('./styles.js');
var {
View,
Text,
StyleSheet
} = React;
var ComponentName = React.createClass({
render: function() {
return (
<Text style={[styles.text, styles.bold]}>
Hello, world
</Text>
);
}
});
Passing Styles as Props
We can also pass styles as properties. The propType View.propTypes.style ensures that only valid styles are passed as props.
We can use this pattern to create extensible components, which can be more effectively controlled and styled by their parents. For example, a component might take in an optional style prop.
'use strict';
var React = require('react-native');
var {
View,
Text
} = React;
var CustomizableText = React.createClass({
propTypes: {
style: Text.propTypes.Style
},
getDefaultProps: function() {
return {
style: {}
};
},
render: function() {
return (
<Text style={[myStyles.text, this.props.style]}>
Hello, world
</Text>
);
}
});
By adding this.props.style to the end of the styles array, we ensure that you can override the default props.
Reusing and Sharing Styles
We typically prefer to reuse styled components, rather than reusing styles, but there are clearly some instances in which you will want to share styles between components. In this case, a common pattern is to organize your project roughly like so:
- js
|- components
|- Button
|- index.js
|- styles.js
|- styles
|- styles.js
|- colors.js
|- fonts.js
By having separate directories for components and for styles, you can keep the intended use of each file clear based on context. A component’s folder should contain its React class, as well as any component-specific files. Shared styles should be kept out of component folders. Shared styles may include things such as your palette, fonts, standardized margins and padding, and so on.
styles/styles.js requires the other shared styles files, and exposes them; then your components can require styles.js and use shared files as needed. Or, you may prefer to have components require specific stylesheets from the styles/ directory instead.
Because we’ve now moved our styles into JavaScript, organizing your styles is really a question of general code organization; there’s no single correct approach here.
Recent Stories
Top DiscoverSDK Experts
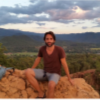
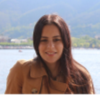
Compare Products
Select up to three two products to compare by clicking on the compare icon () of each product.
{{compareToolModel.Error}}
{{CommentsModel.TotalCount}} Comments
Your Comment